JavaScript is a wealthy language, with generally a seemingly overwhelming variety of libraries and frameworks. With so many choices accessible, it is generally helpful to only take a look at the language itself and remember its core parts. This glossary covers the core JavaScript language, syntax, and capabilities.
JavaScript variables
var
: The most used variable. Can be reassigned however solely accessed inside a operate, that means operate scope. Variables outlined with var
transfer to the highest when code is executed.
const
: Cannot be reassigned and never accessible earlier than they seem throughout the code, that means block scope.
let
: Similar to const
with block scope, nevertheless, the let
variable will be reassigned however not re-declared.
Data sorts
Numbers: var age = 33
Variables: var a
Text (strings): var a = "Sachin"
Operations: var b = 4 + 5 + 6
True or false statements: var a = true
Constant numbers: const PI = 3.14
Objects: var fullName = {firstName:"Sachin", lastName: "Samal"}
Objects
This is a straightforward instance of objects in JavaScript. This object describe the variable automotive
, and contains keys or properties reminiscent of make
, mannequin
, and yr
are the article’s property names. Each property has a price, reminiscent of Nissan
, Altima
, and 2022
. A JavaScript object is a group of properties with values, and it capabilities as a technique.
var automotive = {
make:"Nissan",
mannequin:"Altima",
yr:2022,
};
Comparison operators
==
: Is equal to
===
: Is equal worth and equal sort
!=
: Is not equal
!==
: Is not equal worth or not equal sort
>
: Is higher than
<
: Is lower than
>=
: Is higher than or equal to
<=
: Is lower than or equal to
?
: Ternary operator
Logical operators
&&
: Logical AND
||
: Logical OR
!
: Logical NOT
Output knowledge
alert()
: Output knowledge in an alert field within the browser window
verify()
: Open up a sure/no dialog and return true/false relying on person click on
console.log()
: Write data to the browser console. Good for debugging.
doc.write()
: Write on to the HTML doc
immediate()
: Create a dialog for person enter
Array strategies
Array: An object that may maintain a number of values directly.
concat()
: Join a number of arrays into one
indexOf()
: Return the primitive worth of the desired object
be part of()
: Combine parts of an array right into a single string and return the string
lastIndexOf()
: Give the final place at which a given factor seems in an array
pop()
: Remove the final factor of an array
push()
: Add a brand new factor on the finish
reverse()
: Sort parts in descending order
shift()
: Remove the primary factor of an array
slice()
: Pull a replica of a portion of an array into a brand new array
splice()
: Add positions and parts in a specified manner
toString()
: Convert parts to strings
unshift()
: Add a brand new factor to the start
valueOf()
: Return the primary place at which a given factor seems in an array
JavaScript loops
Loops: Perform particular duties repeatedly beneath utilized situations.
for (earlier than loop; situation for loop; execute after loop) {
// what to do throughout the loop
}
for
: Creates a conditional loop
whereas
: Sets up situations beneath which a loop executes not less than as soon as, so long as the desired situation is evaluated as true
do whereas
: Similar to the whereas
loop, it executes not less than as soon as and performs a test on the finish to see if the situation is met. If it’s, then it executes once more
break
: Stop and exit the cycle at sure situations
proceed
: Skip components of the cycle if sure situations are met
if-else statements
An if
assertion executes the code inside brackets so long as the situation in parentheses is true. Failing that, an elective else
assertion is executed as a substitute.
if (situation) {
// do that if situation is met
} else {
// do that if situation just isn't met
}
Strings
String strategies
charAt()
: Return a personality at a specified place inside a string
charCodeAt()
: Give the Unicode of the character at that place
concat()
: Concatenate (be part of) two or extra strings into one
fromCharCode()
: Return a string created from the desired sequence of UTF-16 code items
indexOf()
: Provide the place of the primary incidence of a specified textual content inside a string
lastIndexOf()
: Same as indexOf()
however with the final incidence, looking out backwards
match()
: Retrieve the matches of a string in opposition to a search sample
change()
: Find and change specified textual content in a string
search()
: Execute a seek for an identical textual content and return its place
slice()
: Extract a bit of a string and return it as a brand new string
break up()
: Split a string object into an array of strings at a specified place
substr()
: Extract a substring relied on a specified variety of characters, just like slice()
substring()
: Can’t settle for damaging indices, additionally just like slice()
toLowerCase()
: Convert strings to decrease case
toUpperCase()
: Convert strings to higher case
valueOf()
: Return the primitive worth (that has no properties or strategies) of a string object
Number strategies
toExponential()
: Return a string with a rounded quantity written as exponential notation
toFixed()
: Return the string of a quantity with a specified variety of decimals
toPrecision()
: String of a quantity written with a specified size
toString()
: Return a quantity as a string
valueOf()
: Return a quantity as a quantity
Math strategies
abs(a)
: Return absolutely the (optimistic) worth of a
acos(x)
: Arccosine of x
, in radians
asin(x)
: Arcsine of x
, in radians
atan(x)
: Arctangent of x
as a numeric worth
atan2(y,x)
: Arctangent of the quotient of its arguments
ceil(a)
: Value of a rounded as much as its nearest integer
cos(a)
: Cosine of a
(x
is in radians)
exp(a)
: Value of Ex
flooring(a)
: Value of a rounded right down to its nearest integer
log(a)
: Natural logarithm (base E) of a
max(a,b,c…,z)
: Return the quantity with the best worth
min(a,b,c…,z)
: Return the quantity with the bottom worth
pow(a,b)
: a
to the ability of b
random()
: Return a random quantity between 0 and 1
spherical(a)
: Value of a
rounded to its nearest integer
sin(a)
: Sine of a
(a
is in radians)
sqrt(a)
: Square root of a
tan(a)
: Tangent of an angle
Dealing with dates in JavaScript
Set dates
Date()
: Create a brand new date object with the present date and time
Date(2022, 6, 22, 4, 22, 11, 0)
: Create a customized date object. The numbers characterize yr, month, day, hour, minutes, seconds, milliseconds. You can omit something apart from yr and month.
Date("2022-07-29")
: Date declaration as a string
Pull date and time values
getDate()
: Day of the month as a quantity (1-31)
getDay()
: Weekday as a quantity (0-6)
getFullYear()
: Year as a four-digit quantity (yyyy)
getHours()
: Hour (0-23)
getMilliseconds()
: Millisecond (0-999)
getMinutes()
: Minute (0-59)
getMonth()
: Month as a quantity (0-11)
getSeconds()
: Second (0-59)
getTime()
: Milliseconds since January 1, 1970
getUTCDate()
: Day (date) of the month within the specified date in keeping with common time (additionally accessible for day, month, full yr, hours, minutes, and many others.)
parse
: Parse a string illustration of a date and return the variety of milliseconds since January 1, 1970
Set a part of a date
setDate()
: Set the day as a quantity (1-31)
setFullYear()
: Set the yr (optionally month and day)
setHours()
: Set the hour (0-23)
setMilliseconds()
: Set milliseconds (0-999)
setMinutes()
: Set the minutes (0-59)
setMonth()
: Set the month (0-11)
setSeconds()
: Set the seconds (0-59)
setTime()
: Set the time (milliseconds since January 1, 1970)
setUTCDate()
: Set the day of the month for a specified date in keeping with common time (additionally accessible for day, month, full yr, hours, minutes, and many others.)
Dom mode
Node strategies
appendChild()
: Add a brand new little one node to a component because the final little one node
cloneNode()
: Clone an HTML factor
compareDocumentPosition()
: Compare the doc place of two parts
getFeature()
: Return an object which implements the APIs of a specified characteristic
hasAttributes()
: Return true if a component has any attributes, in any other case false
hasChildNodes()
: Return true if a component has any little one nodes, in any other case false
insertBefore()
: Insert a brand new little one node earlier than a specified, current little one node
isDefaultNamespace()
: Return true if a specified namespaceURI
is the default, in any other case false
isEqualNode()
: Check if two parts are equal
isSameNode()
: Check if two parts are the identical node
isSupported()
: Return true if a specified characteristic is supported on the factor
lookupNamespaceURI()
: Return the namespaceURI
related to a given node
normalize()
: Join adjoining textual content nodes and removes empty textual content nodes in a component
removeChild()
: Remove a baby node from a component
replaceChild()
: Replace a baby node in a component
Element strategies
getAttribute()
: Return the desired attribute worth of a component node
getAttributeNS()
: Return string worth of the attribute with the desired namespace and title
getAttributeNode()
: Get the desired attribute node
getAttributeNodeNS()
: Return the attribute node for the attribute with the given namespace and title
getElementsByTagName()
: Provide a group of all little one parts with the desired tag title
getElementsByTagNameNS()
: Return a dwell HTMLCollection of parts with a sure tag title belonging to the given namespace
hasAttribute()
: Return true if a component has any attributes, in any other case false
hasAttributeNS()
: Provide a real/false worth indicating whether or not the present factor in a given namespace has the desired attribute
removeAttribute()
: Remove a specified attribute from a component
lookupPrefix()
: Return a DOMString
containing the prefix for a given namespaceURI
, if current
removeAttributeNS()
: Remove the desired attribute from a component inside a sure namespace
removeAttributeNode()
: Take away a specified attribute node and return the eliminated node
setAttribute()
: Set or change the desired attribute to a specified worth
setAttributeNS()
: Add a brand new attribute or modifications the worth of an attribute with the given namespace and title
setAttributeNode()
: Set or change the desired attribute node
setAttributeNodeNS()
: Add a brand new namespaced attribute node to a component
JavaScript occasions
Mouse
onclick
: User clicks on a component
oncontextmenu
: User right-clicks on a component to open a context menu
ondblclick
: User double-clicks on a component
onmousedown
: User presses a mouse button over a component
onmouseenter
: Pointer strikes onto a component
onmouseleave
: Pointer strikes out of a component
onmousemove
: Pointer strikes whereas it’s over a component
onmouseover
: Pointer strikes onto a component or one in every of its youngsters
setInterval()
: Call a operate or evaluates an expression at
oninput
: User enter on a component
onmouseup
: User releases a mouse button whereas over a component
onmouseout
: User strikes the mouse pointer out of a component or one in every of its youngsters
onerror
: Happens when an error happens whereas loading an exterior file
onloadeddata
: Media knowledge is loaded
onloadedmetadata
: Metadata (like dimensions and period) is loaded
onloadstart
: Browser begins in search of specified media
onpause
: Media is paused both by the person or routinely
onplay
: Media is began or is not paused
onplaying
: Media is taking part in after having been paused or stopped for buffering
onprogress
: Browser is within the technique of downloading the media
onratechange
: Media play pace modifications
onseeked
: User finishes transferring/skipping to a brand new place within the media
onseeking
: User begins transferring/skipping
onstalled
: Browser tries to load the media, however it’s not accessible
onsuspend
— Browser is deliberately not loading media
ontimeupdate
: Play place has modified (e.g., due to quick ahead)
onvolumechange
: Media quantity has modified (together with mute)
onwaiting
: Media paused however anticipated to renew (for instance, buffering)
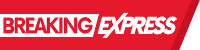