Source code have to be compiled with a purpose to run, and in open supply software program everybody has entry to supply code. Whether you have written code your self and also you wish to compile and run it, or whether or not you have downloaded any person’s venture to strive it out, it is helpful to know methods to course of supply code by way of a compiler, and in addition what precisely a compiler does with all that code.
Build a greater mousetrap
We do not often consider a mousetrap as a pc, however imagine it or not, it does share some similarities with the CPU working the machine you are studying this text on. The traditional (non-cat) mousetrap has two states: it is both set or launched. You would possibly think about that on (the kill bar is about and shops potential vitality) and off (the kill bar has been triggered.) In a way, a mousetrap is a pc that calculates the presence of a mouse. You may think this code, in an imaginary language, describing the method:
if mousetrap == 0 then
There's a mouse!
else
There's no mouse but.
finish
In different phrases, you possibly can derive mouse information primarily based on the state of a mousetrap. The mousetrap is not foolproof, after all. There may very well be a mouse subsequent to the mousetrap, and the mousetrap would nonetheless be registered as on as a result of the mouse has not but triggered the entice. So this system might use a number of enhancements, however that is fairly typical.
Switches
A mousetrap is in the end a swap. You in all probability use a swap to activate the lights in your home. Plenty of info is saved in these mechanisms. For occasion, individuals typically assume that you simply’re at dwelling when the lights are on.
You might program actions primarily based on the exercise of lights on in your neighborhood. If all lights are out, then flip down your loud music as a result of individuals have in all probability gone to mattress.
A CPU makes use of the identical logic, multiplied by a number of orders of measure, and shrunken to a microscopic degree. When a CPU receives {an electrical} sign at a selected register, then another register could be tripped, after which one other, and so forth. If these registers are made to be significant, then there’s communication taking place. Maybe a chip someplace on the identical motherboard turns into energetic, or an LED lights up, or a pixel on a display adjustments shade.
[ Related read 6 Python interpreters to try in 2022 ]
What comes round goes round. If you actually wish to detect a rodent in additional locations than the one spot you occur to have a mousetrap set, you may program an utility to do exactly that. With a webcam and a few rudimentary picture recognition software program, you may set up a baseline of what an empty kitchen seems like after which scan for adjustments. When a mouse enters the kitchen, there is a shift within the pixel values the place there was beforehand no mouse. Log the information, or higher but set off a drone that focuses in on the mouse, captures it, and strikes it outdoors. You’ve constructed a greater mousetrap by way of the magic of on and off indicators.
Compilers
A code compiler interprets human-readable code right into a machine language that speaks on to the CPU. It’s a posh course of as a result of CPUs are legitimately advanced (much more advanced than a mousetrap), but in addition as a result of the method is extra versatile than it strictly “needs” to be. Not all compilers are versatile. There are some compilers which have precisely one goal, and so they solely settle for code information in a selected structure, and so the method is comparatively straight-forward.
Luckily, fashionable general-purpose compilers aren’t easy. They let you write code in quite a lot of languages, and so they allow you to hyperlink libraries in numerous methods, and so they can goal a number of totally different architectures. The GNU C Compiler (GCC) has over 50 traces of choices in its --help
output, and the LLVM clang
compiler has over 1000 traces in its --help
output. The GCC guide comprises over 100,000 phrases.
You have plenty of choices if you compile code.
Of course, most individuals need not know all of the potential choices. There are sections within the GCC man web page I’ve by no means learn, as a result of they’re for Objective-C or Fortran or chip architectures I’ve by no means even heard of. But I worth the power to compile code for a number of totally different architectures, for 64-bit and 32-bit, and to run open supply software program on computer systems the remainder of the trade has left behind.
The compilation lifecycle
Just as importantly, there’s actual energy to understanding the totally different phases of compiling code. Here’s the lifecycle of a easy C program:
-
C supply with macros (
.c
) is preprocessed withcpp
to render an.i
file. -
C supply code with expanded macros (
.i
) is translated withgcc
to render an.s
file. -
A textual content file in Assembly language (
.s
) is assembled withas
into an.o
file. -
Binary object code with directions for the CPU, and with offsets not tied to reminiscence areas relative to different object information and libraries (
*.o
) is linked withld
to supply an executable. -
The last binary file both has all required objects inside it, or it is set to load linked dynamic libraries (
*.so
information).
And this is a easy demonstration you possibly can strive (with some adjustment for library paths):
$ cat << EOF >> hey.c
#embrace
int important(void)
{ printf("hello worldn");
return 0; }
EOF
$ cpp hey.c > hey.i
$ gcc -S hey.i
$ as -o hey.o hey.s
$ ld -static -o hey
-L/usr/lib64/gcc/x86_64-slackware-linux/5.5.0/
/usr/lib64/crt1.o /usr/lib64/crti.o hey.o
/usr/lib64/crtn.o --start-group -lc -lgcc
-lgcc_eh --end-group
$ ./hey
hey world
Attainable information
Computers have change into amazingly highly effective, and pleasantly user-friendly. Don’t let that idiot you into believing both of the 2 potential extremes: computer systems aren’t so simple as mousetraps and light-weight switches, however in addition they aren’t past comprehension. You can find out about compiling code, about methods to hyperlink, and compile for a special structure. Once that, you possibly can debug your code higher. You can perceive the code you obtain. You might even repair a bug or two. Or, in concept, you may construct a greater mousetrap. Or a CPU out of mousetraps. It’s as much as you.
Download our new eBook: An open source developer’s guide to building applications
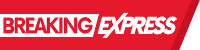