Lua is not an object-oriented programming language, however a scripting language using C features and a C-like syntax. However, there is a cool hack you should use inside Lua code to make Lua act like an object-oriented language while you want it to be. The key’s within the Lua desk assemble, and this text demonstrates learn how to use a Lua desk as a stand-in for an object-oriented class.
What is object-oriented programming?
The time period “object-oriented” is a elaborate manner of describing, primarily, a templating system. Imagine you are programming an software to assist customers spot and log zombies throughout a zombie apocalypse. You’re utilizing an object-oriented language like C++, Java, or Python. You must create code objects that characterize several types of zombies so the consumer can drag them round and prepare them on a map of town. Of course a zombie may be any variety of issues: dormant, gradual, quick, hungry, ravenous, and so forth. That’s simply textual knowledge, which computer systems are good at monitoring, and primarily based on that knowledge you can even assign the digital “object” a graphic so your consumer can determine which basic sort of zombie every widget represents.
You have a couple of choices for how one can resolve this requirement on your software:
-
Force your customers to learn to code to allow them to program their very own zombies into your software
-
Spend the remainder of your life programming each attainable sort of zombie into your software
-
Use a code template to outline the attributes of a zombie object, permitting your customers to create simply the objects they want, primarily based on what zombie they’ve truly noticed
Obviously, the one sensible choice is the ultimate one, and it is performed with a programming assemble referred to as a class. Here’s what a category would possibly seem like (this instance occurs to be Java code, however the idea is similar throughout all object-oriented languages):
Whether or not you perceive the code, you’ll be able to most likely inform that that is primarily a template. It’s declaring that when a digital “object” is created to characterize a zombie, the programming language assigns that object 4 attributes (two integers representing peak and weight, and two phrases representing the motion pace and bodily location). When the consumer clicks the (imaginary) Add merchandise button within the (imaginary) software, this class is used as a template (in programming, they are saying “a new instance” of the category has been created) to assign values entered by the consumer. Infinite zombies for the value of only one class. That’s one of many powers of object-oriented programming.
Lua tables
In Lua, the desk is a knowledge sort that implements an associative array. You can consider a desk in Lua as a database. It’s a retailer of listed data that you would be able to recall through the use of a particular syntax. Here’s a quite simple instance:
instance = {}
instance.greeting = "hello"
instance.area = " "
instance.topic = "world"
print(instance.greeting ..
instance.area ..
instance.topic)
Run the instance code to see the outcomes:
$ lua ./instance.lua
whats up world
As you’ll be able to inform from the pattern code, a desk is basically a bunch of keys and values saved inside a single assortment (a “table”).
A metatable is a desk that serves as a template for a desk. You can designate any desk as a metatable, after which deal with it a lot as you’ll a category in another language.
Here’s a metatable to outline a zombie:
Zombie = {}
operate Zombie.init(h,w,s,l)
native self = setmetatable({}, Zombie)
self.peak = h
self.weight = w
self.pace = s
self.location = l
return self
finish
-- use the metatable
operate setup()
z1 = Zombie.init(176,50,'gradual','Forbes & Murray Avenue')
finish
operate run()
print(z1.location .. ": " ..
z1.peak .. " cm, " .. z1.weight .. " kg, " .. z1.pace)
finish
setup()
run()
To differentiate my metatable from a traditional desk, I capitalize the primary letter of its title. That’s not required, however I discover it a helpful conference.
Here’s the outcomes of the pattern code:
$ lua ./zombie.lua
Forbes & Murray Avenue: 176 cm, 50 kg, gradual
This demonstration would not completely do metatables justice, as a result of the pattern code creates only a single object with no interplay from the consumer. To use a metatable to fulfill the problem of making infinite objects from only one metatable, you’ll as an alternative code a consumer enter operate (utilizing Lua’s io.learn()
operate) asking the consumer to offer the small print of the zombie they noticed. You’d most likely even code a consumer interface with a “New sighting” button, or one thing like that. That’s past the scope of this text, although, and would solely complicate the instance code.
The vital factor to recollect is that to create a metatable, you employ this syntax:
Example = {}
operate Example.init(args)
native self = setmetatable({}, Example)
self.key = worth
return self
finish
To use a metatable as soon as it has been created, use this syntax:
my_instance = Example.init("required args")
Object-oriented Lua
Lua is not object-oriented, however its desk mechanism makes dealing with and monitoring and sorting knowledge so simple as it presumably may be. It’s no exaggeration to say that when you are comfy with tables in Lua, you may be assured that you recognize not less than half of the language. You can use tables (and, by extension, metatables) as arrays, maps, handy variable group, close-enough courses, and extra.
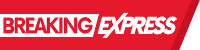