I train college programs part-time, together with a category about basic computing subjects, open to all majors. This is an introductory course that teaches college students about how know-how works, to take away the thriller round computing.
While not a pc science course, one part of this course covers pc programming. I normally speak about programming in very summary phrases, so I do not lose my viewers. But this yr, I needed my college students to do some “hands-on” programming in an “old school” manner. At the identical time, I needed to maintain it easy, so everybody might comply with alongside.
I wish to construction my classes to point out how you bought from “there” to “here.” Ideally, I’d let my college students learn to write a easy program. Then I’d choose it up from there to point out how trendy programming permits builders to create extra advanced packages. I made a decision to attempt an unconventional strategy — train the scholars concerning the final in low-level programming: machine language.
Machine language programming
Early private computer systems just like the Apple II (1977), TRS-80 (1977), and IBM PC (1981) let customers enter packages with a keyboard, and displayed outcomes on a display screen. But computer systems did not at all times include a display screen and keyboard.
The Altair 8800 and IMSAI 8080 (each made in 1975) required customers to enter a program utilizing “switches and lights” on a panel. You would enter an instruction in machine language, utilizing a financial institution of switches, and the machine would gentle up those and zeros of every binary instruction utilizing LEDs.
(Cromemco, CC BY-NC-SA 3.0)
Programming these early machines required understanding the machine language directions, known as opcodes, quick for operation codes, to carry out fundamental operations like including two numbers or storing a price into the pc’s reminiscence. I needed to point out my college students how programmers would enter a sequence of directions and reminiscence addresses by hand, utilizing the switches and lights.
However, utilizing an precise Altair 8800 can be an excessive amount of overhead on this class. I wanted one thing easy that any beginner-level pupil might grasp. Ideally, I hoped to discover a easy “hobby” retro pc that labored equally to the Altair 8800, however I could not discover a appropriate “Altair-like” system for lower than $100. I discovered a number of “Altair” software program emulators, however they faithfully reproduce the Altair 8800 opcodes, and that was an excessive amount of for my wants.
I made a decision to put in writing my very own “educational” retro pc. I name it the Toy CPU. You can discover it on my GitHub repository, together with a number of releases to play with. Version 1 was an experimental prototype that ran on FreeDOS. Version 2 was an up to date prototype that ran on Linux with ncurses. Version 3 is a FreeDOS program that runs in graphics mode.
Programming the Toy CPU
The Toy CPU is a quite simple retro pc. Sporting solely 256 bytes of reminiscence and a minimal instruction set, the Toy CPU goals for simplicity whereas replicating the “switches and lights” programming mannequin. The interface mimics the Altair 8800, with a sequence of eight LEDs for the counter (the “line number” for this system), instruction, accumulator (inside reminiscence used for momentary knowledge), and standing.
When you begin the Toy CPU, it simulates “booting” by clearing the reminiscence. While the Toy CPU is beginning up, it additionally shows INI
(“initialize”) within the standing lights on the bottom-right of the display screen. The PWR
(“power”) gentle signifies the Toy CPU has been turned on.
(Jim Hall, CC BY-SA 4.0)
When the Toy CPU is prepared so that you can enter a program, it signifies INP
(“input” mode) through the standing lights, and begins you at counter 0 in this system. Programs for the Toy CPU at all times begin at counter 0.
In “input” mode, use the up and down arrow keys to point out the totally different program counters, and press Enter to edit the instruction on the present counter. When you enter “edit” mode, the Toy CPU exhibits EDT
(“edit” mode) on the standing lights.
(Jim Hall, CC BY-SA 4.0)
The Toy CPU has a cheat sheet that is “taped” to the entrance of the show. This lists the totally different opcodes the Toy CPU can course of:
-
00000000
(STOP
): Stop program execution. -
00000001
(RIGHT
): Shift the bits within the accumulator to the precise by one place. The worth 00000010 turns into 00000001, and 00000001 turns into 00000000. -
00000010
(LEFT
): Shift the bits within the accumulator to the left by one place. The worth 01000000 turns into 10000000, and 10000000 turns into 00000000. -
00001111
(NOT
): Binary NOT the accumulator. For instance, the worth 10001000 turns into 01110111. -
00010001
(AND
): Binary AND the accumulator with the worth saved at an handle. The handle is saved within the subsequent counter. -
00010010
(OR
): Binary OR the accumulator with the worth saved at an handle. -
00010011
(XOR
): Binary XOR (“exclusive or”) the accumulator with the worth saved at an handle. -
00010100
(LOAD
): Load (copy) the worth from an handle into the accumulator. -
00010101
(STORE
): Store (copy) the worth within the accumulator into an handle. -
00010110
(ADD
): Add the worth saved at an handle to the accumulator. -
00010111
(SUB
): Subtract the worth saved at an handle from the accumulator. -
00011000
(GOTO
): Go to (bounce to) a counter handle. -
00011001
(IFZERO
): If the accumulator is zero, go to (bounce to) a counter handle. -
10000000
(NOP
): No operation; safely ignored.
When in “edit” mode, use the left and proper arrow keys to pick out a bit within the opcode, and press Space
to flip the worth between off (0) and on (1). When you might be achieved modifying, press Enter
to return to “input” mode.
(Jim Hall, CC BY-SA 4.0)
A pattern program
I need to discover the Toy CPU by getting into a brief program that provides two values, and shops the end result within the Toy’s reminiscence. Effectively, this performs the arithmetic operation A+B=C. To create this program, you solely want a couple of opcodes:
-
00010100
(LOAD
): Load (copy) the worth from an handle into the accumulator. -
00010110
(ADD
): Add the worth saved at an handle to the accumulator. -
00010101
(STORE
): Store (copy) the worth within the accumulator into an handle. -
00000000
(STOP
): Stop program execution.
The LOAD
, ADD
, and STORE
directions require a reminiscence handle, which is able to at all times be within the subsequent counter location. For instance, the primary two directions of this system are:
counter 0: 00010100
counter 1: some reminiscence handle the place the primary worth A is saved
The instruction in counter 0 is the LOAD
operation, and the worth in counter 1 is the reminiscence handle the place you could have saved some worth. The two directions collectively copy a price from reminiscence into the Toy’s accumulator, the place you’ll be able to work on the worth.
Having loaded a quantity A into the accumulator, it’s good to add the worth B to it. You can try this with these two directions:
counter 2: 00010110
counter 3: a reminiscence handle the place the second worth B is saved
Say that you just loaded the worth 1 (A) into the accumulator, then added the worth 3 (B) to it. The accumulator will now have the worth 4. Now it’s good to copy the worth 4 into one other reminiscence handle (C) with these two directions:
counter 4: 00010101
counter 5: a reminiscence handle (C) the place we will save the brand new worth
Having added the 2 values collectively, now you can finish this system with this instruction:
counter 6: 00000000
Any directions after counter 6 can be found for this system to make use of as saved reminiscence. That means you need to use the reminiscence at counter 7 for the worth A, the reminiscence in counter 8 for the worth B, and the reminiscence at counter 9 for the saved worth C. You must enter these individually into the Toy:
counter 7: 00000001 (1)
counter 8: 00000011 (3)
counter 9: 00000000 (0, will probably be overwritten later)
Having found out all of the directions and the reminiscence areas for A, B, and C, now you can enter the total program into the Toy. This program provides the values 1 and three to get 4:
counter 0: 00010100
counter 1: 00000111 (7)
counter 2: 00010110
counter 3: 00001000 (8)
counter 4: 00010101
counter 5: 00001001 (9)
counter 6: 00000000
counter 7: 00000001 (1)
counter 8: 00000011 (3)
counter 9: 00000000 (0, will probably be overwritten later)
To run this system, press the R
key when in “input” mode. The Toy CPU will present RUN
(“run” mode) within the standing lights, and execute your program beginning at counter 0.
The Toy has a big delay constructed into it, so you’ll be able to watch the Toy execute every step in this system. You ought to see the counter transfer from 00000000 (0) to 00000110 (6) as this system progresses. After counter 1, this system masses the worth 1 from reminiscence location 7, and the accumulator updates to 00000001 (1). After counter 3, this system will add the worth 3 and replace the accumulator to point out 00000100 (4). The accumulator will stay that manner till this system shops the worth into reminiscence location 9 after counter 5 then ends at counter 6.
(Jim Hall, CC BY-SA 4.0)
Exploring machine language programming
You can use the Toy to create different packages and additional discover machine language programming. Test your creativity by writing these packages in machine language.
A program to flash the lights on the accumulator
Can you gentle up the precise 4 bits on the accumulator, then the left 4 bits, then the entire bits? You can write this program in considered one of two methods:
An easy strategy can be to load three values from totally different reminiscence addresses, like this:
counter 0: LOAD
counter 1: "right"
counter 2: LOAD
counter 3: "left"
counter 4: LOAD
counter 5: "all"
counter 6: STOP
counter 7: 00001111 ("right")
counter 8: 11110000 ("left")
counter 9: 11111111 ("all")
Another strategy to write this program is to experiment with the NOT and OR binary operations. This ends in a smaller program:
counter 0: LOAD
counter 1: "right"
counter 2: NOT
counter 3: OR
counter 4: "right"
counter 5: STOP
counter 6: 00001111 ("right")
Count down from a quantity
You can use the Toy as a countdown timer. This program workout routines the IFZERO check, which is able to bounce this system to a brand new counter provided that the accumulator is zero:
counter 0: LOAD
counter 1: "initial value"
counter 2: IFZERO (that is additionally the "start" of the countdown)
counter 3: "end"
counter 4: SUB
counter 5: "one"
counter 6: GOTO
counter 7: "start"
counter 8: STOP
counter 9: 00000111 ("initial value")
counter 10: 00000001 ("one")
The Toy CPU is a good way to study machine language. I used the Toy CPU in my introductory course, and the scholars stated they discovered it troublesome to put in writing the primary program, however writing the following one was a lot simpler. The college students additionally commented that writing packages on this manner was truly enjoyable, they usually realized rather a lot about how computer systems truly work. The Toy CPU is academic and enjoyable!
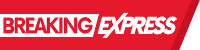