DocArray is a library for nested, unstructured, multimodal knowledge in transit, together with textual content, picture, audio, video, 3D mesh, and so forth. It permits deep-learning engineers to effectively course of, embed, search, retailer, suggest, and switch multi-modal knowledge with a Pythonic API. Starting in November of 2022, DocArray is open supply and hosted by the Linux Foundation AI & Data initiative in order that there’s a impartial residence for constructing and supporting an open AI and knowledge group. This is the beginning of a brand new day for DocArray.
In the ten months since DocArray’s first launch, its builders at Jina AI have seen an increasing number of adoption and contributions from the open supply group. Today, DocArray powers a whole bunch of multimodal AI purposes.
Hosting an open supply mission with the Linux Foundation
Hosting a mission with the Linux Foundation follows open governance, which means there’s nobody firm or particular person accountable for a mission. When maintainers of an open supply mission determine to host it on the Linux Foundation, they particularly switch the mission’s trademark possession to the Linux Foundation.
In this text, I’ll assessment the historical past and way forward for DocArray. In specific, I’ll display some cool options which are already in improvement.
A quick historical past of DocArray
Jina AI launched the idea of “DocArray” in Jina 0.8 in late 2020. It was the jina.sorts
module, meant to finish neural search design patterns by clarifying low-level knowledge illustration in Jina. Rather than working with Protobuf immediately, the brand new Document class provided a less complicated and safer high-level API to characterize multimodal knowledge.
(Jina AI, CC BY-SA 4.0)
Over time, we prolonged jina.sorts
and moved past a easy Pythonic interface of Protobuf. We added DocumentArray to ease batch operations on a number of DocumentArrays. Then we introduced in IO and pre-processing features for various knowledge modalities, like textual content, picture, video, audio, and 3D meshes. The Executor class began to make use of DocumentArray for enter and output. In Jina 2.0 (launched in mid-2021) the design turned stronger nonetheless. Document, Executor, and Flow turned Jina’s three elementary ideas:
• Document is the info IO in Jina
• Executor defines the logic of processing Documents
• Flow ties Executors collectively to perform a activity.
The group cherished the brand new design, because it significantly improved the developer expertise by hiding pointless complexity. This lets builders deal with the issues that basically matter.
(Jina AI, CC BY-SA 4.0)
As jina.sorts
grew, it turned conceptually unbiased from Jina. While jina.sorts
was extra about constructing domestically, the remainder of Jina targeted on service-ization. Trying to attain two very totally different targets in a single codebase created upkeep hurdles. On the one hand, jina.sorts
needed to evolve quick and maintain including options to fulfill the wants of the quickly evolving AI group. On the opposite hand, Jina itself needed to stay secure and strong because it served as infrastructure. The outcome? A slowdown in improvement.
We tackled this by decoupling jina.sorts
from Jina in late 2021. This refactoring served as the inspiration of the later DocArray. It was then that DocArray’s mission crystallized for the crew: to offer a knowledge construction for AI engineers to simply characterize, retailer, transmit, and embed multimodal knowledge. DocArray focuses on native developer expertise, optimized for quick prototyping. Jina scales issues up and uplifts prototypes into companies in manufacturing. With that in thoughts, Jina AI launched DocArray 0.1 in parallel with Jina 3.0 in early 2022, independently as a brand new open supply mission.
We selected the title DocArray as a result of we need to make one thing as elementary and widely-used as NumPy’s ndarray
. Today, DocArray is the entrypoint of many multimodal AI purposes, like the favored DALLE-Flow and DiscoArt. DocArray builders launched new and highly effective options, equivalent to dataclass and doc retailer to enhance usability much more. DocArray has allied with open supply companions like Weaviate, Qdrant, Redis, FastAPI, pydantic, and Jupyter for integration and most significantly for in search of a standard normal.
In the DocArray 0.19 (launched on Nov. 15), you may simply characterize and course of 3D mesh knowledge.
(Jina AI, CC BY-SA 4.0)
The way forward for DocArray
Donating DocArray to the Linux Foundation marks an vital milestone the place we share our dedication with the open supply group brazenly, inclusively, and constructively.
The subsequent launch of DocArray focuses on 4 duties:
• Representing: help Python idioms for representing sophisticated, nested multimodal knowledge with ease.
• Embedding: present easy interfaces for mainstream deep studying fashions to embed knowledge effectively.
• Storing: help a number of vector databases for environment friendly persistence and approximate nearest neighbor retrieval.
• Transiting: permit quick (de)serialization and turn out to be a typical wire protocol on gRPC, HTTP, and WebSockets.
In the next sections, DocArray maintainers Sami Jaghouar and Johannes Messner provide you with a style of the subsequent launch.
All-in-dataclass
In DocArray, dataclass is a high-level API for representing a multimodal doc. It follows the design and idiom of the usual Python dataclass, letting customers characterize sophisticated multimodal paperwork intuitively and course of them simply with DocArray’s API. The new launch makes dataclass a first-class citizen and refactors its previous implementation through the use of pydantic V2.
How to make use of dataclass
Here’s easy methods to use the brand new dataclass. First, you need to know {that a} Document
is a pydantic mannequin with a random ID and the Protobuf interface:
From docarray import Document
To create your personal multimodal knowledge kind you simply must subclass from Document
:
from docarray import Document
from docarray.typing import Tensor
import numpy as np
class Banner(Document):
alt_text: str
picture: Tensor
banner = Banner(textual content='DocArray is wonderful', picture=np.zeros((3, 224, 224)))
Once you’ve got outlined a Banner
, you should use it as a constructing block to characterize extra sophisticated knowledge:
class BlogPost(Document):
title: str
excerpt: str
banner: Banner
tags: List[str]
content material: str
Adding an embedding discipline to BlogPost
is straightforward. You can use the predefined Document
fashions Text
and Image
, which include the embedding discipline baked in:
from typing import Optional
from docarray.typing import Embedding
class Image(Document):
src: str
embedding: Optional[Embedding]
class Text(Document):
content material: str
embedding: Optional[Embedding]
Then you may characterize your BlogPost
:
class Banner(Document):
alt_text: str
picture: Image
class BlogPost(Document):
title: Text
excerpt: Text
banner: Banner
tags: List[str]
content material: Text
This provides your multimodal BlogPost
4 embedding representations: title
, excerpt
, content material
, and banner
.
Milvus help
Milvus is an open-source vector database and an open-source mission hosted underneath Linux Foundation AI & Data. It’s extremely versatile, dependable, and blazing quick, and helps including, deleting, updating, and close to real-time search of vectors on a trillion-byte scale. As step one in direction of a extra inclusive DocArray, developer Johannes Messner has been implementing Milvus integration.
As with different doc shops, you may simply instantiate a DocumentArray
with Milvus storage:
from docarray import DocumentArray
da = DocumentArray(storage='milvus', config={'n_dim': 10})
Here, config
is the configuration for the brand new Milvus assortment, and n_dim
is a compulsory discipline that specifies the dimensionality of saved embeddings. The code under exhibits a minimal working instance with a working Milvus server on localhost:
import numpy as np
from docarray import DocumentArray
N, D = 5, 128
da = DocumentArray.empty(
N, storage='milvus', config={'n_dim': D, 'distance': 'IP'}
) # init
with da:
da.embeddings = np.random.random([N, D])
print(da.discover(np.random.random(D), restrict=10))
To entry endured knowledge from one other server, you want to specify collection_name
, host
, and port
. This permits customers to take pleasure in all the advantages that Milvus affords, via the acquainted and unified API of DocArray.
Embracing open governance
The time period “open governance” refers back to the approach a mission is ruled — that’s, how selections are made, how the mission is structured, and who’s chargeable for what. In the context of open supply software program, “open governance” means the mission is ruled brazenly and transparently, and anybody is welcome to take part in that governance.
Open governance for DocArray has many advantages:
• DocArray is now democratically run, making certain that everybody has a say.
• DocArray is now extra accessible and inclusive, as a result of anybody can take part in governance.
• DocArray might be of upper high quality, as a result of selections are being made in a clear and open approach.
The improvement crew is taking actions to embrace open governance, together with:
• Creating a DocArray technical steering committee (TSC) to assist information the mission.
• Opening up the event course of to extra enter and suggestions from the group.
• Making DocArray improvement extra inclusive and welcoming to new contributors.
Join the mission
If you are excited about open supply AI, Python, or large knowledge, then you definitely’re invited to observe together with the DocArray mission because it develops. If you suppose you’ve got one thing to contribute to it, then be a part of the mission. It’s a rising group, and one which’s open to everybody.
This article was initially revealed on the Jina AI blog and has been republished with permission.
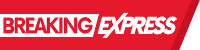