Writing the identical utility in a number of languages is a good way to be taught new methods to program. Most programming languages have sure issues in frequent, equivalent to:
- Variables
- Expressions
- Statements
These ideas are the idea of most programming languages. Once you perceive them, you possibly can take the time it’s good to determine the remainder.
Furthermore, programming languages normally share some similarities. Once you already know one programming language, you possibly can be taught the fundamentals of one other by recognizing its variations.
An excellent device for studying a brand new language is by practising with a normal program.
This permits you to give attention to the language, not this system’s logic. I’m doing that on this article collection utilizing a “guess the number” program, through which the pc picks a quantity between 1 and 100 and asks you to guess it. The program loops till you guess the quantity accurately.
This program workouts a number of ideas in programming languages:
- Variables
- Input
- Output
- Conditional analysis
- Loops
It’s an amazing sensible experiment to be taught a brand new programming language.
Guess the quantity in Zig primary
Zig remains to be within the alpha stage, and topic to alter. This article is appropriate as of zig model 0.11. Install zig
by going to the downloads listing and downloading the suitable model to your working system and structure:
const std = @import("std");
fn ask_user() !i64 {
const stdin = std.io.getStdIn().reader();
const stdout = std.io.getStdOut().author();
var buf: [10]u8 = undefined;
attempt stdout.print("Guess a number between 1 and 100: ", .{});
if (attempt stdin.readUntilDelimiterOrEof(buf[0..], 'n')) |user_input| {
return std.fmt.parseInt(i64, user_input, 10);
} else {
return error.InvalidParam;
}
}
pub fn fundamental() !void {
const stdout = std.io.getStdOut().author();
var prng = std.rand.DefaultPrng.init(blk: {
var seed: u64 = undefined;
attempt std.os.getrandom(std.mem.asBytes(&seed));
break :blk seed;
});
const worth = prng.random().intRangeAtMost(i64, 1, 100);
whereas (true) {
const guess = attempt ask_user();
if (guess == worth) {
break;
}
const message =
if (guess < worth)
"low"
else
"high";
attempt stdout.print("Too {s}n", .{message});
}
attempt stdout.print("That's rightn", .{});
}
The first line const std = @import("std");
imports the Zig standard library.
Almost all applications will want it.
The ask_user perform in Zig
The perform ask_user()
returns a 64-bit integer or an error. This is what the !
(exclamation mark) notes. This means if there’s an I/O situation or the consumer enters an invalid enter, the perform returns an error.
The attempt
operator calls a perform and return its worth. If it returns an error, it instantly returns from the calling perform with an error. This permits specific, however straightforward, error propagation. The first two strains in ask_user
alias incorporates some constants from std
.
This makes the next I/O code easier.
This line prints the immediate:
attempt stdout.print("Guess a number between 1 and 100: ", .{});
It routinely returns a failure if the print fails (for instance, writing to a closed terminal).
This line defines the buffer into which consumer enter is learn:
var buf: [10]u8 = undefined;
The expression contained in the if clause reads consumer enter into the buffer:
(attempt stdin.readUntilDelimiterOrEof(buf[0..], 'n')) |user_input|
The expression returns the slice of the buffer that was learn into. This is assigned to the variable user_input
, which is simply legitimate contained in the if
block.
The perform std.fmt.parseInt
returns an error if the quantity can’t be parsed.
This error is propagated to the caller. If no bytes have been learn, the perform instantly returns an error.
The fundamental perform
The perform begins by getting a random quantity. It makes use of std.rand.DefaultPrng
.
The perform initializes the random quantity generator with std.os.getrandom
. It then makes use of the generator to get a quantity within the vary of 1
to 100
.
The whereas
loop continues whereas true
is true, which is perpetually. The solely manner out is with the break
, which occurs when the guess is the same as the random worth.
When the guess
will not be equal, the if
assertion returns the string low
or excessive
relying on the guess. This is interpolated into the message to the consumer.
Note that fundamental
is outlined as !void
, which suggests it could additionally return an error. This permits utilizing the attempt
operator inside fundamental
.
Sample output
An instance run, after placing this system in fundamental.zig
:
$ zig run fundamental.zig
Guess a quantity between 1 and 100: 50
Too excessive
Guess a quantity between 1 and 100: 25
Too low
Guess a quantity between 1 and 100: 37
Too low
Guess a quantity between 1 and 100: 42
Too excessive
Guess a quantity between 1 and 100: 40
That's proper
Summary
This “guess the number” recreation is a superb introductory program for studying a brand new programming language as a result of it workouts a number of frequent programming ideas in a fairly easy manner. By implementing this easy recreation in numerous programming languages, you possibly can reveal some core ideas of the languages and evaluate their particulars.
Do you’ve gotten a favourite programming language? How would you write the “guess the number” recreation in it? Follow this text collection to see examples of different programming languages which may curiosity you!
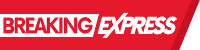