My path to Tcl began with a current must automate a troublesome Java-based command-line configuration utility. I do a little bit of automation programming utilizing Ansible, and I often use the count on module. Frankly, I discover this module has restricted utility for a variety of causes together with: problem with sequencing an identical prompts, capturing values to be used in further steps, restricted flexibility with management logic, and so forth. Sometimes you will get away with utilizing the shell module as an alternative. But generally you hit that ill-behaving and overly difficult command-line interface that appears inconceivable to automate.
In my case, I used to be automating the set up of one in all my firm’s packages. The final configuration step might solely be carried out via the command-line, via a number of ill-formed, repeating prompts and knowledge output that wanted capturing. The good outdated conventional Expect was the one reply. A deep understanding of Tcl isn’t vital to make use of the fundamentals of Expect, however the extra you understand, the extra energy you will get from it. This is a subject for a follow-up article. For now, I discover the fundamental language constructs of Tcl, which embody person enter, output, variables, conditional analysis, looping, and easy capabilities.
Install Tcl
On a Linux system, I take advantage of this:
# dnf set up tcl
# which tclsh
/bin/tclsh
On macOS, you should utilize Homebrew to put in the most recent Tcl:
$ brew set up tcl-tk
$ which tclsh
/usr/native/bin/tclsh
Guess the quantity in Tcl
Start by creating the fundamental executable script numgame.tcl
:
$ contact numgame.tcl
$ chmod 755 numgame.tcl
And then begin coding in your file headed up by the same old shebang script header:
#!/usr/bin/tclsh
Here are a couple of fast phrases about artifacts of Tcl to trace together with this text.
The first level is that every one of Tcl is taken into account a sequence of strings. Variables are usually handled as strings however can change varieties and inside representations robotically (one thing you usually don’t have any visibility into). Functions might interpret their string arguments as numbers ( expr
) and are solely handed in by worth. Strings are normally delineated utilizing double quotes or curly braces. Double quotes enable for variable enlargement and escape sequences, and curly braces impose no enlargement in any respect.
The subsequent level is that Tcl statements might be separated by semicolons however normally should not. Statement traces might be cut up utilizing the backslash character. However, it is typical to surround multiline statements inside curly braces to keep away from needing this. Curly braces are simply less complicated, and the code formatting beneath displays this. Curly braces enable for deferred analysis of strings. A worth is handed to a operate earlier than Tcl does variable substitution.
Finally, Tcl makes use of sq. brackets for command substitution. Anything between the sq. brackets is distributed to a brand new recursive invocation of the Tcl interpreter for analysis. This is useful for calling capabilities in the midst of expressions or for producing parameters for capabilities.
Procedures
Although not vital for this sport, I begin with an instance of defining a operate in Tcl that you should utilize later:
proc used_time {begin} {
return [expr [clock seconds] - $begin]
}
Using proc
units this as much as be a operate (or process) definition. Next comes the identify of the operate. This is then adopted by a listing containing the parameters; on this case 1 parameter {begin}
after which adopted by the operate physique. Note that the physique curly brace begins on this line, it can’t be on the next line. The operate returns a worth. The returned worth is a compound analysis (sq. braces) that begins by studying the system clock [clock seconds]
and does the maths to subtract out the $begin
parameter.
Setup, logic, and end
You can add extra particulars to the remainder of this sport with some preliminary setup, iterating over the participant’s guesses, after which printing outcomes when accomplished:
set num [expr round(rand()*100)]
set starttime [clock seconds]
set guess -1
set rely 0
places "Guess a number between 1 and 100"
whereas { $guess != $num } {
incr rely
places -nonewline "==> "
flush stdout
will get stdin guess
if { $guess < $num } {
places "Too small, try again"
} elseif { $guess > $num } {
places "Too large, try again"
} else {
places "That's right!"
}
}
set used [used_time $starttime]
places "You guessed value $num after $count tries and $used elapsed seconds"
The first set
statements set up variables. The first two consider expressions to discern a random quantity between 1 and 100, and the following one saves the system clock begin time.
The places
and will get
command are used for output to and enter from the participant. The places
I’ve used suggest commonplace out for output. The will get
wants the enter channel to be outlined, so this code specifies stdin
because the supply for terminal enter from the person.
The flush stdout
command is required when places
omits the end-of-line termination as a result of Tcl buffers output and it won’t get displayed earlier than the following I/O is required.
From there the whereas
assertion illustrates the looping management construction and conditional logic wanted to provide the participant suggestions and finally finish the loop.
The last set
command calls our operate to calculate elapsed seconds for gameplay, adopted by the collected stats to finish the sport.
Play it!
$ ./numgame.tcl
Guess a quantity between 1 and 100
==> 100
Too massive, attempt once more
==> 50
Too massive, attempt once more
==> 25
Too massive, attempt once more
==> 12
Too massive, attempt once more
==> 6
Too massive, attempt once more
==> 3
That's proper!
You guessed worth 3 after 6 tries and 20 elapsed seconds
Continue studying
When I began this train, I doubted simply how helpful going again to a late Nineties fad language can be to me. Along the best way, I discovered a couple of issues about Tcl that I actually loved — my favourite being the sq. bracket command analysis. It simply appears a lot simpler to learn and use than many different languages that overuse difficult closure constructions. What I assumed was a dead language was truly nonetheless thriving and supported on a number of platforms. I discovered a couple of new abilities and grew an appreciation for this venerable language.
Check out the official web site over at https://www.tcl-lang.org. You can discover references to the most recent supply, binary distributions, boards, docs, and knowledge on conferences which are nonetheless ongoing.
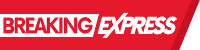