Control constructions are an vital function of programming languages as a result of they permit you to direct the circulate of this system based mostly on circumstances which are usually established dynamically as this system is operating. Different languages present totally different controls, and in Lua there’s the whereas loop, for loop, and repeat till loop. This article covers the whereas and repeat till loops. Because of their flexibility, I cowl for loops in a separate article.
A situation is outlined by an expression utilizing an operator, which is a flowery time period for symbols you might acknowledge from math lessons. Valid operators in Lua are:
Those are often known as relational operators as a result of they immediate an investigation of how two values relate to at least one one other. There are additionally logical operators, which imply the identical as they imply in English and will be integrated into circumstances to additional describe the state you wish to test for:
Here are some instance circumstances:
-
foo > 3
: Is the variablefoo
is bigger than 3? Thefoo
should be 4 or extra to fulfill this situation. -
foo >= 3
: Isfoo
better than or equal to three? Thefoo
should be 3 or extra to fulfill this situation. -
foo > 3 and bar < 1
: Isfoo
better than 3 whereasbar
is lower than 1? For this situation to be true, thefoo
variable should be 4 or extra on the identical second thatbar
is 0. -
foo > 3 or bar < 1
: Isfoo
better than 3? Alternately, isbar
lower than 1? Iffoo
is 4 or extra, orbar
is 0, then this situation is true. What occurs iffoo
is 4 or extra whereasbar
is 0? The reply seems later on this article.
While loop
Some time loop executes directions for so long as some situation is happy. For instance, suppose you are growing an utility to observe an ongoing zombie apocalypse. When there aren’t any zombies remaining, then there isn’t any extra zombie apocalypse:
zombie = 1024
whereas (zombie > 0) do
print(zombie)
zombie = zombie-1
finish
if zombie == 0 then
print("No more zombie apocalypse!")
finish
Run the code to look at the zombies vanish:
$ lua ./whereas.lua
1024
1023
[...]
3
2
1
No extra zombie apocalypse!
Until loop
Lua additionally has a repeat till loop assemble that is basically some time loop with a “catch” assertion. Suppose you have taken up gardening and also you wish to observe what’s left to reap:
mytable = { "tomato", "lettuce", "brains" }
bc = 3
repeat
print(mytable[bc])
bc = bc - 1
till( bc == 0 )
Run the code:
$ lua ./till.lua
brains
lettuce
tomato
That’s useful!
Infinite loops
An infinite loop has a situation that may by no means be happy, so it runs infinitely. This is usually a bug attributable to dangerous logic or an surprising state in your program. For occasion, initially of this text, I posed a logic puzzle. If a loop is about to run till foo > 3 or bar < 1
, then what occurs when foo
is 4 or extra whereas bar
is 0?
Here’s the code to resolve this puzzle, with a security catch utilizing the break
assertion simply in case:
foo = 9
bar = 0
whereas ( foo > 3 or bar < 1 ) do
print(foo)
foo = foo-1
-- security catch
if foo < -800000 then
break
finish
finish
You can safely run this code, nevertheless it does mimic an unintentional infinite loop. The flawed logic is the or
operator, which allows this loop to proceed each when foo
is bigger than 3 and when bar
is lower than 1. The and
operator has a special impact, however I depart that to you to discover.
Infinite loops truly do have their makes use of. Graphical functions use what are technically infinite loops to maintain the appliance window open. There’s no approach of figuring out how lengthy your consumer intends to make use of the appliance, so this system runs infinitely till the consumer selects Quit. The easy situation utilized in these instances is one that’s clearly all the time happy. Here’s an instance infinite loop, once more with a security catch in-built for comfort:
n = 0
whereas true do
print(n)
n = n+1
if n > 100 then
break
finish
finish
The situation whereas true
is all the time happy as a result of true
is all the time true. It’s the terse approach of writing whereas 1 == 1
or one thing equally eternally true.
Loops in Lua
As you’ll be able to inform from the pattern code, though there are totally different implementations, loops all mainly work towards the identical purpose. Choose the one which is smart to you and that works finest with the processing you might want to carry out. And simply in case you want it: The keyboard shortcut to terminate a runaway loop is Ctrl+C.
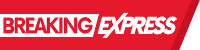