April 1st is all about faux tales and pretending. This makes it the proper day to speak about mocking.
Sometimes, utilizing actual objects is difficult, ill-advised, or difficult. For instance, a requests.Session
connects to actual web sites. Using it in your unittests invitations a…lot…of issues.
Basic mocking in Python
“Mocks” are a unittest idea. They produce objects which are substitutes for the actual ones.
from unittest import mock
There’s an entire cottage business that may clarify that “mock”, “fake”, and “stub” are all subtly totally different. In this text, I exploit the phrases interchangeably.
common = mock.MagicMock()
def do_something(o):
return o.one thing(5)
do_something(common)
This code produces:
<MagicMock title="mock.something()" id='140228824621520'>
Mocks have all of the strategies. The strategies normally return one other Mock. This may be modified by assigning it to return_value
.
For instance, suppose you wish to name the next operate:
def do_something(o):
return o.one thing() + 1
It requires one thing which has the .one thing()
technique. Luckily, mock objects have it:
obj = mock.MagicMock(title="an object")
obj.one thing.return_value = 2
print(do_something(obj))
The reply:
3
It can be doable to override the “magic methods”:
a = mock.MagicMock()
a.__str__.return_value = "an a"
print(str(a))
The reply:
an a
The spec
Make positive {that a} mock doesn’t have “extra” strategies or attributes by utilizing a spec. For instance, here is some code that ought to fail:
import pathlib
def bad_pathlib_usage(path):
## TYPO: lacking underscore
path.writetext("hello")
dummy_path = mock.MagicMock(spec=pathlib.Path)
attempt:
bad_pathlib_usage(dummy_path)
besides Exception as exc:
print("Failed!", repr(exc))
The outcome:
Failed! AttributeError("Mock object has no attribute 'writetext'")
Mock aspect impact
Sometimes, having a MagicMock
that returns the identical factor each time is not fairly the whole lot you want it to be. For instance, sys.stdin.readline()
normally returns totally different values, not the identical worth all through the check.
The property side_effect
permits controlling what a magic mock returns on a extra detailed degree than utilizing return_value
.
Iterable
One of the issues that may be assigned to side_effect
is an iterable, equivalent to a sequence or a generator.
This is a strong function. It permits controlling every name’s return worth, with little code.
different_things = mock.MagicMock()
different_things.side_effect = [1, 2, 3]
print(different_things())
print(different_things())
print(different_things())
The output:
1
2
3
A extra reasonable instance is when simulating file enter. In this case, I need to have the ability to management what readline
returns every time to fake it is file enter:
def parse_three_lines(fpin):
line = fpin.readline()
title, worth = line.cut up()
modifier = fpin.readline().strip()
additional = fpin.readline().strip()
return {title: f"{value}/{modifier}+{extra}"}
from io import TextIOBase
filelike = mock.MagicMock(spec=TextIOBase)
filelike.readline.side_effect = [
"thing importantn",
"a-littlen",
"to-some-peoplen"
]
worth = parse_three_lines(filelike)
print(worth)
The outcome:
{'factor': 'essential/a-little+to-some-people'}
Exception
Another factor that is doable is assigning an exception to the side_effect
attribute. This causes the decision to boost the exception you assigned. Using this function permits simulating edge circumstances within the setting, normally exactly those that:
- You care about
- Are onerous to simulate realistically
One standard case is community points. As per Murphy’s legislation, they all the time occur at 4 AM, inflicting a pager to go off, and by no means at 10 AM while you’re sitting at your desk. The following is predicated on actual code I wrote to check a community service.
In this simplified instance, the code returns the size of the response line, or a unfavorable quantity if a timeout has been reached. The quantity is totally different based mostly on when within the protocol negotiation this has been reached. This permits the code to differentiate “connection timeout” from “response timeout”, for instance.
Testing this code in opposition to an actual server is difficult. Servers attempt onerous to keep away from outages! You may fork the server’s C code and add some chaos or you’ll be able to simply use side_effect
and mock:
import socket
def careful_reader(sock):
sock.settimeout(5)
attempt:
sock.join(("some.host", 8451))
besides socket.timeout:
return -1
attempt:
sock.sendall(b"DO THINGn")
besides socket.timeout:
return -2
fpin = sock.makefile()
attempt:
line = fpin.readline()
besides socket.timeout:
return -3
return len(line.strip())
from io import TextIOBase
from unittest import mock
sock = mock.MagicMock(spec=socket.socket)
sock.join.side_effect = socket.timeout("too long")
print(careful_reader(sock))
The result’s a failure, which on this case means a profitable check:
-1
With cautious uncomfortable side effects, you will get to every of the return values. For instance:
sock = mock.MagicMock(spec=socket.socket)
sock.sendall.side_effect = socket.timeout("too long")
print(careful_reader(sock))
The outcome:
-2
Callable
The earlier instance is simplified. Real community service check code should confirm that the outcomes it bought have been appropriate to validate that the server works accurately. This means doing an artificial request and in search of an accurate outcome. The mock object has to emulate that. It has to carry out some computation on the inputs.
Trying to check such code with out performing any computation is tough. The exams are typically too insensitive or too “flakey”.
- An insensitive check is one that doesn’t fail within the presence of bugs.
- A flakey check is one which typically fails, even when the code is appropriate.
Here, my code is inaccurate. The insensitive check doesn’t catch it, whereas the flakey check would fail even when it was mounted!
import socket
import random
def yolo_reader(sock):
sock.settimeout(5)
sock.join(("some.host", 8451))
fpin = sock.makefile()
order = [0, 1]
random.shuffle(order)
whereas order:
if order.pop() == 0:
sock.sendall(b"GET KEYn")
key = fpin.readline().strip()
else:
sock.sendall(b"GET VALUEn")
worth = fpin.readline().strip()
return {worth: key} ## Woops bug, needs to be {key: worth}
The following can be too “insensitive”, not detecting the bug:
sock = mock.MagicMock(spec=socket.socket)
sock.makefile.return_value.readline.return_value = "interestingn"
assert yolo_reader(sock) == {"interesting": "interesting"}
The following can be too “flakey,” detecting the bug even when it is not there, typically:
for i in vary(10):
sock = mock.MagicMock(spec=socket.socket)
sock.makefile.return_value.readline.side_effect = ["keyn", "valuen"]
if yolo_reader(sock) != {"key": "value"}:
print(i, finish=" ")
For instance:
3 6 7 9
The remaining possibility of getting outcomes from a mock object is to assign a callable object to side_effect
. This calls side_effect
to easily name it. Why not simply assign a callable object on to the attribute? Have persistence, I’ll get to that within the subsequent half!
In this instance, my callable object (only a operate) assigns a return_value
to the attribute of one other object. This is not that unusual. I’m simulating the setting, and in an actual setting, poking one factor usually impacts different issues.
sock = mock.MagicMock(spec=socket.socket)
def sendall(knowledge):
cmd, title = knowledge.decode("ascii").cut up()
if title == "KEY":
sock.makefile.return_value.readline.return_value = "keyn"
elif title == "VALUE":
sock.makefile.return_value.readline.return_value = "valuen"
else:
increase ValueError("got bad command", title)
sock.sendall.side_effect = sendall
print(yolo_reader(sock), dict(key="value"))
The outcome:
{'worth': 'key'} {'key': 'worth'}
Mock name arguments: x-ray for code
When writing a unit check, you’re “away” from the code however making an attempt to look into its guts to see the way it behaves. The Mock object is your sneaky spy. After it will get into the manufacturing code, it data the whole lot faithfully. This is how yow will discover what your code does and whether or not it is the precise factor.
Call counts
The easiest factor is to simply guarantee that the code known as the anticipated variety of instances. The .call_count
attribute is precisely what counts that.
def get_values(names, shopper):
ret_value = []
cache = {}
for title in names:
# title = title.decrease()
if title not in cache:
worth = shopper.get(f"https://httpbin.org/anything/grab?name={name}").json()['args']['name']
cache[name] = worth
ret_value.append(cache[name])
return ret_value
shopper = mock.MagicMock()
shopper.get.return_value.json.return_value = dict(args=dict(title="something"))
outcome = get_values(['one', 'One'], shopper)
print(outcome)
print("call count", shopper.get.call_count)
The outcomes:
['something', 'something']
name rely 2
One good thing about checking .call_count >= 1
versus checking .known as
is that it’s extra resistant to crazy typos.
def call_function(func):
print("I'm going to call the function, really!")
if False:
func()
print("I just called the function")
func = mock.MagicMock()
call_function(func)
print(func.callled) # TYPO -- Extra "l"
I'm going to name the operate, actually!
I simply known as the operate
<MagicMock title="mock.callled" id='140228249343504'>
Using spec
diligently can forestall that. However, spec
shouldn’t be recursive. Even if the unique mock object has a spec, uncommon is the check that makes positive that each single attribute it has additionally has a spec. However, utilizing .call_count
as an alternative of .known as
is an easy hack that utterly eliminates the prospect to make this error.
Call arguments
In the following instance, I make sure the code calls the strategy with the right arguments. When automating knowledge heart manipulations, it is essential to get issues proper. As they are saying, “To err is human, but to destroy an entire data center requires a robot with a bug.”
We wish to be sure that our Paramiko-based automation accurately will get the sizes of information, even when the file names have areas in them.
def get_remote_file_size(shopper, fname):
shopper.join('ssh.instance.com')
stdin, stdout, stderr = shopper.exec_command(f"ls -l {fname}")
stdin.shut()
outcomes = stdout.learn()
errors = stderr.learn()
stdout.shut()
stderr.shut()
if errors != '':
increase ValueError("problem with command", errors)
return int(outcomes.cut up()[4])
fname = "a file"
shopper = mock.MagicMock()
shopper.exec_command.return_value = [mock.MagicMock(name=str(i)) for i in range(3)]
shopper.exec_command.return_value[1].learn.return_value = f"""
-rw-rw-r-- 1 consumer consumer 123 Jul 18 20:25 {fname}
"""
shopper.exec_command.return_value[2].learn.return_value = ""
outcome = get_remote_file_size(shopper, fname)
assert outcome == 123
[args], kwargs = shopper.exec_command.call_args
import shlex
print(shlex.cut up(args))
The outcomes:
['ls', '-l', 'a', 'file']
Woops! That’s not the precise command. Good factor you checked the arguments.
Deep dive into mocks
Mocks have a number of energy. Like any highly effective instrument, utilizing it improperly is a quick option to get into a giant mess. But correctly utilizing the .return_value
, .side_effect
, and the varied .name*
properties, it is doable to write down the most effective kind of unit exams.
A very good unit check is one which:
- Fails within the presence of incorrect code
- Passes within the presence of appropriate code
“Quality” shouldn’t be binary. It exists on a spectrum. The badness of a unit check is decided by:
- How many errors it lets go. That’s a “missing alarm” or “false negative”. If you are a statistician, it is a “type 2 error”.
- How many appropriate code modifications it fails. That’s a “false alarm” or “false positive”. If you are a statistician, it is a “type 1 errors”.
When utilizing a mock, take the time and take into consideration each metrics to judge whether or not this mock and this unit check, will assist or hinder you.
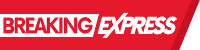