When laptop shows had a restricted coloration palette, net designers typically used a set of web-safe colors to create web sites. While fashionable web sites displaying on newer units can show many extra colours than the unique web-safe coloration palette, I typically wish to consult with the web-safe colours after I create net pages. This manner I do know my pages look good wherever.
You can discover web-safe coloration palettes on the net, however I wished to have my very own copy for simple reference. And you may make one too, utilizing the for
loop in Bash.
Bash for loop
The syntax of a for loop in Bash appears to be like like this:
for variable in set ; do statements ; finished
As an instance, say you wish to print all numbers from 1 to three. You can write a fast for
loop on the Bash command line to do this for you:
$ for n in 1 2 3 ; do echo $n ; finished
1
2
3
The semicolons are a typical Bash assertion separator. They allow you to write a number of instructions on a single line. If you had been to incorporate this for
loop in a Bash script file, you would possibly as an alternative change the semicolons with line breaks and write out the for
loop like this:
for n in 1 2 3
do
echo $n
finished
I like to incorporate the do
on the identical line because the for
so it is simpler for me to learn:
for n in 1 2 3 ; do
echo $n
finished
More than one for loop at a time
You can put one loop inside one other. That will help you to iterate over a number of variables, to do a couple of factor at a time. Let’s say you wished to print out all mixtures of the letters A, B, and C with the numbers 1, 2, and three. You can do this with two for
loops in Bash, like this:
#!/bin/bash
for quantity in 1 2 3 ; do
for letter in A B C ; do
echo $letter$quantity
finished
finished
If you place these strains in a Bash script file known as for.bash
and run it, you see 9 strains exhibiting the mixtures of all of the letters paired with every of the numbers:
$ bash for.bash
A1
B1
C1
A2
B2
C2
A3
B3
C3
Looping via the web-safe colours
The web-safe colours are all colours from hexadecimal coloration #000
(black, the place the crimson, inexperienced, and blue values are all zero) to #fff
(white, the place the crimson, inexperienced, and blue colours are all at their full intensities), stepping via every hexadecimal worth as 0, 3, 6, 9, c, and f.
You can generate an inventory of all mixtures of the web-safe colours utilizing three for
loops in Bash, the place the loops iterate over the crimson, inexperienced, and blue values.
#!/bin/bash
for r in 0 3 6 9 c f ; do
for g in 0 3 6 9 c f ; do
for b in 0 3 6 9 c f ; do
echo "#$r$g$b"
finished
finished
finished
If you save this in a brand new Bash script known as websafe.bash
and run it, you see an iteration of all the online protected colours as hexadecimal values:
$ bash websafe.bash | head
#000
#003
#006
#009
#00c
#00f
#030
#033
#036
#039
To make an HTML web page that you need to use as a reference for web-safe colours, you could make every entry a separate HTML aspect. Put every coloration in a <div>
aspect, and set the background to the web-safe coloration. To make the hexadecimal worth simpler to learn, put it inside a separate <code>
aspect. Update the Bash script to appear to be this:
#!/bin/bash
for r in 0 3 6 9 c f ; do
for g in 0 3 6 9 c f ; do
for b in 0 3 6 9 c f ; do
echo "<div style="background-color:#$r$g$b"><code>#$r$g$b</code></div>"
finished
finished
finished
When you run the brand new Bash script and save the outcomes to an HTML file, you may view the output in an online browser to all of the web-safe colours:
$ bash websafe.bash > websafe.html
(Jim Hall, CC BY-SA 4.0)
The net web page is not very good to take a look at. The black textual content on a darkish background is unimaginable to learn. I like to use some HTML styling to make sure the hexadecimal values are displayed with white textual content on a black background inside the colour rectangle. To make the web page look very nice, I additionally use HTML grid kinds to rearrange the bins with six per row and a few house between every field.
To add this further styling, you could embrace the opposite HTML parts earlier than and after the for loops. The HTML code on the high defines the kinds and the HTML code on the backside closes all of the open HTML tags:
#!/bin/bash
cat<<EOF
<!DOCTYPE html>
<html lang="en">
<head>
<title>Web-safe colours</title>
<meta identify="viewport" content material="width=device-width, initial-scale=1">
<type>
div {
padding-bottom: 1em;
}
code {
background-color: black;
coloration: white;
}
@media solely display and (min-width:600px) {
physique {
show: grid;
grid-template-columns: repeat(6,1fr);
column-gap: 1em;
row-gap: 1em;
}
div {
padding-bottom: 3em;
}
}
</type>
</head>
</physique>
EOF
for r in 0 3 6 9 c f ; do
for g in 0 3 6 9 c f ; do
for b in 0 3 6 9 c f ; do
echo "<div
type="background-color:#$r$g$b"><code>#$r$g$b</code></div>"
finished
finished
finished
cat<<EOF
</physique>
</html>
EOF
This completed Bash script generates a web-safe coloration information in HTML. Whenever you could consult with the web-safe colours, run the script and save the outcomes to an HTML web page. Now you may see a illustration of the web-safe colours in your browser as an easy-reference information on your subsequent net venture:
$ bash websafe.bash > websafe.html
(Jim Hall, CC BY-SA 4.0)
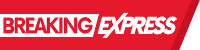