Open supply tasks that create a wrapper round an API have gotten more and more in style. These tasks make it simpler for builders to work together with APIs and use them of their purposes. The openshift-python-wrapper
challenge is a wrapper round openshift-restclient-python. What started as an inner bundle to assist our staff work with the OpenShift API turned an open source project (Apache License 2.0).
This article discusses what an API wrapper is, why it is helpful, and a few examples from the wrapper.
Why use an API wrapper?
An API wrapper is a layer of code that sits between an software and an API. It simplifies the API entry course of by abstracting away a few of the complexities concerned in making requests and parsing responses. A wrapper may present further performance past what the API itself provides, equivalent to caching or error dealing with.
Using an API wrapper makes the code extra modular and simpler to take care of. Instead of writing customized code for each API, you need to use a wrapper that gives a constant interface for interacting with APIs. It saves time, avoids code duplications, and reduces the possibility of errors.
Another good thing about utilizing an API wrapper is that it could actually defend your code from adjustments to the API. If an API adjustments its interface, you’ll be able to replace the wrapper code with out modifying your software code. This can scale back the work required to take care of your software over time.
Install
The software is on PyPi, so set up openshift-python-wrapper
utilizing the pip command:
$ python3 -m pip set up openshift-python-wrapper
Python wrapper
The OpenShift REST API offers programmatic entry to most of the options of the OpenShift platform. The wrapper provides a easy and intuitive interface for interacting with the API utilizing the openshift-restclient-python
library. It standardizes easy methods to work with cluster sources and provides unified useful resource CRUD (Create, Read, Update, and Delete) flows. It additionally offers further capabilities, equivalent to resource-specific performance that in any other case must be applied by customers. The wrapper makes code simpler to learn and preserve over time.
One instance of simplified utilization is interacting with a container. Running a command inside a container requires utilizing Kubernetes stream, dealing with errors, and extra. The wrapper handles all of it and offers simple and intuitive functionality.
>>> from ocp_resources.pod import Pod
>>> from ocp_utilities.infra import get_client
>>> consumer = get_client()
ocp_utilities.infra INFO Trying to get
consumer by way of new_client_from_config
>>> pod = Pod(consumer=consumer, title="nginx-deployment-7fb96c846b-b48mv", namespace="default")
>>> pod.execute("ls")
ocp_resources Pod INFO Execute ls on
nginx-deployment-7fb96c846b-b48mv (ip-10-0-155-108.ec2.inner)
'binnbootndevnetcnhomenlibnlib64nmedianmntnoptnprocnrootnrunnsbinnsrvnsysntmpnusrnvarn'
Developers or testers can use this wrapper—our staff wrote the code whereas conserving testing in thoughts. Using Python capabilities, context managers can present out-of-the-box useful resource creation and deletion, and inheritance can be utilized to increase performance for particular use instances. Pytest fixtures can make the most of the code for setup and teardown, leaving no leftovers. Resources may even be saved for debugging. Resource manifests and logs could be simply collected.
Here’s an instance of a context supervisor:
@pytest.fixture(scope="module")
def namespace():
admin_client = get_client()
with Namespace(consumer=admin_client, title="test-ns",) as ns:
ns.wait_for_status(standing=Namespace.Status.ACTIVE, timeout=240)
yield ns
def test_ns(namespace):
print(namespace.title)
Generators iterate over sources, as seen beneath:
>>> from ocp_resources.node import Node
>>> from ocp_utilities.infra import get_client
>>> admin_client = get_client()
# This returns a generator
>>> for node in Node.get(dyn_client=admin_client):
print(node.title)
ip-10-0-128-213.ec2.inner
Open supply code for open supply communities
To paraphrase a well-liked saying, “If you love your code, set it free.” The openshift-python-wrapper
challenge began as utility modules for OpenShift Virtualization. As an increasing number of tasks benefitted from the code, we determined to extract these utilities right into a separate repository and have it open sourced. To paraphrase one other frequent saying, “If the code does not return to you, it means it was never yours.” We like saying that when that occurs, it is actually open supply.
More contributors and maintainers imply that the code belongs to the group. Everyone is welcome to contribute.
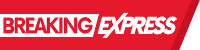