In the first article of this series, I defined easy methods to use Python to create a easy, text-based cube recreation. In the second half, I confirmed you easy methods to construct a recreation from scratch, beginning with creating the game’s environment. But each recreation wants a participant, and each participant wants a playable character, so that is what we’ll do subsequent within the third a part of the collection.
In Pygame, the icon or avatar that a participant controls is known as a sprite. If you have no graphics to make use of for a participant sprite but, create one thing for your self utilizing Krita or Inkscape. If you lack confidence in your inventive abilities, you may also search OpenClipArt.org or OpenGameArt.org for one thing pre-generated. Then, when you did not already accomplish that within the earlier article, create a listing known as pictures
alongside your Python mission listing. Put the pictures you wish to use in your recreation into the pictures
folder.
To make your recreation actually thrilling, you ought to make use of an animated sprite in your hero. It means it’s important to draw extra property, but it surely makes a giant distinction. The commonest animation is a stroll cycle, a collection of drawings that make it appear to be your sprite is strolling. The fast and soiled model of a stroll cycle requires 4 drawings.
Note: The code samples on this article enable for each a static participant sprite and an animated one.
Name your participant sprite hero.png
. If you are creating an animated sprite, append a digit after the title, beginning with hero1.png
.
Create a Python class
In Python, whenever you create an object that you just wish to seem on display, you create a category.
Near the highest of your Python script, add the code to create a participant. In the code pattern beneath, the primary three traces are already within the Python script that you just’re engaged on:
import pygame
import sys
import os # new code beneathclass Player(pygame.sprite.Sprite):
'''
Spawn a participant
'''
def __init__(self):
pygame.sprite.Sprite.__init__(self)
self.pictures = []
img = pygame.picture.load(os.path.be a part of('pictures','hero.png')).convert()
self.pictures.append(img)
self.picture = self.pictures[zero]
self.rect = self.picture.get_rect()
If you’ve a stroll cycle in your playable character, save every drawing as a person file known as hero1.png
to hero4.png
within the pictures
folder.
Use a loop to inform Python to cycle by every file.
'''
Objects
'''class Player(pygame.sprite.Sprite):
'''
Spawn a participant
'''
def __init__(self):
pygame.sprite.Sprite.__init__(self)
self.pictures = []
for i in vary(1,5):
img = pygame.picture.load(os.path.be a part of('pictures','hero' + str(i) + '.png')).convert()
self.pictures.append(img)
self.picture = self.pictures[zero]
self.rect = self.picture.get_rect()
Bring the participant into the sport world
Now that a Player class exists, you need to use it to spawn a participant sprite in your recreation world. If you by no means name on the Player class, it by no means runs, and there might be no participant. You can check this out by operating your recreation now. The recreation will run simply in addition to it did on the finish of the earlier article, with the very same outcomes: an empty recreation world.
To convey a participant sprite into your world, you need to name the Player class to generate a sprite after which add it to a Pygame sprite group. In this code pattern, the primary three traces are present code, so add the traces afterwards:
world = pygame.show.set_mode([worldx,worldy])
backdrop = pygame.picture.load(os.path.be a part of('pictures','stage.png')).convert()
backdropbox = display.get_rect()# new code beneath
participant = Player() # spawn participant
participant.rect.x = zero # go to x
participant.rect.y = zero # go to y
player_list = pygame.sprite.Group()
player_list.add(participant)
Try launching your recreation to see what occurs. Warning: it will not do what you anticipate. When you launch your mission, the participant sprite would not spawn. Actually, it spawns, however just for a millisecond. How do you repair one thing that solely occurs for a millisecond? You may recall from the earlier article that you have to add one thing to the principle loop. To make the participant spawn for longer than a millisecond, inform Python to attract it as soon as per loop.
Change the underside clause of your loop to appear to be this:
world.blit(backdrop, backdropbox)
player_list.draw(display) # draw participant
pygame.show.flip()
clock.tick(fps)
Launch your recreation now. Your participant spawns!
Setting the alpha channel
Depending on the way you created your participant sprite, it might have a coloured block round it. What you might be seeing is the area that must be occupied by an alpha channel. It’s meant to be the “color” of invisibility, however Python would not know to make it invisible but. What you might be seeing, then, is the area throughout the bounding field (or “hit box,” in fashionable gaming phrases) across the sprite.
You can inform Python what shade to make invisible by setting an alpha channel and utilizing RGB values. If you do not know the RGB values your drawing makes use of as alpha, open your drawing in Krita or Inkscape and fill the empty area round your drawing with a singular shade, like #00ff00 (kind of a “greenscreen green”). Take notice of the colour’s hex worth (#00ff00, for greenscreen inexperienced) and use that in your Python script because the alpha channel.
Using alpha requires the addition of two traces in your Sprite creation code. Some model of the primary line is already in your code. Add the opposite two traces:
img = pygame.picture.load(os.path.be a part of('pictures','hero' + str(i) + '.png')).convert()
img.convert_alpha() # optimise alpha
img.set_colorkey(ALPHA) # set alpha
Python would not know what to make use of as alpha except you inform it. In the setup space of your code, add some extra shade definitions. Add this variable definition wherever in your setup part:
In this instance code, zero,255,zero is used, which is identical worth in RGB as #00ff00 is in hex. You can get all of those shade values from a great graphics software like GIMP, Krita, or Inkscape. Alternately, you may also detect shade values with a great system-wide shade chooser, like KColorChooser.
If your graphics software is rendering your sprite’s background as another worth, alter the values of your alpha variable as wanted. No matter what you set your alpha worth, it is going to be made “invisible.” RGB values are very strict, so if you have to use 00zero for alpha, however you want 00zero for the black traces of your drawing, simply change the traces of your drawing to 111, which is shut sufficient to black that no person however a pc can inform the distinction.
Launch your recreation to see the outcomes.
In the fourth part of this series, I will present you easy methods to make your sprite transfer. How thrilling!
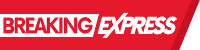