As I described in “My DeLorean runs Perl,” switching to Perl has vastly improved my growth velocity and potentialities. Here I am going to dive deeper into the design of Perl 5 to debate points necessary to programs programming.
Some years in the past, I wrote “OpenGL bindings for Bash” as type of a joke. The implementation was merely an X11 program written in C that learn OpenGL calls on stdin (sure, as textual content) and emitted person enter on stdout. Then I had slightly bash embrace
file that might declare all of the OpenGL features as Bash features, which echoed the identify of the operate right into a pipe, beginning the GL interpreter course of if it wasn’t already operating. The level of the train was to indicate that OpenGL (the 1.four API, not the newer shader stuff) may render quite a lot of graphics with only a few calls per body through the use of GL show lists. The OpenGL library did all of the heavy lifting, and Bash simply printed a number of dozen traces of textual content per body.
In the tip although, Bash is a extremely horrible glue language, each from excessive overhead and restricted obtainable operations and syntax. Perl, however, is a good glue language.
Syntax apart…
If you are not an everyday Perl person, the very first thing you in all probability discover is the syntax.
Perl 5 is constructed on a protracted legacy of awkward syntax, however more moderen variations have eliminated the necessity for a lot of the punctuation. The remaining warts can principally be averted by selecting modules that offer you domain-specific “syntactic sugar,” which even alter the Perl syntax as it’s parsed. This is in stark distinction to most different languages, the place you’re caught with the syntax you are given, and infinitely extra versatile than C’s macros. Combined with Perl’s highly effective sparse-syntax operators, like map
, grep
, kind
, and comparable user-defined operators, I can nearly at all times write advanced algorithms extra legibly and with much less typing utilizing Perl than with JavaScript, PHP, or any compiled language.
So, as a result of syntax is what you make of it, I feel the underlying machine is crucial side of the language to think about. Perl 5 has a really succesful engine, and it differs in fascinating and helpful methods from different languages.
A layer above C
I do not suggest anybody begin working with Perl by wanting on the interpreter’s inner API, however a fast description is beneficial. One of the principle issues we cope with on this planet of C is buying and releasing reminiscence whereas additionally supporting management circulate by means of a sequence of operate calls. C has a tough skill to throw exceptions utilizing longjmp
, but it surely would not do any cleanup for you, so it’s nearly ineffective with out a framework to handle assets. The Perl interpreter is strictly this type of framework.
Perl offers a stack of variables unbiased from C’s stack of operate calls on which you’ll mark the logical boundaries of a Perl scope. There are additionally API calls you need to use to allocate reminiscence, Perl variables, and so on., and inform Perl to robotically free them on the finish of the Perl scope. Now you can also make no matter C calls you want, “die” out of the center of them, and let Perl clear all the things up for you.
Although it is a actually unconventional perspective, I convey it as much as emphasize that Perl sits on high of C and means that you can use as a lot or as little interpreted overhead as you want. Perl’s inner API is definitely not as good as C++ for normal programming, however C++ would not offer you an interpreted language on high of your work if you’re accomplished. I’ve misplaced monitor of the variety of occasions that I wished reflective functionality to examine or alter my C++ objects, and following that rabbit gap has derailed a couple of of my private tasks.
Lisp-like features
Perl features take an inventory of arguments. The draw back is that you need to do argument depend and kind checking at runtime. The upside is you do not find yourself doing that a lot, as a result of you possibly can simply let the interpreter’s personal runtime test catch these errors. You also can create the impact of C++’s overloaded features by inspecting the arguments you got and behaving accordingly.
Because arguments are an inventory, and return values are an inventory, this encourages Lisp-style programming, the place you utilize a collection of features to filter an inventory of knowledge components. This “piping” or “streaming” impact may end up in some actually difficult loops turning right into a single line of code.
Every operate is out there to the language as a coderef
that may be handed round in variables, together with nameless closure features. Also, I discover sub
extra handy to sort than JavaScript’s operate()
or C++11’s [&]()
.
Generic information buildings
The variables in Perl are both “scalars,” references, arrays, or “hashes” … or another stuff that I am going to skip.
Scalars act as a string/integer/float hybrid and are robotically typecast as wanted for the aim you’re utilizing them. In different phrases, as an alternative of figuring out the operation by the kind of variable, the kind of operator determines how the variable must be interpreted. This is much less environment friendly than if the language is aware of the kind upfront, however not as inefficient as, for instance, shell scripting as a result of Perl caches the kind conversions.
Perl scalars could comprise null characters, so they’re totally usable as buffers for binary information. The scalars are mutable and copied by worth, however optimized with copy-on-write, and substring operations are additionally optimized. Strings assist unicode characters however are saved effectively as regular bytes till you append a codepoint above 255.
References (that are thought of scalars as properly) maintain a reference to every other variable; hashrefs
and arrayrefs
are most typical, together with the coderefs
described above.
Arrays are merely a dynamic-length array of scalars (or references).
Hashes (i.e., dictionaries, maps, or no matter you wish to name them) are a performance-tuned hash desk implementation the place each key’s a string and each worth is a scalar (or reference). Hashes are utilized in Perl in the identical method structs are utilized in C. Clearly a hash is much less environment friendly than a struct, but it surely retains issues generic so duties that require dozens of traces of code in different languages can grow to be one-liners in Perl. For occasion, you possibly can dump the contents of a hash into an inventory of (key, worth) pairs or reconstruct a hash from such an inventory as a pure a part of the Perl syntax.
Object mannequin
Any reference might be “blessed” to make it into an object, granting it a multiple-inheritance method-dispatch desk. The blessing is just the identify of a bundle (namespace), and any operate in that namespace turns into an obtainable methodology of the thing. The inheritance tree is outlined by variables within the bundle. As a end result, you can also make modifications to lessons or class hierarchies or create new lessons on the fly with easy information edits, quite than particular key phrases or built-in reflection APIs. By combining this with Perl’s native
key phrase (the place modifications to a world are robotically undone on the finish of the present scope), you possibly can even make short-term modifications to class strategies or inheritance!
Perl objects solely have strategies, so attributes are accessed by way of accessors just like the canonical Java get_
and set_
strategies. Perl authors normally mix them right into a single methodology of simply the attribute identify and differentiate get
from set
by whether or not a parameter was given.
You also can “re-bless” objects from one class to a different, which allows fascinating tips not obtainable in most different languages. Consider state machines, the place every methodology would usually begin by checking the thing’s present state; you possibly can keep away from that in Perl by swapping the strategy desk to 1 that matches the thing’s state.
Visibility
While different languages spend a bunch of effort on entry guidelines between lessons, Perl adopted a easy “if the name begins with underscore, don’t touch it unless it’s yours” conference. Although I can see how this could possibly be an issue with an undisciplined software program group, it has labored nice in my expertise. The solely factor C++’s personal
key phrase ever did for me was impair my debugging efforts, but it felt soiled to make all the things public
. Perl removes my guilt.
Likewise, an object offers strategies, however you possibly can ignore them and simply entry the underlying Perl information construction. This is one other enormous increase for debugging.
Garbage assortment by way of reference counting
Although reference counting is a quite leak-prone type of reminiscence administration (it would not detect cycles), it has a number of upsides. It provides you deterministic destruction of your objects, like in C++, and by no means interrupts your program with a shock rubbish assortment. It strongly encourages module authors to make use of a tree-of-objects sample, which I a lot desire vs. the tangle-of-objects sample typically seen in Java and JavaScript. (I’ve discovered bushes to be far more simply examined with unit assessments.) But, if you happen to want a tangle of objects, Perl does provide “weak” references, which will not be thought of when deciding if it is time to garbage-collect one thing.
On the entire, the one time this ever bites me is when making heavy use of closures for event-driven callbacks. It’s straightforward to have an object maintain a reference to an occasion deal with holding a reference to a callback that references the containing object. Again, weak references clear up this, but it surely’s an additional factor to pay attention to that JavaScript or Python do not make you are concerned about.
Parallelism
The Perl interpreter is a single thread, though modules written in C can use threads of their very own internally, and Perl typically contains assist for a number of interpreters inside the identical course of.
Although it is a massive limitation, figuring out that a information construction will solely ever be touched by one thread is sweet, and it means you do not want locks when accessing them from C code. Even in Java, the place locking is constructed into the syntax in handy methods, it may be an actual time sink to cause by means of all of the ways in which threads can work together (and particularly annoying that they power you to cope with that in each GUI program you write).
There are a number of occasion libraries obtainable to help in writing event-driven callback applications within the model of Node.js to keep away from the necessity for threads.
Access to C libraries
Aside from immediately writing your individual C extensions by way of Perl’s XS system, there are already numerous frequent C libraries wrapped for you and obtainable on Perl’s CPAN repository. There can be a fantastic module, Inline::C, that takes many of the ache out of bridging between Perl and C, to the purpose the place you simply paste C code into the center of a Perl module. (It compiles the primary time you run it and caches the .so shared object file for subsequent runs.) You nonetheless have to be taught a few of the Perl interpreter API if you wish to manipulate the Perl stack or pack/unpack Perl’s variables aside from your C operate arguments and return worth.
Memory utilization
Perl can use a shocking quantity of reminiscence, particularly if you happen to make use of heavyweight libraries and create 1000’s of objects, however with the scale of as we speak’s programs it normally would not matter. It additionally is not a lot worse than different interpreted programs. My private choice is to solely use light-weight libraries, which additionally typically enhance efficiency.
Startup velocity
The Perl interpreter begins in beneath 5 milliseconds on trendy hardware. If you are taking care to make use of solely light-weight modules, you need to use Perl for something you might need used Bash for, like hotplug
scripts.
Regex implementation
Perl offers the mom of all regex implementations… however you in all probability already knew that. Regular expressions are constructed into Perl’s syntax quite than being an object-oriented or function-based API; this helps encourage their use for any textual content processing you may have to do.
Ubiquity and stability
Perl 5 is put in on nearly each trendy Unix system, and the CPAN module assortment is in depth and straightforward to put in. There’s a production-quality module for nearly any job, with strong check protection and good documentation.
Perl 5 has practically full backward compatibility throughout twenty years of releases. The group has embraced this as properly, so most of CPAN is fairly secure. There’s even a crew of testers who run unit assessments on all of CPAN regularly to assist detect breakage.
The toolchain can be fairly strong. The documentation syntax (POD) is a bit more verbose than I might like, but it surely yields far more helpful outcomes than doxygen or Javadoc. You can run perldoc FILENAME
to immediately see the documentation of the module you are writing. perldoc Module::Name
reveals you the precise documentation for the model of the module that you’d load out of your embrace
path and may likewise present you the supply code of that module with no need to browse deep into your filesystem.
The testcase system (the show
command and Test Anything Protocol, or TAP) is not particular to Perl and is very simple to work with (versus unit testing based mostly round language-specific object-oriented construction, or XML). Modules like Test::More
make writing the check instances really easy you can write a check suite in about the identical time it might take to check your module as soon as by hand. The testing effort barrier is so low that I’ve began utilizing TAP and the POD documentation model for my non-Perl tasks as properly.
In abstract
Perl 5 nonetheless has lots to supply regardless of the big variety of newer languages competing with it. The frontend syntax hasn’t stopped evolving, and you may enhance it nonetheless you want with customized modules. The Perl 5 engine is able to dealing with most programming issues you possibly can throw at it, and it’s even appropriate for low-level work as a “glue” layer on high of C libraries. Once you get actually accustomed to it, it could possibly even be an setting for growing C code.
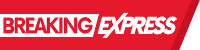