I’ve lengthy promised to put in writing in regards to the scripting language Basic and creating macros in LibreOffice. This article is dedicated to the kinds of knowledge utilized in LibreOffice Basic, and to a larger extent, descriptions of variables and the foundations for utilizing them. I’ll attempt to present sufficient data for superior in addition to novice customers.
(And, I want to thank everybody who commented on and provided suggestions on the Russian article, particularly those that helped reply tough questions.)
Variable naming conventions
Variable names can not include greater than 255 characters. They ought to begin with both upper- or lower-case letters of the Latin alphabet, and so they can embody underscores (“_”) and numerals. Other punctuation or characters from non-Latin alphabets could cause a syntax error or a BASIC runtime error if names aren’t put inside sq. brackets.
Here are some examples of appropriate variable names:
MyNumber=5MyNumber5=15
MyNumber_5=20
_MyNumber=96
[My Number]=20.5
[5MyNumber]=12
[Number,Mine]=12
[DéjàVu]="It seems that I have seen it!"
[Моя переменная]="The first has went!"
[Мой % от зделки]=zero.0001
Note: In examples that include sq. brackets, in the event you take away the brackets, macros will present a window with an error. As you possibly can see, you should use localized variable names. Whether it is smart to take action is as much as you.
Declaring variables
Strictly talking, it’s not essential to declare variables in LibreOffice Basic (apart from arrays). If you write a macro from a pair of strains to work with small paperwork, you need not declare variables, because the variable will robotically be declared because the variant kind. For longer macros or these that may work in giant paperwork, it’s strongly advisable that you just declare variables. First, it will increase the readability of the textual content. Second, it permits you to management variables that may drastically facilitate the seek for errors. Third, the variant kind may be very resource-intensive, and appreciable time is required for the hidden conversion. In addition, the variant kind doesn’t select the optimum variable kind for knowledge, which will increase the workload of pc sources.
Basic can robotically assign a variable kind by its prefix (the primary letter within the title) to simplify the work in the event you want to make use of the Hungarian notation. For this, the assertion DefXXX is used; XXX is the letter kind designation. A press release with a letter will work within the module, and it have to be specified earlier than subprograms and capabilities seem. There are 11 varieties:
DefBool - for boolean variables;
DefInt - for integer variables of kind Integer;
DefLng - for integer variables of kind Long Integer;
DefSng - for variables with a single-precision floating level;
DefDbl - for variables with double-precision floating-point kind Double;
DefCur - for variables with a hard and fast level of kind Currency;
DefStr - for string variables;
DefDate - for date and time variables;
DefVar - for variables of Variant kind;
DefObj - for object variables;
DefErr - for object variables containing error data.
If you have already got an thought of the kinds of variables in LibreOffice Basic, you most likely observed that there isn’t a Byte kind on this checklist, however there’s a unusual beast with the Error kind. Unfortunately, you simply want to recollect this; I’ve not but found why that is true. This technique is handy as a result of the kind is assigned to the variables robotically. But it doesn’t assist you to discover errors associated to typos in variable names. In addition, it won’t be attainable to specify non-Latin letters; that’s, all names of variables in sq. brackets that must be declared have to be declared explicitly.
To keep away from typos when utilizing declared variables explicitly, you should use the assertion OPTION EXPLICIT. This assertion needs to be the primary line of code within the module. All different instructions, besides feedback, needs to be positioned after it. This assertion tells the interpreter that every one variables have to be declared explicitly; in any other case, it produces an error. Naturally, this assertion makes it meaningless to make use of the Def assertion within the code.
A variable is asserted utilizing the assertion Dim. You can declare a number of variables concurrently, even differing kinds, in the event you separate their names with commas. To decide the kind of a variable with an express declaration, you should use both a corresponding key phrase or a type-declaration signal after the title. If a type-declaration signal or a key phrase will not be used after the variable, then the Variant kind is robotically assigned to it. For instance:
Dim iMyVar 'variable of Variant kind
Dim iMyVar1 As Integer, iMyVar2 As Integer 'in each circumstances Integer kind
Dim iMyVar3, iMyVar4 As Integer 'on this case the primary variable
'is Variant, and the second is Integer
Variable varieties
LibreOffice Basic helps seven lessons of variables:
- Logical variables containing one of many values: TRUE or FALSE
- Numeric variables containing numeric values. They may be integer, integer-positive, floating-point, and fixed-point
- String variables containing character strings
- Date variables can include a date and/or time within the inside format
- Object variables can include objects of various varieties and constructions
- Arrays
- Abstract kind Variant
Logical variables – Boolean
Variables of the Boolean kind can include solely one among two values: TRUE or FALSE. In the numerical equal, the worth FALSE corresponds to the quantity zero, and the worth TRUE corresponds to -1 (minus one). Any worth aside from zero handed to a variable of the Boolean kind will likely be transformed to TRUE; that’s, transformed to a minus one. You can explicitly declare a variable within the following approach:
Dim MyBoolVar As Boolean
I didn’t discover a particular image for it. For an implicit declaration, you should use the DefBool assertion. For instance:
DefBool b 'variables starting with b by default are the kind Boolean
The preliminary worth of the variable is about to FALSE. A Boolean variable requires one byte of reminiscence.
Integer variables
There are three kinds of integer variables: Byte, Integer, and Long Integer. These variables can solely include integers. When you switch numbers with a fraction into such variables, they’re rounded in line with the foundations of classical arithmetic (to not the bigger aspect, because it acknowledged within the assist part). The preliminary worth for these variables is zero (zero).
Byte
Variables of the Byte kind can include solely integer-positive values within the vary from zero to 255. Do not confuse this kind with the bodily dimension of knowledge in bytes. Although we will write down a hexadecimal quantity to a variable, the phrase “Byte” signifies solely the dimensionality of the quantity. You can declare a variable of this kind as follows:
Dim MyByteVar As Byte
There isn’t any a type-declaration signal for this kind. There isn’t any the assertion Def of this kind. Because of its small dimension, this kind will likely be most handy for a loop index, the values of which don’t transcend the vary. A Byte variable requires one byte of reminiscence.
Integer
Variables of the Integer kind can include integer values from -32768 to 32767. They are handy for quick calculations in integers and are appropriate for a loop index. % is a type-declaration signal. You can declare a variable of this kind within the following methods:
Dim MyIntegerVar%
Dim MyIntegerVar As Integer
For an implicit declaration, you should use the DefInt assertion. For instance:
DefInt i 'variables beginning with i by default have kind Integer
An Integer variable requires two bytes of reminiscence.
Long integer
Variables of the Long Integer kind can include integer values from -2147483648 to 2147483647. Long Integer variables are handy in integer calculations when the vary of kind Integer is inadequate for the implementation of the algorithm. & is a type-declaration signal. You can declare a variable of this kind within the following methods:
Dim MyLongVar&
Dim MyLongVar As Long
For an implicit declaration, you should use the DefLng assertion. For instance:
DefLng l 'variables beginning with l have Long by default
A Long Integer variable requires 4 bytes of reminiscence.
Numbers with a fraction
All variables of those varieties can take optimistic or adverse values of numbers with a fraction. The preliminary worth for them is zero (zero). As talked about above, if a quantity with a fraction is assigned to a variable succesful of containing solely integers, LibreOffice Basic rounds the quantity in line with the foundations of classical arithmetic.
Single
Single variables can take optimistic or adverse values within the vary from three.402823x10E+38 to 1.401293x10E-38. Values of variables of this kind are in single-precision floating-point format. In this format, solely eight numeric characters are saved, and the remaining is saved as an influence of ten (the quantity order). In the Basic IDE debugger, you possibly can see solely 6 decimal locations, however this can be a blatant lie. Computations with variables of the Single kind take longer than Integer variables, however they’re quicker than computations with variables of the Double kind. A kind-declaration signal is !. You can declare a variable of this kind within the following methods:
Dim MySingleVar!
Dim MySingleVar As Single
For an implicit declaration, you should use the DefSng assertion. For instance:
DefSng f 'variables beginning with f have the Single kind by default
A single variable requires 4 bytes of reminiscence.
Double
Variables of the Double kind can take optimistic or adverse values within the vary from 1.79769313486231598x10E308 to 1.0x10E-307. Why such an odd vary? Most probably within the interpreter, there are further checks that result in this case. Values of variables of the Double kind are in double-precision floating-point format and may have 15 decimal locations. In the Basic IDE debugger, you possibly can see solely 14 decimal locations, however that is additionally a blatant lie. Variables of the Double kind are appropriate for exact calculations. Calculations require extra time than the Single kind. A kind-declaration signal is #. You can declare a variable of this kind within the following methods:
Dim MyDoubleVar#
Dim MyDoubleVar As Double
For an implicit declaration, you should use the DefDbl assertion. For instance:
DefDbl d 'variables starting with d have the kind Double by default
A variable of the Double kind requires eight bytes of reminiscence.
Currency
Variables of the Currency kind are displayed as numbers with a hard and fast level and have 15 indicators within the integral a part of a quantity and Four indicators in fractional. The vary of values contains numbers from -922337203685477.6874 to +92337203685477.6874. Variables of the Currency kind are supposed for precise calculations of financial values. A kind-declaration signal is @. You can declare a variable of this kind within the following methods:
Dim MyCurrencyVar@
Dim MyCurrencyVar As Currency
For an implicit declaration, you should use the DefCur assertion. For instance:
DefCur c 'variables starting with c have the kind Currency by default
A Currency variable requires eight bytes of reminiscence.
String
Variables of the String kind can include strings during which every character is saved because the corresponding Unicode worth. They are used to work with textual data, and along with printed characters (symbols), they will additionally include non-printable characters. I have no idea the utmost dimension of the road. Mike Kaganski experimentally set the worth to 2147483638 characters, after which LibreOffice falls. This corresponds to nearly Four gigabytes of characters. A kind-declaration signal is $. You can declare a variable of this kind within the following methods:
Dim MyStringVar$
Dim MyStringVar As String
For an implicit declaration, you should use the DefStr assertion. For instance:
DefStr s 'variables beginning with s have the String kind by default
The preliminary worth of those variables is an empty string (“”). The reminiscence required to retailer string variables depends upon the variety of characters within the variable.
Date
Variables of the Date kind can include solely date and time values saved within the inside format. In truth, this inside format is the double-precision floating-point format (Double), the place the integer half is the variety of days, and the fractional is a part of the day (that’s, zero.00001157407 is one second). The worth zero is the same as 30.12.1899. The Basic interpreter robotically converts it to a readable model when outputting, however not when loading. You can use the Dateserial, Datevalue, Timeserial, or Timevalue capabilities to shortly convert to the interior format of the Date kind. To extract a sure half from a variable within the Date format, you should use the Day, Month, Year, Hour, Minute, or Second capabilities. The inside format permits us to match the date and time values by calculating the distinction between two numbers. There isn’t any a type-declaration sing for the Date kind, so in the event you explicitly outline it, you might want to use the Date key phrase.
Dim MyDateVar As Date
For an implicit declaration, you should use the DefDate assertion. For instance:
DefDate y 'variables beginning with y have the Date kind by default
A Date variable requires eight bytes of reminiscence.
Types of object variables
We can take two variables kinds of LibreOffice Basic to Objects.
Objects
Variables of the Object kind are variables that retailer objects. In normal, the item is any remoted a part of this system that has the construction, properties, and strategies of entry and knowledge processing. For instance, a doc, a cell, a paragraph, and dialog packing containers are objects. They have a reputation, dimension, properties, and strategies. In flip, these objects additionally encompass objects, which in flip can even encompass objects. Such a “pyramid” of objects is commonly known as an object mannequin, and it permits us, when creating small objects, to mix them into bigger ones. Through a bigger object, we’ve entry to smaller ones. This permits us to function with our paperwork, to create and course of them whereas abstracting from a particular doc. There isn’t any a type-declaration sing for the Object kind, so for an express definition, you might want to use the Object key phrase.
Dim MyObjectVar As Object
For an implicit declaration, you should use the DefObj assertion. For instance:
DefObj o 'variables starting with o have the kind Object by default
The variable of kind Object doesn’t retailer in itself an object however is simply a reference to it. The preliminary worth for this kind of variables is Null.
Structures
The construction is basically an object. If you look within the Basic IDE debugger, most (however not all) are the Object kind. Some aren’t; for instance, the construction of the Error has the kind Error. But roughly talking, the constructions in LibreOffice Basic are merely grouped into one object variable, with out particular entry strategies. Another vital distinction is that when declaring a variable of the Structure kind, we should specify its title, fairly than the Object. For instance, if MyNewStructure is the title of a construction, the declaration of its variable will appear to be this:
Dim MyStructureVar As MyNewStructure
There are a number of built-in constructions, however the consumer can create private ones. Structures may be handy when we have to function with units of heterogeneous data that needs to be handled as a single complete. For instance, to create a tPerson construction:
Type tPerson
Name As String
Age As Integer
Weight As Double
End Type
The definition of the construction ought to go earlier than subroutines and capabilities that use it.
To fill a construction, you should use, for instance, the built-in construction com.solar.star.beans.PropertyValue:
Dim oProp As New com.solar.star.beans.PropertyValue
OProp.Name = "Age" 'Set the Name
OProp.Value = "Amy Boyer" 'Set the Property
For a less complicated filling of the construction, you should use the With operator.
Dim oProp As New com.solar.star.beans.PropertyValue
With oProp
.Name = "Age" 'Set the Name
.Value = "Amy Boyer" 'Set the Property
End With
The preliminary worth is just for every variable within the construction and corresponds to the kind of the variable.
Variant
This is a digital kind of variables. The Variant kind is robotically chosen for the info to be operated on. The solely downside is that the interpreter doesn’t want to avoid wasting our sources, and it doesn’t supply probably the most optimum variants of variable varieties. For instance, it doesn’t know that 1 may be written in Byte, and 100000 in Long Integer, though it reproduces a kind if the worth is handed from one other variable with the declared kind. Also, the transformation itself is kind of resource-intensive. Therefore, this kind of variable is the slowest of all. If you might want to declare this type of variable, you should use the Variant key phrase. But you possibly can omit the kind description altogether; the Variant kind will likely be assigned robotically. There isn’t any a type-declaration signal for this kind.
Dim MyVariantVar
Dim MyVariantVar As Variant
For an implicit declaration, you should use the DefVar assertion. For instance:
DefVar v 'variables beginning with v have the Variant kind by default
This variables kind is assigned by default to all undeclared variables.
Arrays
Arrays are a particular kind of variable within the type of a knowledge set, paying homage to a mathematical matrix, besides that the info may be of various varieties and permit one to entry its components by index (ingredient quantity). Of course, a one-dimensional array will likely be just like a column or row, and a two-dimensional array will likely be like a desk. There is one characteristic of arrays in LibreOffice Basic that distinguishes it from different programming languages. Since we’ve an summary kind of variant, then the weather of the array don’t must be homogeneous. That is, if there may be an array MyArray and it has three components numbered from zero to 2, and we write the title within the first ingredient of MyArray(zero), the age within the second MyArray(1), and the burden within the third MyArray(2), we will have, respectively, the next kind values: String for MyArray(zero), Integer for MyArray(1), and Double for MyArray(2). In this case, the array will resemble a construction with the flexibility to entry the ingredient by its index. Array components will also be homogeneous: Other arrays, objects, constructions, strings, or some other knowledge kind can be utilized in LibreOffice Basic.
Arrays have to be declared earlier than they’re used. Although the index area may be within the vary of kind Integer—from -32768 to 32767—by default, the preliminary index is chosen as zero. You can declare an array in a number of methods:
Dim MyArrayVar(5) as string | String array with 6 components from zero to five |
Dim MyArrayVar$(5) | Same because the earlier |
Dim MyArrayVar(1 To 5) as string | String array with 5 components from 1 to five |
Dim MyArrayVar(5,5) as string | Two-dimensional array of rows with 36 components with indexes in every stage from zero to five |
Dim MyArrayVar$(-Four To 5, -Four To 5) | Two-dimensional strings array with 100 components with indexes in every stage from -Four to five |
Dim MyArrayVar() | Empty array of the Variant kind |
You can change the decrease sure of an array (the index of the primary ingredient of the array) by default utilizing the Option Base assertion; that have to be specified earlier than utilizing subprograms, capabilities, and defining consumer constructions. Option Base can take solely two values, zero or 1, which should observe instantly after the key phrases. The motion applies solely to the present module.
Learn extra
If you might be simply beginning out in programming, Wikipedia offers normal details about the array, construction, and lots of different matters.
For a extra in-depth research of LibreOffice Basic, Andrew Pitonyak’s web site is a high useful resource, as is the Basic Programmer’s guide. You can even use the LibreOffice online help. Completed widespread macros may be discovered within the Macros part of The Document Foundation’s wiki, the place you may also discover further hyperlinks on the subject.
For extra suggestions, or to ask questions, go to Ask LibreOffice and OpenOffice forum.
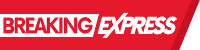