In my earlier article, I defined easy methods to make Python modular by utilizing capabilities, creating modules, or each. Functions are invaluable to keep away from repeating code you propose to make use of a number of occasions, and modules be sure that you need to use your code throughout totally different initiatives. But there’s one other part to modularity: the category.
If you’ve got heard the time period object-oriented programming, then you will have some notion of the aim courses serve. Programmers have a tendency to think about a category as a digital object, typically with a direct correlation to one thing within the bodily world, and different occasions as a manifestation of some programming idea. Either manner, the concept is that you could create a category if you need to create “objects” inside a program for you or different components of this system to work together with.
Templates with out courses
Assume you are writing a sport set in a fantasy world, and also you want this utility to have the ability to drum up quite a lot of baddies to carry some pleasure into your gamers’ lives. Knowing quite a bit about capabilities, you would possibly suppose this feels like a textbook case for capabilities: code that must be repeated usually however is written as soon as with allowance for variations when referred to as.
Here’s an instance of a purely function-based implementation of an enemy generator:
#!/usr/bin/env python3import random
def enemy(ancestry,gear):
enemy=ancestry
weapon=gear
hp=random.randrange(zero,20)
ac=random.randrange(zero,20)
return [enemy,weapon,hp,ac]def struggle(tgt):
print("You take a swing at the " + tgt[zero] + ".")
hit=random.randrange(zero,20)
if hit > tgt[three]:
print("You hit the " + tgt[zero] + " for " + str(hit) + " damage!")
tgt[2] = tgt[2] - hit
else:
print("You missed.")foe=enemy("troll","great axe")
print("You meet a " + foe[zero] + " wielding a " + foe[1])
print("Type the a key and then RETURN to attack.")whereas True:
motion=enter()if motion.decrease() == "a":
struggle(foe)if foe[2] < 1:
print("You killed your foe!")
else:
print("The " + foe[zero] + " has " + str(foe[2]) + " HP remaining")
The enemy perform creates an enemy with a number of attributes, corresponding to ancestry, a weapon, well being factors, and a protection score. It returns an inventory of every attribute, representing the sum complete of the enemy.
In a way, this code has created an object, regardless that it is not utilizing a category but. Programmers name this “enemy” an object as a result of the end result (an inventory of strings and integers, on this case) of the perform represents a singular however complicated factor within the sport. That is, the strings and integers within the checklist aren’t arbitrary: collectively, they describe a digital object.
When writing a group of descriptors, you employ variables so you need to use them any time you need to generate an enemy. It’s a bit of like a template.
In the instance code, when an attribute of the thing is required, the corresponding checklist merchandise is retrieved. For occasion, to get the ancestry of an enemy, the code seems at foe[0], for well being factors, it seems at foe[2] for well being factors, and so forth.
There’s nothing essentially flawed with this method. The code runs as anticipated. You may add extra enemies of various varieties, you could possibly create an inventory of enemy varieties and randomly choose from the checklist throughout enemy creation, and so forth. It works properly sufficient, and actually Lua makes use of this precept very successfully to approximate an object-oriented mannequin.
However, there’s typically extra to an object than only a checklist of attributes.
The manner of the thing
In Python, all the things is an object. Anything you create in Python is an occasion of some predefined template. Even fundamental strings and integers are derivatives of the Python kind class. You can witness this for your self an interactive Python shell:
>>> foo=three
>>> kind(foo)
<class 'int'>
>>> foo="bar"
>>> kind(foo)
<class 'str'>
When an object is outlined by a category, it’s greater than only a assortment of attributes. Python courses have capabilities all their very own. This is handy, logically, as a result of actions that pertain solely to a sure class of objects are contained inside that object’s class.
In the instance code, the struggle code is a perform of the principle utility. That works advantageous for a easy sport, however in a fancy one, there could be extra than simply gamers and enemies within the sport world. There could be townsfolk, livestock, buildings, forests, and so forth, and none of them ever want entry to a struggle perform. Placing code for fight in an enemy class means your code is healthier organized; and in a fancy utility, that is a big benefit.
Furthermore, every class has privileged entry to its personal native variables. An enemy’s well being factors, for example, is not information that ought to ever change besides by some perform of the enemy class. A random butterfly within the sport shouldn’t unintentionally cut back an enemy’s well being to zero. Ideally, even with out courses, that might by no means occur, however in a fancy utility with a number of shifting components, it is a highly effective trick of the commerce to make sure that components that need not work together with each other by no means do.
Python courses are additionally topic to rubbish assortment. When an occasion of a category is now not used, it’s moved out of reminiscence. You could by no means know when this occurs, however you have a tendency to note when it does not occur as a result of your utility takes up extra reminiscence and runs slower than it ought to. Isolating information units into courses helps Python observe what’s in use and what’s now not wanted.
Classy Python
Here’s the identical easy fight sport utilizing a category for the enemy:
#!/usr/bin/env python3import random
class Enemy():
def __init__(self,ancestry,gear):
self.enemy=ancestry
self.weapon=gear
self.hp=random.randrange(10,20)
self.ac=random.randrange(12,20)
self.alive=Truedef struggle(self,tgt):
print("You take a swing at the " + self.enemy + ".")
hit=random.randrange(zero,20)if self.alive and hit > self.ac:
print("You hit the " + self.enemy + " for " + str(hit) + " damage!")
self.hp = self.hp - hit
print("The " + self.enemy + " has " + str(self.hp) + " HP remaining")
else:
print("You missed.")if self.hp < 1:
self.alive=False# sport begin
foe=Enemy("troll","great axe")
print("You meet a " + foe.enemy + " wielding a " + foe.weapon)# important loop
whereas True:
print("Type the a key and then RETURN to attack.")
motion=enter()if motion.decrease() == "a":
foe.struggle(foe)
if foe.alive == False:
print("You have won...this time.")
exit()
This model of the sport handles the enemy as an object containing the identical attributes (ancestry, weapon, well being, and protection), plus a brand new attribute measuring whether or not the enemy has been vanquished but, in addition to a perform for fight.
The first perform of a category is a particular perform referred to as (in Python) an init, or initialization, perform. This is much like a constructor in different languages; it creates an occasion of the category, which is identifiable to you by its attributes and to no matter variable you employ when invoking the category (foe within the instance code).
Self and sophistication cases
The class’ capabilities settle for a brand new type of enter you do not see exterior of courses: self. If you do not embrace self, then Python has no manner of figuring out which occasion of the category to make use of if you name a category perform. It’s like difficult a single orc to a duel by saying “I’ll fight the orc” in a room filled with orcs; no one is aware of which one you are referring to, and so dangerous issues occur.
Each attribute created inside a category is prepended with the self notation, which identifies that variable as an attribute of the category. Once an occasion of a category is spawned, you swap out the self prefix with the variable representing that occasion. Using this system, you could possibly problem only one orc to a duel in a room filled with orcs by saying “I’ll fight the gorblar.orc”; when Gorblar the Orc hears gorblar.orc, he is aware of which orc you are referring to (himself), and so that you get a good struggle as an alternative of a brawl. In Python:
gorblar=Enemy("orc","sword")
print("The " + gorblar.enemy + " has " + str(gorblar.hp) + " remaining.")
Instead of seeking to foe[0] (as within the useful instance) or gorblar[0] for the enemy kind, you retrieve the category attribute (gorblar.enemy or gorblar.hp or no matter worth for no matter object you want).
Local variables
If a variable in a category is just not prepended with the self key phrase, then it’s a native variable, simply as in any perform. For occasion, it doesn’t matter what you do, you can not entry the hit variable exterior the Enemy.struggle class:
>>> print(foe.hit)
Traceback (most up-to-date name final):
File "./enclass.py", line 38, in <module>
print(foe.hit)
AttributeError: 'Enemy' object has no attribute 'hit'>>> print(foe.struggle.hit)
Traceback (most up-to-date name final):
File "./enclass.py", line 38, in <module>
print(foe.struggle.hit)
AttributeError: 'perform' object has no attribute 'hit'
The hit variable is contained throughout the Enemy class, and solely “lives” lengthy sufficient to serve its function in fight.
More modularity
This instance makes use of a category in the identical textual content doc as your important utility. In a fancy sport, it is simpler to deal with every class nearly as if it had been its personal self-standing utility. You see this when a number of builders work on the identical utility: one developer works on a category, and the opposite works on the principle program, and so long as they convey with each other about what attributes the category will need to have, the 2 code bases may be developed in parallel.
To make this instance sport modular, break up it into two information: one for the principle utility and one for the category. Were it a extra complicated utility, you may need one file per class, or one file per logical teams of courses (for example, a file for buildings, a file for pure environment, a file for enemies and NPCs, and so forth).
Save one file containing simply the Enemy class as enemy.py and one other file containing all the things else as important.py.
Here’s enemy.py:
import randomclass Enemy():
def __init__(self,ancestry,gear):
self.enemy=ancestry
self.weapon=gear
self.hp=random.randrange(10,20)
self.stg=random.randrange(zero,20)
self.ac=random.randrange(zero,20)
self.alive=Truedef struggle(self,tgt):
print("You take a swing at the " + self.enemy + ".")
hit=random.randrange(zero,20)if self.alive and hit > self.ac:
print("You hit the " + self.enemy + " for " + str(hit) + " damage!")
self.hp = self.hp - hit
print("The " + self.enemy + " has " + str(self.hp) + " HP remaining")
else:
print("You missed.")if self.hp < 1:
self.alive=False
Here’s important.py:
#!/usr/bin/env python3import enemy as en
# sport begin
foe=en.Enemy("troll","great axe")
print("You meet a " + foe.enemy + " wielding a " + foe.weapon)# important loop
whereas True:
print("Type the a key and then RETURN to attack.")motion=enter()
if motion.decrease() == "a":
foe.struggle(foe)if foe.alive == False:
print("You have won...this time.")
exit()
Importing the module enemy.py is finished very particularly with a press release that refers back to the file of courses as its title with out the .py extension, adopted by a namespace designator of your selecting (for instance, import enemy as en). This designator is what you employ within the code when invoking a category. Instead of simply utilizing Enemy(), you preface the category with the designator of what you imported, corresponding to en.Enemy.
All of those file names are fully arbitrary, though not unusual in precept. It’s a standard conference to call the a part of the applying that serves because the central hub important.py, and a file filled with courses is commonly named in lowercase with the courses inside it, every starting with a capital letter. Whether you observe these conventions does not have an effect on how the applying runs, nevertheless it does make it simpler for knowledgeable Python programmers to shortly decipher how your utility works.
There’s some flexibility in the way you construction your code. For occasion, utilizing the code pattern, each information have to be in the identical listing. If you need to bundle simply your courses as a module, then it’s essential to create a listing referred to as, for example, mybad and transfer your courses into it. In important.py, your import assertion adjustments a bit of:
from mybad import enemy as en
Both programs produce the identical outcomes, however the latter is greatest if the courses you’ve got created are generic sufficient that you simply suppose different builders may use them of their initiatives.
Regardless of which you select, launch the modular model of the sport:
$ python3 ./important.py
You meet a troll wielding an excellent axe
Type the a key and then RETURN to assault.
a
You take a swing on the troll.
You missed.
Type the a key and then RETURN to assault.
a
You take a swing on the troll.
You hit the troll for eight harm!
The troll has four HP remaining
Type the a key and then RETURN to assault.
a
You take a swing on the troll.
You hit the troll for 11 harm!
The troll has -7 HP remaining
You have received...this time.
The sport works. It’s modular. And now you already know what it means for an utility to be object-oriented. But most significantly, you already know to be particular when difficult an orc to a duel.
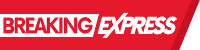