Go is a contemporary programming language that derives a lot of its historical past from the C programming language. As such, Go is more likely to really feel acquainted to anybody who writes applications in C. Go makes it easy to write new programs whereas feeling acquainted to C programmers however avoiding most of the widespread pitfalls of the C programming language.
This article compares a easy C and Go program that provides the numbers from one to 10. Because this program makes use of solely small values, the numbers will not develop to be too large, so that they solely use plain integer variables. Loops like this are quite common in programming, so this straightforward program makes it simple to match C and Go.
How to do loops in C
The primary loop in C is the for
loop, which lets you iterate by way of a set of values. The primary syntax of the for
loop is:
for (begin situation ; finish situation ; motion after every iteration) { issues to do contained in the loop ; }
You can write a for
loop that prints the numbers from one to 10 by setting the beginning situation to rely = 1
and the ending situation to rely <= 10
. That begins the loop with the rely
variable equal to at least one. The ending situation means the loop continues so long as the rely
variable is lower than or equal to 10.
After every iteration, you employ rely = rely + 1
to increment the worth of the rely
variable by one. Inside the loop, you should utilize printf
to print the worth of the rely
variable:
for (rely = 1; rely <= 10; rely = rely + 1) {
printf("%dn", rely);
}
A typical conference in C programming is ++
, which implies “add one to something.” If you write rely++
, that is the identical as rely = rely + 1
. Most C programmers would use this to put in writing the for
loop utilizing rely++
for the motion after every iteration, like this:
for (rely = 1; rely <= 10; rely++) {
printf("%dn", rely);
}
Here’s a pattern program that provides the numbers from one to 10, then prints the end result. Use the for
loop to iterate by way of the numbers, however as a substitute of printing the quantity, add the numbers to the sum
variable:
#embrace <stdio.h>
int fundamental() {
int sum;
int rely;
places("adding 1 to 10 ..");
sum = 0;
for (rely = 1; rely <= 10; rely++) {
sum = sum + rely;
}
This program makes use of two totally different C features to print outcomes to the person. The places
perform prints a string that is inside quotes. If that you must print plain textual content, places
is an effective option to do it.
The printf
function prints formatted output utilizing particular characters in a format string. The printf
perform can print a lot of totally different sorts of values. The key phrase %d
prints a decimal (or integer) worth.
If you compile and run this program, you see this output:
including 1 to 10 ..
The sum is 55
How to do loops in Go
Go supplies for
loops which might be similar to C for
loops. The for loop from the C program could be immediately translated to a Go for
loop with an identical illustration:
for rely = 1; rely <= 10; rely++ {
fmt.Printf("%dn", rely)
}
With this loop, you possibly can write a direct transition to Go of the pattern program:
package deal fundamental
import "fmt"
func fundamental() {
var sum, rely int
fmt.Println("adding 1 to 10 ..")
for rely = 1; rely <= 10; rely++ {
sum = sum + rely
}
fmt.Printf("The sum is %dn", sum)
}
While the above is actually a sound and proper Go, it is not essentially the most idiomatic Go. To be idiomatic is to make use of expressions which might be pure to a local speaker. A aim of any language is efficient communication, this contains programming languages. When transitioning between programming languages, it’s also necessary to acknowledge that what’s typical in a single programming language is probably not precisely so in one other, regardless of any outward similarities.
To replace the above program utilizing the extra idiomatic Go, you may make a few small modifications:
-
Use the
+=
add-to-self operator to put in writingsum = sum + rely
extra succinctly assum += rely
. C can use this fashion, as properly. -
Use the assign-and-infer-type operator to say
rely := 1
moderately thanvar rely int
adopted byrely = 1
. The:=
syntax each defines and initializes the rely variable. -
Move the declaration of
rely
into thefor
loop header itself. This reduces a little bit of cognitive overhead, and will increase readability by lowering the variety of variables the programmer should mentally account for at any time. This change additionally will increase security by declaring variables as shut as attainable to their use and within the smallest scope attainable. This reduces the probability of unintentional manipulation because the code evolves.
The mixture of the adjustments described above ends in:
package deal fundamental
import "fmt"
func fundamental() {
fmt.Println("adding 1 to 10 ..")
var sum int
for rely := 1; rely <= 10; rely++ {
sum += rely
}
fmt.Printf("The sum is %dn", sum)
}
You can experiment with this pattern program within the Go playground with this link to go.dev.
C and Go are comparable, however totally different
By writing the identical program in two programming languages, you possibly can see that C and Go are comparable, however totally different. Here are a couple of necessary ideas to bear in mind when transitioning from C to Go:
-
In C, each programming instruction should finish with a semicolon. This tells the compiler the place one assertion ends and the subsequent one begins. In Go, semicolons are legitimate however nearly all the time inferred.
-
While most trendy C compilers initialize variables to a zero worth for you, the C specification says that variables get no matter worth was in reminiscence on the time. Go values are all the time initialized to their zero worth. This helps make Go a extra reminiscence protected language. This distinction turns into much more attention-grabbing with pointers.
-
Note using the Go package deal specifier on imported identifiers. For instance,
fmt
for features that implement formatted enter and output, much like C’sprintf
andscanf
fromstdio.h
. Thefmt
package deal is documented in pkg.go.dev/fmt. -
In Go, the
fundamental
perform all the time returns with an exit code of 0. If you want to return another worth, you could nameos.Exit(n)
the place n is usually 1 to point an error. This could be known as from wherever, not simplyfundamental
, to terminate this system. You can do the identical in C utilizing theexit(n)
perform, outlined instdlib.h
.
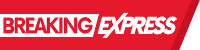