I’m no professional on educating programming, however after I need to get higher at one thing, I attempt to discover a option to have enjoyable with it. For instance, after I wished to get higher at shell scripting, I made a decision to follow by programming a model of the Minesweeper sport in Bash.
If you’re an skilled Bash programmer and need to hone your abilities whereas having enjoyable, observe alongside to write down your individual model of Minesweeper within the terminal. The full supply code is discovered on this GitHub repository.
Getting prepared
Before I began writing any code, I outlined the substances I wanted to create my sport:
- Print a minefield
- Create the gameplay logic
- Create logic to find out the accessible minefield
- Keep depend of obtainable and found (extracted) mines
- Create the endgame logic
Print a minefield
In Minesweeper, the sport world is a 2D array (columns and rows) of hid cells. Each cell could or could not include an explosive mine. The participant’s goal is to disclose cells that include no mine, and to by no means reveal a mine. Bash model of the sport makes use of a 10×10 matrix, carried out utilizing easy bash arrays.
First, I assign some random variables. These are the areas that mines could possibly be positioned on the board. By limiting the variety of areas, will probably be straightforward to construct on high of this. The logic could possibly be higher, however I wished to maintain the sport trying easy and a bit immature. (I wrote this for enjoyable, however I might fortunately welcome your contributions to make it look higher.)
The variables beneath are some default variables, declared to name randomly for subject placement, just like the variables a-g, we’ll use them to calculate our extractable mines:
# variables
rating=Zero # will probably be used to retailer the rating of the sport
# variables beneath will probably be used to randomly get the extract-able cells/fields from our mine.
a="1 10 -10 -1"
b="-1 0 1"
c="0 1"
d="-1 0 1 -2 -3"
e="1 2 20 21 10 0 -10 -20 -23 -2 -1"
f="1 2 3 35 30 20 22 10 0 -10 -20 -25 -30 -35 -3 -2 -1"
g="1 4 6 9 10 15 20 25 30 -30 -24 -11 -10 -9 -8 -7"
#
# declarations
declare -a room # declare an array room, it should symbolize every cell/subject of our mine.
Next, I print my board with columns (Zero-9) and rows (a-j), forming a 10×10 matrix to function the minefield for the sport. (M[10][10] is a 100-value array with indexes Zero-99.) If you need to know extra about Bash arrays, learn You don’t know Bash: An introduction to Bash arrays.
Lets name it a operate, plough, we print the header first: two clean traces, the column headings, and a line to stipulate the highest of the enjoying subject:
printf 'nn'
printf '%s' " a b c d e f g h i j"
printf 'n %sn' "-----------------------------------------"
Next, I set up a counter variable, referred to as r, to maintain observe of what number of horizontal rows have been populated. Note that, we’ll use the identical counter variable ‘r‘ as our array index later within the sport code. In a Bash for loop, utilizing the seq command to increment from Zero to 9, I print a digit (d%) to symbolize the row quantity ($row, which is outlined by seq):
r=Zero # our counter
for row in $(seq Zero 9); do
printf '%d ' "$row" # print the row numbers from Zero-9
Before we transfer forward from right here, lets test what we now have made until now. We printed sequence [a-j] horizontally first after which we printed row numbers in a spread [0-9], we will probably be utilizing these two ranges to behave as our customers enter coordinates to find the mine to extract.
Next, Within every row, there’s a column intersection, so it is time to open a brand new for loop. This one manages every column, so it basically generates every cell within the enjoying subject. I’ve added some helper capabilities that you would be able to see the complete definition of within the supply code. For every cell, we’d like one thing to make the sector appear to be a mine, so we initialize the empty ones with a dot (.), utilizing a customized operate referred to as is_null_field. Also, we’d like an array variable to retailer the worth for every cell, we’ll use the predefined world array variable room together with an index variable r. As r increments, we iterate over the cells, dropping mines alongside the best way.
for col in $(seq Zero 9); do
((r+=1)) # increment the counter as we transfer ahead in column sequence
is_null_field $r # assume a operate which is able to test, if the sector is empty, in that case, initialize it with a dot(.)
printf '%s e[33mpercentse[0m ' "|" "$" # lastly print the separator, be aware that, the primary worth of $ will probably be '.', as it's simply initialized.
#shut col loop
achieved
Finally, I maintain the board well-defined by enclosing the underside of every row with a line, after which shut the row loop:
printf '%sn' "|" # print the road finish separator
printf ' %sn' "-----------------------------------------"
# shut row for loop
achieved
printf 'nn'
The full plough operate seems to be like:
plough()
" "$"
achieved
printf '%sn' "
It took me a while to determine on needing the is_null_field, so let’s take a better have a look at what it does. We want a reliable state from the start of the sport. That alternative is unfair–it might have been a quantity or any character. I made a decision to imagine the whole lot was declared as a dot (.) as a result of I imagine it makes the gameboard look fairly. Here’s what that appears like:
is_null_field()
Now that, I have all of the cells in our mine initialized, I get a depend of all accessible mines by declaring and later calling a easy operate proven beneath:
get_free_fields()
Here is the printed minefield, the place [a-j] are columns, and [Zero-9] are rows.
Create the logic to drive the participant
The participant logic reads an choice from stdin as a coordinate to the mines and extracts the precise subject on the minefield. It makes use of Bash’s parameter expansion to extract the column and row inputs, then feeds the column to a swap that factors to its equal integer notation on the board, to grasp this, see the values getting assigned to variable ‘o’ within the swap case assertion beneath. For occasion, a participant would possibly enter c3, which Bash splits into two characters: c and three. For simplicity, I am skipping over how invalid entry is dealt with.
colm=$ # get the primary char, the alphabet
ro=$ # get the second char, the digit
case $colm in
a ) o=1;; # lastly, convert the alphabet to its equal integer notation.
b ) o=2;;
c ) o=three;;
d ) o=four;;
e ) o=5;;
f ) o=6;;
g ) o=7;;
h ) o=eight;;
i ) o=9;;
j ) o=10;;
esac
Then it calculates the precise index and assigns the index of the enter coordinates to that subject.
There can also be a number of use of shuf command right here, shuf is a Linux utility designed to supply a random permutation of knowledge the place the -i choice denotes indexes or potential ranges to shuffle and -n denotes the utmost quantity or output given again. Double parentheses enable for mathematical evaluation in Bash, and we’ll use them closely right here.
Let’s assume our earlier instance obtained c3 through stdin. Then, ro=three and o=three from above swap case assertion transformed c to its equal integer, put it into our formulation to calculate last index ‘i’.
i=$(((ro*10)+o)) # Follow BODMAS rule, to calculate last index.
is_free_field $i $(shuf -i Zero-5 -n 1) # name a customized operate that checks if the ultimate index worth factors to a an empty/free cell/subject.
Walking by way of this math to grasp how the ultimate index ‘i‘ is calculated:
i=$(((ro*10)+o))
i=$(((three*10)+three))=$((30+three))=33
The last index worth is 33. On our board, printed above, the ultimate index factors to 33rd cell and that needs to be third (ranging from Zero, in any other case 4th) row and third (C) column.
Create the logic to find out the accessible minefield
To extract a mine, after the coordinates are decoded and the index is discovered, this system checks whether or not that subject is obtainable. If it is not, this system shows a warning, and the participant chooses one other coordinate.
In this code, a cell is obtainable if it comprises a dot (.) character. Assuming it is accessible, the worth within the cell is reset and the rating is up to date. If a cell is unavailable as a result of it doesn’t include a dot, then a variable not_allowed is ready. For brevity, I go away it to you to have a look at the supply code of the sport for the contents of the warning statement within the sport logic.
is_free_field()
native f=$1
native val=$2
not_allowed=Zero
if [[ "$" = "." ]]; then
room[$f]=$val
rating=$((rating+val))
else
not_allowed=1
fi
If the coordinate entered is obtainable, the mine is found, as proven beneath. When h6 is supplied as enter, some values at random populated on our minefields, these values are added to customers rating after the minutes are extracted.
Now bear in mind the variables we declared firstly, [a-g], I’ll now use them right here to extract random mines assigning their worth to the variable m utilizing Bash indirection. So, relying upon the enter coordinates, this system picks a random set of extra numbers (m) to calculate the extra fields to be populated (as proven above) by including them to the unique enter coordinates, represented right here by i (calculated above).
Please be aware the character X in beneath code snippet, is our sole GAME-OVER set off, we added it to our shuffle listing to seem at random, with the fantastic thing about shuf command, it may possibly seem after any variety of possibilities or could not even seem for our fortunate profitable consumer.
m=$(shuf -e a b c d e f g X -n 1) # add an additional char X to the shuffle, when m=X, its GAMEOVER
if [[ "$m" != "X" ]]; then # X will probably be our explosive mine(GAME-OVER) set off
for restrict in $!m; do # !m represents the worth of worth of m
subject=$(shuf -i Zero-5 -n 1) # once more get a random quantity and
index=$((i+restrict)) # add values of m to our index and calculate a brand new index until m reaches its final component.
is_free_field $index $subject
achieved
I need all revealed cells to be contiguous to the cell chosen by the participant.
Keep a depend of obtainable and extracted mines
The program must maintain observe of obtainable cells within the minefield; in any other case, it retains asking the participant for enter even after all of the cells have been revealed. To implement this, I create a variable referred to as free_fields, initially setting it to Zero. In a for loop outlined by the remaining variety of accessible cells/fields in our minefields. If a cell comprises a dot (.), then the depend of free_fields is incremented.
get_free_fields()
free_fields=Zero
for n in $(seq 1 $#room[@]); do
if [[ "$room[$n]" = "." ]]; then
((free_fields+=1))
fi
achieved
Wait, what if, the free_fields=Zero? That means, our consumer had extracted all of the mines. Please be at liberty to have a look at the exact code to grasp higher.
if [[ $free_fields -eq Zero ]]; then # properly which means you extracted all of the mines.
printf 'nntpercents: %s %dnn' "You Win" "you scored" "$rating"
exit Zero
fi
Create the logic for Gameover
For the Gameover scenario, we print to the center of the terminal utilizing some nifty logic that I go away it to the reader to discover the way it works.
if [[ "$m" = "X" ]]; then
g=Zero # to make use of it in parameter growth
room[$i]=X # override the index and print X
for j in 42..49; do # in the midst of the minefields,
out="gameover"
ok=$out:$g:1 # print one alphabet in every cell
room[$j]=$ok^^
((g+=1))
achieved
fi
Finally, we will print the 2 traces that are most awaited.
if [[ "$m" = "X" ]]; then
printf 'nntpercents: %s %dn' "GAMEOVER" "you scored" "$rating"
printf 'nntpercentsnn' "You have been simply $free_fields mines away."
exit Zero
fi
That’s it, people! If you need to know extra, entry the supply code for this Minesweeper sport and different video games in Bash from my GitHub repo. I hope it offers you some inspiration to study extra Bash and to have enjoyable whereas doing so.
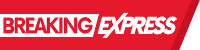