Early annually, TIOBE proclaims its Programming Language of The Year. When its newest annual TIOBE index report got here out, I used to be by no means stunned to see Python again winning the title, which was primarily based on capturing essentially the most search engine rating factors (particularly on Google, Bing, Yahoo, Wikipedia, Amazon, YouTube, and Baidu) in 2018.
Adding weight to TIOBE’s findings, earlier this yr, almost 90,000 builders took Stack Overflow’s annual Developer Survey, which is the most important and most complete survey of people that code all over the world. The foremost takeaway from this yr’s outcomes was:
“Python, the fastest-growing major programming language, has risen in the ranks of programming languages in our survey yet again, edging out Java this year and standing as the second most loved language (behind Rust).”
Ever since I began programming and exploring totally different languages, I’ve seen admiration for Python hovering excessive. Since 2003, it has constantly been among the many high 10 hottest programming languages. As TIOBE’s report said:
“It is the most frequently taught first language at universities nowadays, it is number one in the statistical domain, number one in AI programming, number one in scripting and number one in writing system tests. Besides this, Python is also leading in web programming and scientific computing (just to name some other domains). In summary, Python is everywhere.”
There are a number of causes for Python’s speedy rise, bloom, and dominance in a number of domains, together with net improvement, scientific computing, testing, knowledge science, machine studying, and extra. The causes embody its readable and maintainable code; in depth assist for third-party integrations and libraries; modular, dynamic, and transportable construction; versatile programming; studying ease and assist; user-friendly knowledge constructions; productiveness and velocity; and, most vital, neighborhood assist. The numerous utility of Python is a results of its mixed options, which give it an edge over different languages.
But in my view, the comparative simplicity of its syntax and the staggering flexibility it gives builders coming from many different languages win the cake. Very few languages can match Python’s potential to evolve to a developer’s coding model slightly than forcing her or him to code in a specific approach. Python lets extra superior builders use the model they really feel is greatest suited to resolve a specific drawback.
While working with Python, you’re like a snake charmer. This means that you can benefit from Python’s promise to supply a non-conforming surroundings for builders to code within the model greatest fitted to a specific state of affairs and to make the code extra readable, testable, and coherent.
Python programming paradigms
Python helps 4 foremost programming paradigms: crucial, practical, procedural, and object-oriented. Whether you agree that they’re legitimate and even helpful, Python strives to make all 4 out there and dealing. Before we dive in to see which programming paradigm is most fitted for particular use circumstances, it’s a good time to do a fast overview of them.
Imperative programming paradigm
The imperative programming paradigm makes use of the crucial temper of pure language to specific instructions. It executes instructions in a step-by-step method, identical to a sequence of verbal instructions. Following the “how-to-solve” strategy, it makes direct modifications to the state of this system; therefore it is usually known as the stateful programming mannequin. Using the crucial programming paradigm, you may shortly write quite simple but elegant code, and it’s super-handy for duties that contain knowledge manipulation. Owing to its comparatively slower and sequential execution technique, it can’t be used for complicated or parallel computations.
Consider this instance process, the place the objective is to take a listing of characters and concatenate it to kind a string. A approach to do it in an crucial programming model could be one thing like:
>>> sample_characters = ['p','y','t','h','o','n']
>>> sample_string = ''
>>> sample_string
''
>>> sample_string = sample_string + sample_characters[zero]
>>> sample_string
'p'
>>> sample_string = sample_string + sample_characters[1]
>>> sample_string
'py'
>>> sample_string = sample_string + sample_characters[2]
>>> sample_string
'pyt'
>>> sample_string = sample_string + sample_characters[three]
>>> sample_string
'pyth'
>>> sample_string = sample_string + sample_characters[four]
>>> sample_string
'pytho'
>>> sample_string = sample_string + sample_characters[5]
>>> sample_string
'python'
>>>
Here, the variable sample_string can be like a state of this system that’s getting modified after executing the sequence of instructions, and it may be simply extracted to trace the progress of this system. The similar may be executed utilizing a for loop (additionally thought-about crucial programming) in a shorter model of the above code:
>>> sample_characters = ['p','y','t','h','o','n']
>>> sample_string = ''
>>> sample_string
>>> for c in sample_characters:
... sample_string = sample_string + c
... print(sample_string)
...
p
py
pyt
pyth
pytho
python
>>>
Functional programming paradigm
The functional programming paradigm treats program computation because the analysis of mathematical features primarily based on lambda calculus. Lambda calculus is a proper system in mathematical logic for expressing computation primarily based on perform abstraction and utility utilizing variable binding and substitution. It follows the “what-to-solve” strategy—that’s, it expresses logic with out describing its management movement—therefore it is usually labeled because the declarative programming mannequin.
The practical programming paradigm promotes stateless features, however it’s vital to notice that Python’s implementation of practical programming deviates from normal implementation. Python is claimed to be an impure practical language as a result of it’s attainable to keep up state and create unintended effects in case you are not cautious. That mentioned, practical programming is helpful for parallel processing and is super-efficient for duties requiring recursion and concurrent execution.
>>> sample_characters = ['p','y','t','h','o','n']
>>> import functools
>>> sample_string = functools.scale back(lambda s,c: s + c, sample_characters)
>>> sample_string
'python'
>>>
Using the identical instance, the practical approach of concatenating a listing of characters to kind a string could be the identical as above. Since the computation occurs in a single line, there isn’t any specific approach to acquire the state of this system with sample_string and monitor the progress. The practical programming implementation of this instance is fascinating, because it reduces the strains of code and easily does its job in a single line, except for utilizing the functools module and the scale back technique. The three key phrases—functools, scale back, and lambda—are outlined as follows:
- functools is a module for higher-order features and gives for features that act on or return different features. It encourages writing reusable code, as it’s simpler to duplicate present features with some arguments already handed and create a brand new model of a perform in a well-documented method.
- scale back is a technique that applies a perform of two arguments cumulatively to the gadgets in sequence, from left to proper, to scale back the sequence to a single worth. For instance:
>>> sample_list = [1,2,three,four,5]
>>> import functools
>>> sum = functools.scale back(lambda x,y: x + y, sample_list)
>>> sum
15
>>> ((((1+2)+three)+four)+5)
15
>>> - lambda features are small, anonymized (i.e., anonymous) features that may take any variety of arguments however spit out just one worth. They are helpful when they’re used as an argument for one more perform or reside inside one other perform; therefore they’re meant for use just for one occasion at a time.
Procedural programming paradigm
The procedural programming paradigm is a subtype of crucial programming by which statements are structured into procedures (also referred to as subroutines or features). The program composition is extra of a process name the place the packages would possibly reside someplace within the universe and the execution is sequential, thus changing into a bottleneck for useful resource utilization. Like the crucial programming paradigm, procedural programming follows the stateful mannequin. The procedural programming paradigm facilitates the apply of excellent program design and permits modules to be reused within the type of code libraries.
This modularized type of improvement is a really previous improvement model. The totally different modules in a program can haven’t any relationship with one another and may be positioned in several areas, however having a mess of modules creates hardships for a lot of builders, because it not solely results in duplication of logic but additionally lots of overhead by way of discovering and making the suitable calls. Note that within the following implementation, the strategy stringify could possibly be outlined anyplace within the universe and, to do its trick, solely require the right name with the specified arguments.
>>> def stringify(characters):
... string = ''
... for c in characters:
... string = string + c
... return stringify
...
>>> sample_characters = ['p','y','t','h','o','n']
>>> stringify(sample_characters)
'python'
>>>
Object-oriented programming paradigm
The object-oriented programming paradigm considers primary entities as objects whose occasion can comprise each knowledge and the corresponding strategies to switch that knowledge. The totally different ideas of object-oriented design assist code reusability, knowledge hiding, and so forth., however it’s a complicated beast, and writing the identical logic an in object-oriented technique is hard. For instance:
>>> class StringOps:
... def __init__(self, characters):
... self.characters = characters
... def stringify(self):
... self.string = ''.be a part of(self.characters)
...
>>> sample_characters = ['p','y','t','h','o','n']
>>> sample_string = StringOps(sample_characters)
>>> sample_string.stringify()
>>> sample_string.string
'python'
>>>
What programming paradigm ought to I select?
It’s vital to notice that there isn’t any comparability between the various kinds of programming paradigms. Since software program is nothing however data illustration, the reply to the query: “What is the best way to represent my problem?” is selecting a selected programming paradigm.
In layman’s phrases, in case your drawback entails a sequence of easy sequential manipulations, following the old-school crucial programming paradigm could be the least costly by way of effort and time and provide the greatest outcomes. In the case of issues requiring mathematical transformations of values, filtering info, mapping, and reductions, practical programming with program computation as mathematical features would possibly come in useful. If the issue is structured as a bunch of interrelated objects with sure attributes that may change with the passage of time, relying on sure situations, object-oriented programming might be super-useful. Of course, a rule-based strategy wouldn’t work right here, as programming paradigm alternative can be closely depending on the kind of knowledge to be processed, the dynamic wants of the system, and numerous different issues like scalability.
Recent tendencies
Analyzing the most recent tech buzzwords can assist establish why sure programming paradigms work higher than others.
- Machine studying makes use of a wholesome mixture of crucial programming and practical programming with a splash of immutability. Feature extraction and preprocessing are greatest approached functionally, as they require mathematical processing of information as a result of mappings, reductions, and filtrations can just about be executed in parallel with out a lot dependence on every others’ knowledge factors. Training of machine studying fashions is greatest approached through old-school crucial programming, as optimizing features’ worth (a.okay.a. the state of this system) must be up to date at every iteration and subsequently requires sequential execution at many factors within the algorithm. It is faster than practical programming in that case. It additionally avoids creating copies of every part after every step; as an alternative it simply updates the previous-value placeholders.
- Deep studying may be carried out effectively in a practical method, as deep studying fashions are compositional. The whole course of optimizes a set of composite features, weights are immutable and stateless, and updates may be utilized in any order so long as corresponding inputs are computed. Using practical programming gives concurrency and parallelism without charge and likewise makes it simpler to work with massive, distributed fashions. There are additionally sure customized paradigms the place practical programming is intertwined with informational concept to keep away from overfitting within the statistical fashions.
- Data manipulation may be approached with both practical or object-oriented programming. In the practical programming approach, every part is immutable, algorithms are expressed succinctly, and there’s native sample matching, however formulation of the mathematical expression-like command is an artwork. Approaching it in an object-oriented programming approach gives for recursive and iterative loops and a class-based construction that makes it simpler to scale for larger knowledge and new features. The draw back is that the algorithms and the code logic will not be expressed in a readable approach. Although each paradigms are inclined to have an computerized garbage-collection system and might entry and manipulate databases easily, the selection of which one to decide on is closely depending on the programmer’s data.
Takeaway
There is a excessive chance that any two builders would disagree on the perfect coding model for any state of affairs and have legitimate arguments to assist their opinion. The superb factor about Python is that it enables you to select the programming paradigm that works the perfect for you in a given state of affairs.
As the above examples exhibit, a process can all the time be damaged into sub-tasks the place every smaller half is coded in a very totally different paradigm. The mix-and-match model works completely so long as the packages used are minimal, the inputs and outputs are clearly outlined, and the complexity is moderated. There are not any guidelines that say you may’t mix types as wanted. Python doesn’t cease in the course of deciphering your utility and show a mode error if you combine types.
Because there isn’t any good guidebook for selecting an accurate coding model for a given use case, the perfect suggestion is to strive a number of paradigms weighing of their professionals and cons till you discover the one which results in a easy but environment friendly answer. There might be occasions throughout this experimentation when you will notice that as an alternative of utilizing a single model all through, a mixture of programming paradigms works higher for various components to an answer. During this course of, it is usually extremely beneficial to doc the necessities and the trials of various types to share with the neighborhood and get suggestions. The feedback and options will assist with improvement in addition to your teammates and any future builders which are added to the staff.
Jigyasa Grover offered Taming styles of Python programming at All Things Open, October 13-15 in Raleigh, N.C.
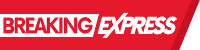