Bash is a strong programming language, one completely designed to be used on the command line and in shell scripts. This three-part collection, primarily based on my three-volume Linux self-study course, explores utilizing Bash as a programming language on the command-line interface (CLI).
The first article on this collection explored some easy command-line programming with Bash, together with utilizing variables and management operators. The second article seemed into the kinds of file, string, numeric, and miscellaneous logical operators that present execution-flow management logic and various kinds of shell expansions in Bash. This third (and last) article examines the usage of loops for performing numerous kinds of iterative operations and methods to manage these loops.
Loops
Every programming language I’ve ever used has not less than a pair kinds of loop constructions that present numerous capabilities to carry out repetitive operations. I exploit the for loop very often however I additionally discover the whereas and till loops helpful.
for loops
Bash’s implementation of the for command is, for my part, a bit extra versatile than most as a result of it will probably deal with non-numeric values; in distinction, for instance, the usual C language for loop can deal solely with numeric values.
The primary construction of the Bash model of the for command is straightforward:
for Var in list1 ; do list2 ; accomplished
This interprets to: “For every worth in list1, set the $Var to that worth after which carry out this system statements in list2 utilizing that worth; when the entire values in list1 have been used, it’s completed, so exit the loop.” The values in list1 is usually a easy, specific string of values, or they are often the results of a command substitution (described within the second article within the collection). I exploit this assemble often.
To strive it, be sure that ~/testdir remains to be the current working listing (PWD). Clean up the listing, then take a look at a trivial instance of the for loop beginning with an specific checklist of values. This checklist is a mixture of alphanumeric values—however don’t forget that every one variables are strings and could be handled as such.
[scholar@studentvm1 testdir]$ rm *
[scholar@studentvm1 testdir]$ for I in a b c d 1 2 Three four ; do echo $I ; accomplished
a
b
c
d
1
2
Three
four
Here is a little more helpful model with a extra significant variable title:
[scholar@studentvm1 testdir]$ for Dept in "Human Resources" Sales Finance "Information Technology" Engineering Administration Research ; do echo "Department $Dept" ; accomplished
Department Human Resources
Department Sales
Department Finance
Department Information Technology
Department Engineering
Department Administration
Department Research
Make some directories (and present some progress data whereas doing so):
[scholar@studentvm1 testdir]$ for Dept in "Human Resources" Sales Finance "Information Technology" Engineering Administration Research ; do echo "Working on Department $Dept" ; mkdir "$Dept" ; accomplished
Working on Department Human Resources
Working on Department Sales
Working on Department Finance
Working on Department Information Technology
Working on Department Engineering
Working on Department Administration
Working on Department Research
[scholar@studentvm1 testdir]$ ll
complete 28
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 Administration
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 Engineering
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 Finance
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 'Human Resources'
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 'Information Technology'
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 Research
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:45 Sales
The $Dept variable should be enclosed in quotes within the mkdir assertion; in any other case, two-part division names (equivalent to “Information Technology”) shall be handled as two separate departments. That highlights a greatest observe I wish to comply with: all file and listing names ought to be a single phrase. Although most fashionable working programs can cope with areas in names, it takes additional work for sysadmins to make sure that these particular circumstances are thought of in scripts and CLI packages. (They virtually definitely ought to be thought of, even when they’re annoying since you by no means know what recordsdata you’ll have.)
So, delete every part in ~/testdir—once more—and do that yet another time:
[scholar@studentvm1 testdir]$ rm -rf * ; ll
complete Zero
[scholar@studentvm1 testdir]$ for Dept in Human-Resources Sales Finance Information-Technology Engineering Administration Research ; do echo "Working on Department $Dept" ; mkdir "$Dept" ; accomplished
Working on Department Human-Resources
Working on Department Sales
Working on Department Finance
Working on Department Information-Technology
Working on Department Engineering
Working on Department Administration
Working on Department Research
[scholar@studentvm1 testdir]$ ll
complete 28
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Administration
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Engineering
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Finance
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Human-Resources
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Information-Technology
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Research
drwxrwxr-x 2 scholar scholar 4096 Apr eight 15:52 Sales
Suppose somebody asks for an inventory of all RPMs on a specific Linux pc and a brief description of every. This occurred to me once I labored for the State of North Carolina. Since open supply was not “approved” to be used by state businesses at the moment, and I solely used Linux on my desktop pc, the pointy-haired bosses (PHBs) wanted an inventory of every piece of software program that was put in on my pc in order that they may “approve” an exception.
How would you strategy that? Here is a technique, beginning with the data that the rpm –qa command supplies an entire description of an RPM, together with the 2 objects the PHBs need: the software program title and a short abstract.
Build as much as the ultimate outcome one step at a time. First, checklist all RPMs:
[scholar@studentvm1 testdir]$ rpm -qa
perl-HTTP-Message-6.18-Three.fc29.noarch
perl-IO-1.39-427.fc29.x86_64
perl-Math-Complex-1.59-429.fc29.noarch
lua-5.Three.5-2.fc29.x86_64
java-11-openjdk-headless-11.Zero.ea.28-2.fc29.x86_64
util-linux-2.32.1-1.fc29.x86_64
libreport-fedora-2.9.7-1.fc29.x86_64
rpcbind-1.2.5-Zero.fc29.x86_64
libsss_sudo-2.Zero.Zero-5.fc29.x86_64
libfontenc-1.1.Three-9.fc29.x86_64
<snip>
Add the kind and uniq instructions to kind the checklist and print the distinctive ones (because it’s potential that some RPMs with equivalent names are put in):
[scholar@studentvm1 testdir]$ rpm -qa | kind | uniq
a2ps-four.14-39.fc29.x86_64
aajohan-comfortaa-fonts-Three.001-Three.fc29.noarch
abattis-cantarell-fonts-Zero.111-1.fc29.noarch
abiword-Three.Zero.2-13.fc29.x86_64
abrt-2.11.Zero-1.fc29.x86_64
abrt-addon-ccpp-2.11.Zero-1.fc29.x86_64
abrt-addon-coredump-helper-2.11.Zero-1.fc29.x86_64
abrt-addon-kerneloops-2.11.Zero-1.fc29.x86_64
abrt-addon-pstoreoops-2.11.Zero-1.fc29.x86_64
abrt-addon-vmcore-2.11.Zero-1.fc29.x86_64
<snip>
Since this offers the right checklist of RPMs you need to take a look at, you need to use this because the enter checklist to a loop that may print all the main points of every RPM:
[scholar@studentvm1 testdir]$ for RPM in `rpm -qa | kind | uniq` ; do rpm -qi $RPM ; accomplished
This code produces far more knowledge than you need. Note that the loop is full. The subsequent step is to extract solely the data the PHBs requested. So, add an egrep command, which is used to pick ^Name or ^Summary. The carat (^) specifies the start of the road; thus, any line with Name or Summary firstly of the road is displayed.
[scholar@studentvm1 testdir]$ for RPM in `rpm -qa | kind | uniq` ; do rpm -qi $RPM ; accomplished | egrep -i "^Name|^Summary"
Name : a2ps
Summary : Converts textual content and different kinds of recordsdata to PostScript
Name : aajohan-comfortaa-fonts
Summary : Modern model true kind font
Name : abattis-cantarell-fonts
Summary : Humanist sans serif font
Name : abiword
Summary : Word processing program
Name : abrt
Summary : Automatic bug detection and reporting device
<snip>
You can strive grep as an alternative of egrep within the command above, however it is not going to work. You may additionally pipe the output of this command by way of the much less filter to discover the outcomes. The last command sequence appears like this:
[scholar@studentvm1 testdir]$ for RPM in `rpm -qa | kind | uniq` ; do rpm -qi $RPM ; accomplished | egrep -i "^Name|^Summary" > RPM-summary.txt
This command-line program makes use of pipelines, redirection, and a for loop—all on a single line. It redirects the output of your little CLI program to a file that can be utilized in an electronic mail or as enter for different functions.
This means of build up this system one step at a time permits you to see the outcomes of every step and be sure that it’s working as you anticipate and supplies the specified outcomes.
From this train, the PHBs obtained an inventory of over 1,900 separate RPM packages. I severely doubt that anybody learn that checklist. But I gave them precisely what they requested for, and I by no means heard one other phrase from them about it.
Other loops
There are two extra kinds of loop constructions accessible in Bash: the whereas and till constructions, that are similar to one another in each syntax and performance. The primary syntax of those loop constructions is straightforward:
whereas [ expression ] ; do checklist ; accomplished
and
till [ expression ] ; do checklist ; accomplished
The logic of the primary reads: “While the expression evaluates as true, execute the list of program statements. When the expression evaluates as false, exit from the loop.” And the second: “Until the expression evaluates as true, execute the list of program statements. When the expression evaluates as true, exit from the loop.”
While loop
The whereas loop is used to execute a collection of program statements whereas (as long as) the logical expression evaluates as true. Your PWD ought to nonetheless be ~/testdir.
The easiest type of the whereas loop is one which runs perpetually. The following kind makes use of the true assertion to all the time generate a “true” return code. You may additionally use a easy “1”—and that might work simply the identical—however this illustrates the usage of the true assertion:
[scholar@studentvm1 testdir]$ X=Zero ; whereas [ true ] ; do echo $X ; X=$((X+1)) ; accomplished | head
Zero
1
2
Three
four
5
6
7
eight
9
[scholar@studentvm1 testdir]$
This CLI program ought to make extra sense now that you’ve got studied its elements. First, it units $X to zero in case it has a price left over from a earlier program or CLI command. Then, for the reason that logical expression [ true ] all the time evaluates to 1, which is true, the checklist of program directions between do and accomplished is executed perpetually—or till you press Ctrl+C or in any other case ship a sign 2 to this system. Those directions are an arithmetic growth that prints the present worth of $X after which increments it by one.
One of the tenets of The Linux Philosophy for Sysadmins is to try for magnificence, and one solution to obtain magnificence is simplicity. You can simplify this program by utilizing the variable increment operator, ++. In the primary occasion, the present worth of the variable is printed, after which the variable is incremented. This is indicated by putting the ++ operator after the variable:
[scholar@studentvm1 ~]$ X=Zero ; whereas [ true ] ; do echo $((X++)) ; accomplished | head
Zero
1
2
Three
four
5
6
7
eight
9
Now delete | head from the top of this system and run it once more.
In this model, the variable is incremented earlier than its worth is printed. This is specified by putting the ++ operator earlier than the variable. Can you see the distinction?
[scholar@studentvm1 ~]$ X=Zero ; whereas [ true ] ; do echo $((++X)) ; accomplished | head
1
2
Three
four
5
6
7
eight
9
You have lowered two statements right into a single one which prints the worth of the variable and increments that worth. There can also be a decrement operator, —.
You want a way for stopping the loop at a particular quantity. To accomplish that, change the true expression to an precise numeric analysis expression. Have this system loop to five and cease. In the instance code beneath, you possibly can see that -le is the logical numeric operator for “less than or equal to.” This means: “So lengthy as $X is lower than or equal to five, the loop will proceed. When $X increments to six, the loop terminates.”
[scholar@studentvm1 ~]$ X=Zero ; whereas [ $X -le 5 ] ; do echo $((X++)) ; accomplished
Zero
1
2
Three
four
5
[scholar@studentvm1 ~]$
Until loop
The till command could be very very like the whereas command. The distinction is that it’s going to proceed to loop till the logical expression evaluates to “true.” Look on the easiest type of this assemble:
[scholar@studentvm1 ~]$ X=Zero ; till false ; do echo $((X++)) ; accomplished | head
Zero
1
2
Three
four
5
6
7
eight
9
[scholar@studentvm1 ~]$
It makes use of a logical comparability to depend to a particular worth:
[scholar@studentvm1 ~]$ X=Zero ; till [ $X -eq 5 ] ; do echo $((X++)) ; accomplished
Zero
1
2
Three
four
[scholar@studentvm1 ~]$ X=Zero ; till [ $X -eq 5 ] ; do echo $((++X)) ; accomplished
1
2
Three
four
5
[scholar@studentvm1 ~]$
Summary
This collection has explored many highly effective instruments for constructing Bash command-line packages and shell scripts. But it has barely scratched the floor on the numerous fascinating issues you are able to do with Bash; the remaining is as much as you.
I’ve found that one of the simplest ways to study Bash programming is to do it. Find a easy undertaking that requires a number of Bash instructions and make a CLI program out of them. Sysadmins do many duties that lend themselves to CLI programming, so I’m positive that you’ll simply discover duties to automate.
Many years in the past, regardless of being aware of different shell languages and Perl, I made the choice to make use of Bash for all of my sysadmin automation duties. I’ve found that—typically with a little bit of looking out—I’ve been in a position to make use of Bash to perform every part I would like.
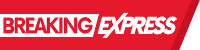