Python has earned a popularity as an exquisite newbie programming language. But the place does one start?
One of my favourite methods to get individuals all in favour of programming is by writing video games.
PursuedPyBear (ppb) is a sport programming library optimized for instructing, and I not too long ago used it to show my kids extra about my favorite programming language.
The Jupyter undertaking is a browser-based Python console, initially designed for knowledge scientists to play with knowledge.
I’ve a Jupyter Notebook designed to show you the right way to make a easy interactive sport, which you’ll be able to obtain from here. In order to open the file, you have to to put in the most recent Jupyter undertaking, JupyterLab.
Prerequisites:
- Running a current model of Python (directions for Linux, Mac, and Windows)
- Running a current model of Git (directions here)
We will briefly configure a digital surroundings to create a separate house for the wanted libraries. (You can study extra about how digital environments work here.)
$ git clone https://github.com/moshez/penguin-bit-by-bit.git
$ cd penguin-bit-by-bit
$ python -m venv venv
$ supply ./venv/bin/activate
$ pip set up -r necessities.txt
$ jupyter lab .
The final command ought to open JupyterLab in your default browser on the tackle http://localhost:8888/lab. Choose the dynamic_penguin.ipynb file within the left-hand column, and we will get began!
The occasion loop that can run the sport
Jupyter runs an occasion loop internally, which is a course of that manages the working of additional asynchronous operations. The occasion loop utilized in Jupyter is asyncio, and PursuedPyBear runs its personal occasion loop.
We can combine the 2 utilizing one other library, Twisted, like glue. This sounds sophisticated, however fortunately, the complexity is hidden behind libraries, which is able to do all of the exhausting work for us.
The following cell in Jupyter takes care of the primary half—integrating Twisted with the asyncio occasion loop.
The__file__ = None
is required to combine PursuedPyBear with Jupyter.
from twisted.web import asyncioreactor
asyncioreactor.set up()
__file__ = None
Next, we’d like a “setup” operate. A setup operate is a standard time period for the configuration of key sport parts. However, our operate will solely put the sport “scene” in a worldwide variable. Think of it like us defining the desk on which we’ll play our sport.
The following cell in Jupyter Notebook will do the trick.
def setup(scene):
world SCENE
SCENE = scene
Now we have to combine PursuedPyBear’s occasion loop with Twisted. We use the txppb
module for that:
import txppb
d = txppb.run(setup)
d.addBoth(print)
The print
on the finish helps us if the sport crashes due to a bug—it can print out a traceback to the Jupyter output.
This will present an empty window, prepared for the sport parts.
This is the place we begin benefiting from Jupyter—historically, the entire sport must be written earlier than we begin enjoying. We buck conference, nonetheless, and begin enjoying the sport instantly!
Making the sport fascinating with interplay
It shouldn’t be a really fascinating sport, although. It has nothing and simply sits there. If we wish one thing, we higher add it.
In online game programming, the issues shifting on the display are known as “sprites.” In PursuedPyBear, sprites are represented by courses. A sprite will robotically use a picture named the identical as the category. I acquired a little bit penguin picture from Kenney, a set of free and open supply online game belongings.
import ppbclass Penguin(ppb.Sprite):
cross
Now let’s put the penguin riiiiiight within the center.
SCENE.add(Penguin(pos=(zero,zero)))
It fastidiously sits there within the center. This is marginally extra fascinating than having nothing. That’s good—that is precisely what we wish. In incremental sport growth, each step must be solely marginally extra fascinating.
Adding motion to our penguin sport with ppb
But penguins usually are not meant to sit down nonetheless! The penguin ought to transfer round. We could have the participant management the penguin with the arrow keys. First, let’s map the keys to vectors:
from ppb import keycodesDIRECTIONS =
Now we’ll use a utility library. The set_in_class
operate units the tactic within the class. Python’s means so as to add features to courses retroactively is basically coming in useful!
from mzutil import set_in_classPenguin.route = ppb.Vector(zero, zero)
@set_in_class(Penguin)
def on_update(self, update_event, sign):
self.place += update_event.time_delta * self.route
The code for set_in_class
shouldn’t be lengthy, but it surely does use some non-trivial Python tips. We will put the total utility library on the finish of the article for evaluate, and for the sake of stream, we’ll skip it for now.
Back to the penguin!
Oh, um, properly.
The penguin is diligently shifting…at zero pace, exactly nowhere. Let’s manually set the route to see what occurs.
Penguin.route = DIRECTIONS[keycodes.Up]/four
The route is up, however a little bit gradual. This provides sufficient time to set the penguin’s route again to zero manually. Let’s try this now!
Penguin.route = ppb.Vector(zero, zero)
Adding interactivity to our penguin sport
Phew, that was thrilling—however not what we needed. We need the penguin to reply to keypresses. Controlling it from the code is what avid gamers consult with as “cheating.”
Let’s set it to set the route to the keypress, and again to zero when the secret is launched.
@set_in_class(Penguin)
def on_key_pressed(self, key_event, sign):
self.route = DIRECTIONS.get(key_event.key, ppb.Vector(zero, zero))@set_in_class(Penguin)
def on_key_released(self, key_event, sign):
if key_event.key in DIRECTIONS:
self.route = ppb.Vector(zero, zero)
The Penguin is a bit bored, is not it? Maybe we must always give it an orange ball to play with.
class OrangeBall(ppb.Sprite):
cross
Again, I made positive to have a picture known as orangeball.png
. Now let’s put the ball on the left facet of the display.
SCENE.add(OrangeBall(pos=(-four, zero)))
Try as it would, the penguin can not kick the ball. Let’s have the ball transfer away from the penguin when it approaches.
First, let’s outline what it means to “kick” the ball. Kicking the ball means deciding the place it will be in a single second, after which setting its state to “moving.”
At first, we’ll simply transfer it by having the primary replace transfer it to the goal place.
OrangeBall.is_moving = False@set_in_class(OrangeBall)
def kick(self, route):
self.target_position = self.place + route
self.original_position = self.place
self.time_passed = zero
self.is_moving = True@set_in_class(OrangeBall)
def on_update(self, update_event, sign):
if self.is_moving:
self.place = self.target_position
self.is_moving = False
Now, let’s kick it!
ball, = SCENE.get(variety=OrangeBall)
ball.kick(ppb.Vector(1, 1))
But this simply teleports the ball; it instantly adjustments the place. In actual life, the ball goes between the intermediate factors. When it is shifting, it can interpolate between the place it’s and the place it must go.
Naively, we’d use linear interpolation. But a cool online game trick is to make use of an “easing” operate. Here, we use the widespread “smooth step.”
from mzutil import smooth_step@set_in_class(OrangeBall)
def maybe_move(self, update_event, sign):
if not self.is_moving:
return False
self.time_passed += update_event.time_delta
if self.time_passed >= 1:
self.place = self.target_position
self.is_moving = False
return False
t = smooth_step(self.time_passed)
self.place = (1-t) * self.original_position + t * self.target_position
return TrueOrangeBall.on_update = OrangeBall.maybe_move
Now, let’s strive kicking it once more.
ball, = SCENE.get(variety=OrangeBall)
ball.kick(ppb.Vector(1, -1))
But actually, the penguin must be kicking the ball. When the ball sees that it’s colliding with the penguin, it can kick itself in the other way. If the penguin has gotten proper on prime of it, the ball will select a random route.
The replace operate now calls maybe_move
and can solely test collision if we’re not shifting proper now.
from mzutil import collide
import randomOrangeBall.x_offset = OrangeBall.y_offset = zero.25
@set_in_class(OrangeBall)
def on_update(self, update_event,sign):
if self.maybe_move(update_event, sign):
return
penguin, = update_event.scene.get(variety=Penguin)
if not collide(penguin, self):
return
strive:
route = (self.place - penguin.place).normalize()
besides ZeroDivisionError:
route = ppb.Vector(random.uniform(-1, 1), random.uniform(-1, 1)).normalize()
self.kick(route)
But simply kicking a ball round shouldn’t be that a lot enjoyable. Let’s add a goal.
class Target(ppb.Sprite):
cross
Let’s put the goal on the proper of the display.
SCENE.add(Target(pos=(four, zero)))
Rewarding our penguin
Now, we’ll need a reward for the penguin when it kicks the ball into the goal. How a couple of fish?
class Fish(ppb.Sprite):
cross
When the goal will get the ball, it ought to take away it and create a brand new ball on the different finish of the display. Then, it can trigger a fish to seem.
@set_in_class(Target)
def on_update(self, update_event, sign):
for ball in update_event.scene.get(variety=OrangeBall):
if not collide(ball, self):
proceed
update_event.scene.take away(ball)
update_event.scene.add(OrangeBall(pos=(-four, random.uniform(-three, three))))
update_event.scene.add(Fish(pos=(random.uniform(-four, -three),
random.uniform(-three, three))))
We need to have the penguin eat the fish. When the fish sees the penguin, it ought to vanish.
Fish.x_offset = zero.05
Fish.y_offset = zero.2
@set_in_class(Fish)
def on_update(self, update_event,sign):
penguin, = update_event.scene.get(variety=Penguin)
if collide(penguin, self):
update_event.scene.take away(self)
It works!
Iterative sport design is enjoyable for penguins and other people alike!
This has all of the makings of a sport: the player-controlled penguin kicks the ball into the goal, will get a fish, eats the fish, and kicks a brand new ball. This would work as a “grinding level” a part of a sport, or we may add obstacles to make the penguin’s life tougher.
Whether you might be an skilled programmer, or simply getting began, programming video video games is enjoyable. PursuedPyBear with Jupyter brings all the enjoyment of basic 2D video games with the interactive programming capabilities of the basic environments like Logo and Smalltalk. Time to get pleasure from a little bit retro 80s!
Appendix
Here is the total supply code of our utility library. It offers some fascinating ideas to make the sport board work. For extra on the way it does that, examine collision detection, setattr. and the __name__ attribute.
def set_in_class(klass):
def retval(func):
setattr(klass, func.__name__, func)
return func
return retvaldef smooth_step(t):
return t * t * (three - 2 * t)_WHICH_OFFSET = dict(
prime='y_offset',
backside='y_offset',
left='x_offset',
proper='x_offset'
)_WHICH_SIGN = dict(prime=1, backside=-1, left=-1, proper=1)
def _effective_side(sprite, route):
return (getattr(sprite, route) -
_WHICH_SIGN[route] *
getattr(sprite, _WHICH_OFFSET[route], zero))def _extreme_side(sprite1, sprite2, route):
signal = -_WHICH_SIGN[route]
return signal * max(signal * _effective_side(sprite1, route),
signal * _effective_side(sprite2, route))
def collide(sprite1, sprite2):
return (_extreme_side(sprite1, sprite2, 'backside') <
_extreme_side(sprite1, sprite2, 'prime')
and
_extreme_side(sprite1, sprite2, 'left') <
_extreme_side(sprite1, sprite2, 'proper'))
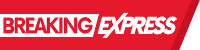