When I construct infrastructure, I do it as code. The motion towards infrastructure as code implies that each change is seen, whether or not it is by way of configuration administration recordsdata or full-blown GitOps.
Terraform is a software for constructing, upgrading, and sustaining your infrastructure as code. As its GitHub page explains:
“Terraform enables you to safely and predictably create, change, and improve infrastructure. It is an open source tool that codifies APIs into declarative configuration files that can be shared amongst team members, treated as code, edited, reviewed, and versioned.”
The better part is you’ll be able to import your current infrastructure right into a Terraform configuration state, and all future adjustments might be tracked. That monitoring gives a whole understanding of your manufacturing atmosphere (and some other environments), which will be backed as much as a neighborhood or distant Git repository to version-control your complete infrastructure.
In this text, I’ll clarify the best way to use Terraform to handle state in a Minikube Kubernetes cluster.
Prerequisites
Terraform is cloud-agnostic, so you’ll be able to run about any kind of Kubernetes cluster in any cloud through the use of the related suppliers. A provider is the core of Terraform’s plugin structure, and every supplier is “responsible for understanding API interactions and exposing resources” in order that the primary Terraform mission can stay lean, however the mission can broaden to any system.
In this instance, I will use Terraform 11 on a Linux desktop. To observe alongside, additionally, you will want Helm 2.16.7, Minikube, and kubectl:
Before operating a construct, discover out what the command-line utility gives. Run terraform
, and the output will present the execution plan and apply instructions. At the underside, there’s an improve guidelines that may come in useful if you’re on an older model of Terraform. The builders have inbuilt an amazing command to examine compatibility between variations.
Getting began
To construct one thing in Terraform, you might want to create modules, that are folders with a set of configuration recordsdata that may collect info and execute the motion you might want to full. Terraform recordsdata at all times finish within the file extension .tf
. Start with one most important.tf
file, comparable to:
jess@Athena:~/terraform_doc$ contact most important.tf
You additionally must know some primary Terraform instructions and necessities earlier than you can begin making something throughout the cluster:
terraform init
: Initializes a Terraform working listing
– It should be throughout the identical listing because the.tf
recordsdata or nothing will occur.terraform validate
: Confirms the Terraform file’s syntax is appropriate
– Always run this to substantiate the code is constructed appropriately and won’t have errors.terraform plan
: Generates and exhibits what is going to change if you runterraform apply
– Run this earlier thanapply
to substantiate the outcomes are as you plan.terraform apply
: Builds or adjustments infrastructure
– It will present the execution plan and requires a sure or no to execute until you utilize the--auto-approve
flag, which can make it execute mechanically.Terraform refresh
: Updates the native state file towards actual assets
– This ensures Terraform has an correct view of what’s within the present atmosphere.terraform destroy
: Deletes and removes Terraform-managed infrastructure
– This will completely take away something created and saved within the state file from the cluster.
For the configuration on this instance, all the things managed by Terraform is held in a neighborhood state file. According to Terraform’s docs:
“This state is saved by default in a neighborhood file named
terraform.tfstate
, nevertheless it can be saved remotely, which works higher in a staff atmosphere. Terraform makes use of this native state to create plans and make adjustments to your infrastructure. Prior to any operation, Terraform does a refresh to replace the state with the true infrastructure.”
Now that you’ve got this background info, you’ll be able to transfer on and edit your most important.tf
file, examine your cluster, and work in direction of including configurations with Terraform.
Prep and construct Minikube
Before getting began with Terraform, you should create a Minikube cluster. This instance makes use of Minikube model v1.9.2. Run minikube begin
:
jess@Athena:~/terraform_doc$ minikube begin
? minikube 1.11.zero is on the market! Download it: https://github.com/kubernetes/minikube/releases/tag/v1.11.0
? To disable this discover, run: 'minikube config set WantUpdateNotification false'? minikube v1.9.2 on Ubuntu 18.04
✨ Using the kvm2 driver based mostly on current profile
? Starting management aircraft node m01 in cluster minikube
? Restarting current kvm2 VM for "minikube" ...
? Preparing Kubernetes v1.18.zero on Docker 19.03.eight ...
? Enabling addons: default-storageclass, storage-provisioner
? Done! kubectl is now configured to make use of "minikube"
Check your new cluster and add a namespace
Check your new Minikube cluster along with your trusty kubectl
instructions:
jess@Athena:~/terraform_doc$ kubectl get nodes
NAME STATUS ROLES AGE VERSION
minikube Ready grasp 4m5s v1.18.zero
The cluster is up and operating, so add configurations to your most important.tf
file. First, you may want a provider, which “is responsible for understanding API interactions and exposing resources.” The supplier on this instance might be (aptly) named Kubernetes. Edit your most important.tf
file and add the supplier:
supplier "kubernetes"
This syntax tells Terraform that the cluster is operating in Minikube.
Now you’ll need a definition of a useful resource block. A useful resource block describes a number of infrastructure objects, comparable to digital networks, compute cases, or higher-level elements comparable to DNS data.
Add one Kubernetes namespace to the cluster:
useful resource "kubernetes_namespace" "1-minikube-namespace"
metadata
title = "my-first-terraform-namespace"
Next, run the terraform init
command to examine your supplier model and initialize Terraform:
jess@Athena:~/terraform_doc$ terraform initInitializing supplier plugins...
The following suppliers wouldn't have any model constraints in configuration,
so the newest model was put in.To forestall computerized upgrades to new main variations that will include breaking
adjustments, it is suggested so as to add model = "..." constraints to the
corresponding supplier blocks in configuration, with the constraint strings
advised beneath.
* supplier.kubernetes: model = "~> 1.11"Terraform has been efficiently initialized!
You might now start working with Terraform. Try operating "terraform plan" to see
any adjustments which might be required in your infrastructure. All Terraform instructions
ought to now work.If you ever set or change modules or backend configuration for Terraform,
rerun this command to reinitialize your working listing. If you overlook, different
instructions will detect it and remind you to take action if mandatory.
Run your plan to see what might be executed:
jess@Athena:~/terraform_doc$ terraform plan
Refreshing Terraform state in-memory previous to plan...
The refreshed state might be used to calculate this plan, however is not going to be
continued to native or distant state storage.------------------------------------------------------------------------
An execution plan has been generated and is proven beneath.
Resource actions are indicated with the next symbols:
+ create
Terraform will carry out the next actions:
+ kubernetes_namespace.1-minikube-namespace
id: <computed>
metadata.#: "1"
metadata.zero.technology: <computed>
metadata.zero.title: "my-first-terraform-namespace"
metadata.zero.resource_version: <computed>
metadata.zero.self_link: <computed>
metadata.zero.uid: <computed>
Plan: 1 so as to add, zero to alter, zero to destroy.------------------------------------------------------------------------
Note: You did not specify an "-out" parameter to save lots of this plan, so Terraform
cannot assure that precisely these actions might be carried out if
"terraform apply" is subsequently run.
Now that you already know what Terraform will do, apply your configuration:
jess@Athena:~/terraform_doc$ terraform applyAn execution plan has been generated and is proven beneath.
Resource actions are indicated with the next symbols:
+ create
Terraform will carry out the next actions:
+ kubernetes_namespace.1-minikube-namespace
id: <computed>
metadata.#: "1"
metadata.zero.technology: <computed>
metadata.zero.title: "my-first-terraform-namespace"
metadata.zero.resource_version: <computed>
metadata.zero.self_link: <computed>
metadata.zero.uid: <computed>
Plan: 1 so as to add, zero to alter, zero to destroy.Do you wish to carry out these actions?
Terraform will carry out the actions described above.
Only 'sure' might be accepted to approve.
Enter a price: sure-----------------------------------
kubernetes_namespace.1-minikube-namespace: Creating...
metadata.#: "" => "1"
metadata.zero.technology: "" => "<computed>"
metadata.zero.title: "" => "my-first-terraform-namespace"
metadata.zero.resource_version: "" => "<computed>"
metadata.zero.self_link: "" => "<computed>"
metadata.zero.uid: "" => "<computed>"
kubernetes_namespace.1-minikube-namespace: Creation full after 0s (ID: my-first-terraform-namespace)
Apply full! Resources: 1 added, zero modified, zero destroyed.
Finally, verify that your new namespace exists by operating kubectl get ns
:
jess@Athena:~/terraform_doc$ kubectl get ns
NAME STATUS AGE
default Active 28d
kube-node-lease Active 28d
kube-public Active 28d
kube-system Active 28d
my-first-terraform-namespace Active 2m19s
Run it by way of a Helm chart
The potential to manually write a Terraform configuration file, run it, and see leads to Kubernetes is good. What’s even nicer? Being capable of rerun the identical instructions by way of a Helm chart.
Run the helm create <title>
command to generate a chart:
$ helm create buildachart
Creating buildachart
You want one other supplier block for this train. There is a particular Helm supplier, and it requires a Kubernetes cluster title in order that Helm is aware of the place to put in its chart. Add the brand new supplier, which is proven beneath, to your current most important.tf
file:
supplier "helm"
Now that Helm is configured, you might want to add a Helm chart for this terraform module to put in. To preserve issues easy, preserve the Helm chart in the identical folder you are utilizing in your Terraform state:
jess@Athena:~/terraform_doc$ ls
buildachart most important.tf terraform.tfstate
Add the brand new Helm useful resource in order that the chart will be put in and tracked by way of your Terraform state through the use of the helm_release
useful resource. I named this useful resource native
and imported my chart title and my chart location:
useful resource "helm_release" "local"
Now that you’ve got added these items, run Terraform’s initialization command once more. It will replace the state based mostly in your adjustments, together with downloading a brand new supplier:
jess@Athena:~/terraform_doc$ terraform initInitializing supplier plugins...
- Checking for accessible supplier plugins on https://releases.hashicorp.com...
- Downloading plugin for supplier "helm" (1.2.2)...The following suppliers wouldn't have any model constraints in configuration,
so the newest model was put in.To forestall computerized upgrades to new main variations that will include breaking
adjustments, it is suggested so as to add model = "..." constraints to the
corresponding supplier blocks in configuration, with the constraint strings
advised beneath.
* supplier.helm: model = "~> 1.2"
* supplier.kubernetes: model = "~> 1.11"Terraform has been efficiently initialized!
You might now start working with Terraform. Try operating "terraform plan" to see
any adjustments which might be required in your infrastructure. All Terraform instructions
ought to now work.If you ever set or change modules or backend configuration for Terraform,
rerun this command to reinitialize your working listing. If you overlook, different
instructions will detect it and remind you to take action if mandatory.
Then plan your new configurations:
jess@Athena:~/terraform_doc$ terraform plan
Refreshing Terraform state in-reminiscence previous to plan...
The refreshed state might be used to calculate this plan, however is not going to be
continued to native or distant state storage.kubernetes_namespace.1-minikube-namespace: Refreshing state... (ID: my-first-terraform-namespace)
------------------------------------------------------------------------
An execution plan has been generated and is proven beneath.
Resource actions are indicated with the next symbols:
+ createTerraform will carry out the next actions:
+ helm_release.native
id: <computed>
atomic: "false"
chart: "./buildachart"
cleanup_on_fail: "false"
create_namespace: "false"
dependency_update: "false"
disable_crd_hooks: "false"
disable_openapi_validation: "false"
disable_webhooks: "false"
force_update: "false"
lint: "false"
max_history: "0"
metadata.#: <computed>
title: "buildachart"
namespace: "default"
recreate_pods: "false"
render_subchart_notes: "true"
substitute: "false"
reset_values: "false"
reuse_values: "false"
skip_crds: "false"
standing: "deployed"
timeout: "300"
confirm: "false"
model: "0.1.0"
wait: "true"Plan: 1 so as to add, zero to alter, zero to destroy.
------------------------------------------------------------------------
Note: You didn't specify an "-out" parameter to save lots of this plan, so Terraform
can't assure that precisely these actions might be carried out if
"terraform apply" is subsequently run.
Apply your configuration, solely this time add the --auto-approve
flag so it can execute with out affirmation:
jess@Athena:~/terraform_doc$ terraform apply --auto-approve
kubernetes_namespace.1-minikube-namespace: Refreshing state... (ID: my-first-terraform-namespace)
helm_release.native: Creating...
atomic: "" => "false"
chart: "" => "./buildachart"
cleanup_on_fail: "" => "false"
create_namespace: "" => "false"
dependency_update: "" => "false"
disable_crd_hooks: "" => "false"
disable_openapi_validation: "" => "false"
disable_webhooks: "" => "false"
force_update: "" => "false"
lint: "" => "false"
max_history: "" => "0"
metadata.#: "" => "<computed>"
title: "" => "buildachart"
namespace: "" => "default"
recreate_pods: "" => "false"
render_subchart_notes: "" => "true"
substitute: "" => "false"
reset_values: "" => "false"
reuse_values: "" => "false"
skip_crds: "" => "false"
standing: "" => "deployed"
timeout: "" => "300"
confirm: "" => "false"
model: "" => "0.1.0"
wait: "" => "true"
helm_release.native: Creation full after 8s (ID: buildachart)
Apply full! Resources: 1 added, zero modified, zero destroyed.
Although it says the chart deployed, it is at all times good to double-check and make sure that the brand new Helm chart is in place. Check to see in case your pod made it through the use of a kubectl
command:
jess@Athena:~/terraform_doc$ kubectl get pods
NAME READY STATUS RESTARTS AGE
buildachart-68c86ccf5f-lchc5 1/1 Running zero 43s
This confirms your pod is operating, which suggests your chart deployed! You even have a brand new backup state file:
jess@Athena:~/terraform_doc$ ls
buildachart most important.tf terraform.tfstate terraform.tfstate.backup
Terraform is protecting of state, which is a superb characteristic. It mechanically generates a previous-state file after every replace. This permits model management of your infrastructure, and you’ll at all times save the present and most up-to-date state. Since this can be a native construct, persist with the present and former states with out model management.
Rollback a change and import one thing
As you run Terraform instructions, the backup state file is generated and up to date, which suggests you’ll be able to roll again earlier adjustments precisely as soon as until you’re holding state recordsdata in storage some place else (e.g., a database) with different configurations to handle the recordsdata.
In this instance, you might want to roll again your Helm chart deployment. Why? Well, as a result of you’ll be able to.
Before you do something, take a minute to run the terraform refresh
command to see if there’s something completely different between the cluster and the present state:
jess@Athena:~/terraform_doc$ terraform refresh
helm_release.native: Refreshing state... (ID: buildachart)
kubernetes_namespace.1-minikube-namespace: Refreshing state... (ID: my-first-terraform-namespace)
There is a bizarre workaround to roll again adjustments: You can overwrite your state file with the backup file, or you’ll be able to remark the code adjustments you rolled out by way of Terraform recordsdata and permit Terraform to destroy them.
In this instance, I will comment-out code and rerun Terraform, so the Helm chart might be deleted. Comments start with //
in Terraform recordsdata:
jess@Athena:~/terraform_doc$ cat most important.tf
supplier "kubernetes"useful resource "kubernetes_namespace" "1-minikube-namespace"
metadata
title = "my-first-terraform-namespace"
//supplier "helm"
// kubernetes
//
//useful resource "helm_release" "local"
// title = "buildachart"
// chart = "./buildachart"
//
After you remark all the things out, run terraform apply
:
jess@Athena:~/terraform_doc$ terraform apply
helm_release.native: Refreshing state... (ID: buildachart)
null_resource.minikube: Refreshing state... (ID: 4797320155365789412)
kubernetes_namespace.1-minikube-namespace: Refreshing state... (ID: my-terraform-namespace)An execution plan has been generated and is proven beneath.
Resource actions are indicated with the next symbols:
- destroy
Terraform will carry out the next actions:
- helm_release.native
Plan: zero so as to add, zero to alter, 1 to destroy.Do you wish to carry out these actions?
Terraform will carry out the actions described above.
Only 'sure' might be accepted to approve.
Enter a price: sure
helm_release.native: Destroying... (ID: buildachart)
helm_release.native: Destruction full after 0s
Apply full! Resources: zero added, zero modified, 1 destroyed.
To see what overwriting the file appears like, reapply the Helm chart and overwrite the state file. Here is a snippet of the chart being recreated (this textual content output will be fairly lengthy):
helm_release.native: Still creating... (10s elapsed)
helm_release.native: Creation full after 15s (ID: buildachart)
Apply full! Resources: 1 added, zero modified, zero destroyed.Here’s the file overwrite and the plan displaying that the helm chart must be rerun.
jess@Athena:~/terraform_doc$ cp terraform.tfstate.backup terraform.tfstate
jess@Athena:~/terraform_doc$ terraform plan
Refreshing Terraform state in-memory previous to plan...
The refreshed state might be used to calculate this plan, however is not going to be
continued to native or distant state storage.
null_resource.minikube: Refreshing state... (ID: 4797320155365789412)
kubernetes_namespace.1-minikube-namespace: Refreshing state... (ID: my-terraform-namespace)------------------------------------------------------------------------
An execution plan has been generated and is proven beneath.
Resource actions are indicated with the next symbols:
+ create
Terraform will carry out the next actions:
+ helm_release.native
id: <computed>
atomic: "false"
chart: "./buildachart"
cleanup_on_fail: "false"
create_namespace: "false"
dependency_update: "false"
disable_crd_hooks: "false"
disable_openapi_validation: "false"
disable_webhooks: "false"
force_update: "false"
max_history: "0"
metadata.#: <computed>
title: "buildachart"
namespace: "default"
recreate_pods: "false"
render_subchart_notes: "true"
substitute: "false"
reset_values: "false"
reuse_values: "false"
skip_crds: "false"
standing: "deployed"
timeout: "300"
confirm: "false"
model: "0.1.0"
wait: "true"
Plan: 1 so as to add, zero to alter, zero to destroy.------------------------------------------------------------------------
Note: You did not specify an "-out" parameter to save lots of this plan, so Terraform
cannot assure that precisely these actions might be carried out if
"terraform apply" is subsequently run.
Be conscious that you’ll run into an issue if you don’t clear up the atmosphere when overwriting your state recordsdata. That drawback exhibits up within the title utilization:
Do you wish to carry out these actions?
Terraform will carry out the actions described above.
Only 'sure' might be accepted to approve.
Enter a price: sure
helm_release.native: Creating...
atomic: "" => "false"
chart: "" => "./buildachart"
cleanup_on_fail: "" => "false"
create_namespace: "" => "false"
dependency_update: "" => "false"
disable_crd_hooks: "" => "false"
disable_openapi_validation: "" => "false"
disable_webhooks: "" => "false"
force_update: "" => "false"
max_history: "" => "0"
metadata.#: "" => "<computed>"
title: "" => "buildachart"
namespace: "" => "default"
recreate_pods: "" => "false"
render_subchart_notes: "" => "true"
substitute: "" => "false"
reset_values: "" => "false"
reuse_values: "" => "false"
skip_crds: "" => "false"
standing: "" => "deployed"
timeout: "" => "300"
confirm: "" => "false"
model: "" => "0.1.0"
wait: "" => "true"
Error: Error making use of plan:
1 error occurred:
* helm_release.native: 1 error occurred:
* helm_release.native: can not re-use a reputation that's nonetheless in useTerraform doesn't mechanically rollback within the face of errors.
Instead, your Terraform state file has been partially up to date with
any assets that efficiently accomplished. Please tackle the error
above and apply once more to incrementally change your infrastructure.
This creates a reuse subject because of commenting out and bringing again a useful resource. To repair this, do a state import, which lets you take one thing that’s already out within the atmosphere and let Terraform begin to observe it once more.
For this, you want the namespace and chart title you wish to import together with the module title you’re importing. The module on this case is helm.native
, and it’s generated out of your useful resource code within the useful resource that begins with "helm_release" "local"
.
For the reimport, you’ll need the namespace the place the useful resource is at present deployed, so the import will seem like default/buildachart
. This format is required for something involving a namespace:
jess@Athena:~/terraform_doc$ terraform import helm_release.native default/buildachart
helm_release.native: Importing from ID "default/buildachart"...
helm_release.native: Import full!
Imported helm_release (ID: buildachart)
helm_release.native: Refreshing state... (ID: buildachart)Import profitable!
The assets that had been imported are proven above. These assets at the moment are in
your Terraform state and can henceforth be managed by Terraform.
This strategy of reimporting will be tough, nevertheless it’s essential for state administration.
Clean up
What’s so good about Terraform is which you can rapidly clear up after your self if you’re testing. The observe
command is one other nice strategy to destroy your day if you happen to aren’t cautious about the place you run it:
jess@Athena:~/terraform_doc$ terraform destroy
helm_release.native: Refreshing state... (ID: buildachart)
kubernetes_namespace.1-minikube-namespace: Refreshing state... (ID: my-first-terraform-namespace)An execution plan has been generated and is proven beneath.
Resource actions are indicated with the next symbols:
- destroy
Terraform will carry out the next actions:
- helm_release.native
- kubernetes_namespace.1-minikube-namespace
Plan: zero so as to add, zero to alter, 2 to destroy.Do you actually wish to destroy all assets?
Terraform will destroy all your managed infrastructure, as proven above.
There is no undo. Only 'sure' might be accepted to substantiate.
Enter a price: sure
helm_release.native: Destroying... (ID: buildachart)
kubernetes_namespace.1-minikube-namespace: Destroying... (ID: my-first-terraform-namespace)
helm_release.native: Destruction full after 1s
kubernetes_namespace.1-minikube-namespace: Destruction full after 7s
Destroy full! Resources: 2 destroyed.
One run of terraform destroy
eliminated your pods and namespace, however your cluster stays. You are again to sq. one:
jess@Athena:~/terraform_doc$ kubectl get pods
No assets present in default namespace.jess@Athena:~/terraform_doc$ kubectl get ns
NAME STATUS AGE
default Active 28d
kube-node-lease Active 28d
kube-public Active 28d
kube-system Active 28djess@Athena:~/terraform_doc$ minikube standing
m01
host: Running
kubelet: Running
apiserver: Running
kubeconfig: Configured
Final notes
To say you can also make something with Terraform is an understatement. It’s a software that may handle each facet of atmosphere creation and destruction. It has a robust and easy idea of state administration that may permit groups to remain in sync with the group’s supposed infrastructure.
However, if you’re not cautious, this software will be fairly unforgiving. If you’re transferring state recordsdata and never paying consideration, you’ll be able to trigger a little bit of a difficulty with what Terraform believes it must handle. When you’re utilizing this software, watch out to not overextend your self or write an excessive amount of without delay, as you’ll be able to code your self right into a nook if you happen to aren’t paying consideration.
Terraform is greatest when it is used for infrastructure provisioning. It minimizes what issues occur when managing state, and permits instruments designed for configuration and deployment to enhance its options.
What have you ever achieved with Terraform and Kubernetes? Share your expertise within the feedback beneath.
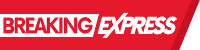