In this text sequence, Opensource.com Correspondents and others have been writing the identical “guess the number” sport in numerous programming languages. This train reveals how the fundamental ideas you will discover in most programming languages—variables, expressions, and statements—will be utilized to study new languages.
Most languages have a “way of doing things” that is supported by their design, and people methods will be fairly completely different from one program to a different. These methods embrace modularity (grouping associated performance collectively), declarative vs. crucial, object-orientation, low- vs. high-level syntactic options, and so forth.
In the “guess the number” program, the pc picks a quantity between one and 100 and asks you to guess the quantity. The program loops till you guess the fitting reply.
In this text, I will present you tips on how to write this utility in Algol 68. I will additionally attempt to train the next ideas you will discover in any programming language:
- Variables
- Input
- Output
- Conditional analysis
- Loops
Guess the quantity in Algol 68
This instance makes use of the Algol 68 Genie compiler, obtainable in lots of Linux distros, created by Marcel Van Der Veer.
OK, I can hear a few of you on the market moaning, “oh no, why Algol 68? That language is so old and has been irrelevant since before there were implementations.” And I respect your opinions, I actually do. Really. But I consider many cool issues exist in right this moment’s programming languages that come from the onerous considering finished by the designers of Algol 68, which justifies studying a bit in regards to the language.
Algol 68 is statically typed and never notably verbose. Algol 68 Genie compiles and executes in a single go, so Algol 68 can really feel a bit like a scripting language. The Genie implementation presents many helpful hooks into our favourite working system, which retains it from feeling horribly dated and means a good bit of helpful work will be finished with it.
Some factors value mentioning on the outset:
- One of Algol 68’s design ideas is letting something that might moderately be considered an expression (a assemble that delivers a price) be authorized code.
- Algol 68 “reserved words” are sometimes expressed as boldface symbols in this system supply, which is sort of onerous to do with textual content information, so most compilers have a manner of indicating token is a reserved phrase. In Algol 68 Genie, the default is to make use of all higher case letters; for instance,
BEGIN
,IF
,THEN
, and many others. - Speaking of issues like
BEGIN … END
,IF … THEN … ELSE
, Algol 68 has a “closed syntax.” ABEGIN
will need to have a correspondingEND
, just likeIF
will need to have aFI
, and aDO
will need to have anOD
. - Algol 68 usually treats whitespace as irrelevant—even inside numbers or variable names. Therefore,
myvariablename
andmy variable identify
(and evenmyvar iablename
) all seek advice from the identical variable. - Algol 68 requires “go on symbols”—semicolons—between (however not following) statements in a sequence to be evaluated statement-by-statement.
- Algol 68 incorporates a “string” sort however doesn’t present a wealthy set of string processing primitives, which could be a bit irritating.
- Algol 68 is crucial reasonably than declarative and never object-oriented.
With that preamble, right here is my “guess the number” implementation (with line numbers to make it simpler to evaluation a few of the particular options):
1 on logical file finish (stand in,
2 (REF FILE f) BOOL: (print(("Goodbye!",new line));cease));
three first random(42);
four INT random quantity = ENTIER (subsequent random * 100.zero) + 1;
5 print(("the secret number is",random quantity,new line));
6 print("guess a number: ");
7 WHILE
eight INT guess = learn int;
9 IF guess < random quantity THEN
10 print("too low, try again: ");
11 TRUE
12 ELIF guess > random quantity THEN
13 print("too high, try again: ");
14 TRUE
15 ELSE
16 print(("that's right",new line));
17 FALSE
18 FI
19 DO SKIP OD
Breaking it down
Jumping proper in: traces 1 and a couple of outline what occurs when an finish of file is detected on the enter coming from the console.
This state of affairs is managed by calling the process on logical file finish
and passing two arguments: a file to be monitored and a process to be referred to as when the top of file is detected. The file you need to monitor is the usual enter, stand in
. Line 2 is the definition of the process; that is an expression that yields a process. It has one parameter, which is a pointer to a file named “f” that’s written as REF FILE f
. It returns a boolean worth, indicated by BOOL
.
The textual content after the :
is the process physique, which:
- Starts with a quick start image,
(
- Is adopted by a name to the
print
process with a listing of two arguments, the string"Goodbye!"
and thenew line
process that may emit a newline within the output stream - Is adopted by a “go on symbol”—the semicolon
- Is adopted by a name to the process
cease
- Is adopted by the transient finish image,
)
- Is adopted by the parenthesis closing the record of arguments to the “on logical file end “name
- Is adopted by the “go on symbol,”
;
More verbosely, I may have written this process definition as:
(REF FILE f) BOOL: BEGIN
print(("Goodbye!",new line));
cease
END
It’s most likely value mentioning that Algol 68 makes a really sturdy distinction between values and references to values. An Algol 68 worth is conceptually just like a relentless or immutable or ultimate worth seen in lots of right this moment’s common programming languages. A worth can’t be modified. A reference to a price, then again, basically defines a location the place a price will be saved and the contents of that location will be modified. This corresponds to a variable, or mutable, or non-final worth.
By manner of concrete examples:
INT forty two = 42
defines an “integer value” named forty two
, which evaluates to the quantity 42
.
And:
INT fink := 42
defines an integral variable fink
and makes use of :=
to assign the worth 42
to it. This expression is definitely shorthand for:
REF INT fink = LOC INT;
fink := 42
This makes the correspondence between values (INT forty two
) and variables (REF INT fink
) clearer on the expense of some verbosity. The LOC INT
factor is a “local generator”—house for an integer worth is allotted on the stack. There can be a “heap generator” helpful for constructing buildings that persist throughout process calls.
Phew! Back to the code.
Lines three and four initialize the system random quantity generator by calling the “setup” process first random() with an integer “seed” argument (which kinda hasta be 42, proper?), then calling the process subsequent random—which takes no arguments and due to this fact would not require parentheses with nothing between—and multiplies that by 100 to provide a consequence between zero.zero and 99.9999…, truncating the consequence created utilizing the unary operator ENTIER to provide a consequence between zero and 99, and at last including 1 to provide a consequence between 1 and 100.
Worth mentioning at this level is that Algol 68 appears to be the primary language to assist the definition of unary and binary operators, that are distinct from procedures. You should put parentheses across the expression:
subsequent random * 100.zero
as a result of in any other case ENTIER
would bind to the following random, giving the quantity zero, reasonably than to the entire expression.
On line 5 is a name to the print
process, which is used right here as a debugging factor. Notice the nested parentheses; print
takes an argument that’s both a printable worth or expression or a listing of printable values or expressions. When it is a record, you utilize a “denotation,” which opens and closes with parentheses.
Line 6 makes use of print
once more to supply the one string guess a quantity:
.
Lines 7 by 20 are a WHILE … DO … OD
loop. A couple of attention-grabbing issues right here: First, all of the work is completed as part of the logical expression evaluated by the WHILE
, so the physique of the loop incorporates solely the reserved phrase SKIP
, which implies “do nothing.”
Lines eight by 17 are a sequence of two statements: the definition of the integer worth guess
, which is obtained by calling the process learn int
to get an integer from the enter, adopted by the IF … THEN … ELIF … ELSE … FI
assertion. Note that every of the THEN
, ELIF
, and ELSE
elements finish with a boolean worth TRUE
or FALSE
. This causes the entire IF… FI
assertion to return both TRUE
or FALSE
, which, being the final assertion within the sequence of statements, is the worth “delivered” to WHILE
to find out whether or not to loop round once more or not.
A extra typical language may need a construction just like:
boolean doAgain = true;
whereas (doAgain)
if much less then
doAgain = true
else if extra then
doAgain = true
else
doAgain = false
Because Algol 68 is expression-oriented, you need not declare that variable doAgain
; you simply incorporate the values to be generated into the expression being evaluated by the WHILE
half.
What’s cool about that is you are able to do issues just like the ternary operator in C—besides way more broadly and higher—with the usual IF...FI
:
do once more := IF guess < random quantity THEN print("something"); TRUE ELIF guess > random quantity THEN print("something else"); TRUE ELSE print("another thing"); FALSE FI
Note that I additionally took care to not declare something as a mutable worth when pointless. Since the worth guess
solely has the scope of the WHILE
loop, it simply defines a brand new worth every time.
One nagging little drawback I did not deal with stems from the usage of learn int
; if the annoyed person varieties in a price that’s not convertible to an integer, this system will cease with an error situation. You may handle this drawback by calling the process on worth error
, which is analogous to the on logical file finish
process. I will go away that so that you can determine. You did not suppose you’d get away from this with out an train, did you?
What we discovered
In the introduction, I listed the programming ideas this train ought to discover. How did I do?
- Variables: This reveals that Algol 68 thinks of variables as named places and helps named (immutable) values.
- Input: It used
stand in
as predefined console enter and dealt with end-of-file circumstances. - Output: It used
print
to print messages on the console. - Conditional analysis: It used Algol 68
if-then-else-fi
andif
statements as expressions. - Loops: It used Algol 68’s
whereas
loop, together with utilizing a sequence of statements to calculate the worth to be examined.
It additionally used some Algol 68 customary library (which Algol 68 calls “standard prelude”) performance, together with the random quantity generator and I/O exception testing.
Run this system:
$ a68g guess.a68
the key quantity is +26
guess a quantity: 50
too excessive, strive once more: 25
too low, strive once more: 37
too excessive, strive once more: 31
too excessive, strive once more: 28
too excessive, strive once more: 26
that's proper
$
One factor I did not cowl is feedback. In Algol 68 Genie, feedback can start and finish with the image COMMENT
, or CO
, or #
, as in:
# this can be a remark #
If you are keen on exploring Algol 68, check out Marcel’s site or the various contributed options in Algol 68 on Rosetta Code.
In closing, again to the “dead languages” factor. Yes, it is a bit of an esoteric pursuit. But studying obscure languages is a good way to understand how far we have come (or, in some circumstances, not) and to provide a extra rounded perspective on language options we take without any consideration.
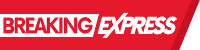