There are loads of textual content editors accessible. There are people who run within the terminal, in a GUI, in a browser, and in a browser engine. Many are superb, and a few are nice. But generally, essentially the most satisfying reply to any query is the one you construct your self.
Make no mistake: constructing a very good textual content editor is loads tougher than it might appear. But then once more, it’s additionally not as onerous as you would possibly concern to construct a primary one. In reality, most programming toolkits have already got many of the textual content editor components prepared so that you can use. The parts across the textual content enhancing, similar to a menu bar, file chooser dialogues, and so forth, are straightforward to drop into place. As a consequence, a primary textual content editor is a surprisingly enjoyable and elucidating, although intermediate, lesson in programming. You would possibly end up keen to make use of a device of your personal building, and the extra you employ it, the extra you is likely to be impressed so as to add to it, studying much more in regards to the programming language you’re utilizing.
To make this train practical, it’s greatest to decide on a language with a very good GUI toolkit. There are many to select from, together with Qt, FLTK, or GTK, however make sure you evaluate the documentation first to make sure it has the options you count on. For this text, I take advantage of Java with its built-in Swing widget set. If you wish to use a unique language or a unique toolset, this text can nonetheless be helpful in supplying you with an concept of method the issue.
Writing a textual content editor in any main toolkit is surprisingly related, regardless of which one you select. If you’re new to Java and wish additional data on getting began, learn my Guessing Game article first.
Project setup
Normally, I take advantage of and suggest an IDE like Netbeans or Eclipse, however I discover that, when training a brand new language, it may be useful to do some handbook labor, so that you higher perceive the issues that get hidden from you when utilizing an IDE. In this text, I assume you’re programming utilizing a textual content editor and a terminal.
Before getting began, create a venture listing for your self. In the venture folder, create one listing known as src
to carry your supply recordsdata.
$ mkdir -p myTextEditor/src
$ cd myTextEditor
Create an empty file known as TextEdit.java
in your src
listing:
$ contact src/TextEditor.java
Open the file in your favourite textual content editor (I imply your favourite one that you simply didn’t write) and prepare to code!
Package and imports
To guarantee your Java software has a singular identifier, you will need to declare a package deal title. The typical format for that is to make use of a reverse area title, which is especially straightforward do you have to even have a site title. If you don’t, you should utilize native
as the highest stage. As common for Java and plenty of languages, the road is terminated with a semicolon.
After naming your Java package deal, you will need to inform the Java compiler (javac
) what libraries to make use of when constructing your code. In follow, that is one thing you often add to as you code since you hardly ever know your self what libraries you want. However, there are some which might be apparent beforehand. For occasion, you recognize this textual content editor relies across the Swing GUI toolkit, so importing javax.swing.JFrame
and javax.swing.UIManager
and different associated libraries is a given.
package deal com.instance.textedit;import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JTextSpace;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.filechooser.FileSystemView;
import java.awt.Component;
import java.awt.occasion.ActionEvent;
import java.awt.occasion.ActionListener;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileAuthor;
import java.io.IOException;
import java.util.Scanner;
import java.util.logging.Level;
import java.util.logging.Logger;
For the aim of this train, you get prescient information of all of the libraries you want upfront. In actual life, no matter what language you like, you’ll uncover libraries as you analysis resolve any given drawback, and then you definately’ll import it into your code and use it. And don’t fear—do you have to overlook to incorporate a library, your compiler or interpreter will warn you!
Main window
This is a single-window software, so the first class of this software is a JFrame with an ActionListener
hooked up to catch menu occasions. In Java, once you’re utilizing an current widget component, you “extend” it along with your code. This predominant window wants three fields: the window itself (an occasion of JFrame), an indicator for the return worth of the file chooser, and the textual content editor itself (JTextSpace).
Amazingly, these few traces do about 80% of the work towards implementing a primary textual content editor as a result of JTextSpace is Java’s textual content entry discipline. Most of the remaining 80 traces deal with helper options, like saving and opening recordsdata.
Building a menu
The JMenuBar
widget is designed to sit down on the prime of a JFrame, offering as many entries as you need. Java isn’t a drag-and-drop programming language, although, so for each menu you add, you will need to additionally program a operate. To maintain this venture manageable, I present 4 features: creating a brand new file, opening an current file, saving textual content to a file, and shutting the appliance.
The course of of making a menu is mainly the identical in hottest toolkits. First, you create the menubar itself, then you definately create a top-level menu (similar to “File”), and then you definately create submenu gadgets (similar to “New,” “Save,” and so forth).
All that’s left to do now’s to implement the features described by the menu gadgets.
Programming menu actions
Your software responds to menu choices as a result of your JFrame has an ActionListener
hooked up to it. When you implement an occasion handler in Java, you will need to “override” its built-in features. This sounds extra extreme than it truly is. You’re not rewriting Java; you’re simply implementing features which were outlined however not applied by the occasion handler.
In this case, you will need to override the actionPerformed
methodology. Because practically all entries within the File menu have one thing to do with recordsdata, my code defines a JFileChooser early. The remainder of the code is separated into clauses of an if
assertion, which seems to be to see what occasion was acquired and acts accordingly. Each clause is drastically completely different from the opposite as a result of every merchandise suggests one thing wholly distinctive. The most related are Open and Save as a result of they each use the JFileChooser to pick out a degree within the filesystem to both get or put information.
The “New” choice clears the JTextSpace with out warning, and Quit closes the appliance with out warning. Both of those “features” are harmful, so do you have to wish to make a small enchancment to this code, that’s a very good place to start out. A pleasant warning that the content material hasn’t been saved is an important function of any good textual content editor, however for simplicity’s sake, that’s a function for the long run.
@Override
public void actionPerformed(ActionEvent e) {
String ingest = null;
JFileChooser jfc = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
jfc.setDialogTitle("Choose destination.");
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES);String ae = e.getActionCommand();
if (ae.equals("Open"))
returnValue = jfc.presentOpenDialog(null);
if (returnValue == JFileChooser.APPROVE_OPTION)
File f = new File(jfc.getSelectedFile().getAbsolutePath());
strive
catch ( FileNotFoundException ex) ex.printStackTrace();
// SAVE
else if (ae.equals("Save"))
returnValue = jfc.presentSaveDialog(null);
strive
File f = new File(jfc.getSelectedFile().getAbsolutePath());
FileWriter out = new FileWriter(f);
out.write(space.getText());
out.shut();
catch (FileNotFoundException ex) catch (IOException ex)
Component f = null;
JOptionPane.showMessageDialog(f,"Error.");
else if (ae.equals("New"))
space.setText("");
else if (ae.equals("Quit"))
}
}
That’s technically all there’s to this textual content editor. Of course, nothing’s ever really performed, and apart from, there’s nonetheless the testing and packaging steps, so there’s nonetheless loads of time to find lacking requisites. In case you’re not choosing up on the trace: there’s positively one thing lacking on this code. Do you recognize what it’s but? (It’s talked about primarily within the Guessing Game article.)
Testing
You can now take a look at your software. Launch your textual content editor from a terminal:
$ java ./src/TextEdit.java
error: can’t discover predominant(String[]) methodology in class: com.instance.textedit.TextEdit
It appears that the code hasn’t obtained a predominant methodology. There are a number of methods to repair this drawback: you can create a predominant methodology in TextEdit.java
and have it run an occasion of the TextEdit
class, or you possibly can create a separate file containing the primary methodology. Both work equally properly, however the latter is extra practical when it comes to what to anticipate from massive tasks, so it’s price getting used to coping with separate recordsdata that work collectively to make an entire software.
Create a Main.java
file in src
and open in your favourite editor:
You can strive once more, however now there are two recordsdata that rely on each other to run, so it’s a must to compile the code. Java makes use of the javac
compiler, and you may set your vacation spot listing with the -d
choice:
$ javac src/*java -d .
This creates a brand new listing construction modeled precisely after your package deal title: com/instance/textedit
. This new classpath accommodates the recordsdata Main.class
and TextEdit.class
, that are the 2 recordsdata that make up your software. You can run them with java
by referencing the situation and title (not the filename) of your Main class:
$ java information/slackermedia/textedit/Main
Your textual content editor opens, and you may kind into it, open recordsdata, and even save your work.
Sharing your work as a Java package deal
While it appears to be acceptable to some programmers to ship functions as an assortment of supply recordsdata and hearty encouragement to learn to run them, Java makes it very easy to package deal up your software so others can run it. You have many of the construction required, however you do want so as to add some metadata to a Manifest.txt
file:
$ echo "Manifest-Version: 1.0" > Manifest.txt
The jar
command, used for packaging, is loads just like the tar command, so most of the choices could look acquainted to you. To create a JAR file:
$ jar cvfme TextEdit.jar
Manifest.txt
com.instance.textedit.Main
com/instance/textedit/*.class
From the syntax of the command, it’s possible you’ll surmise that it creates a brand new JAR file known as TextEdit.jar
, with its required manifest information situated in Manifest.txt
. Its predominant class is outlined as an extension of the package deal title, and the category itself is com/instance/textedit/Main.class
.
You can view the contents of the JAR file:
$ jar tvf TextEdit.jar
zero Wed Nov 25 META-INF/
105 Wed Nov 25 META-INF/MANIFEST.MF
338 Wed Nov 25 com/instance/textedit/textedit/Main.class
4373 Wed Nov 25 com/instance/textedit/textedit/TextEdit.class
And you possibly can even extract it with the xvf
choices, if you happen to’d prefer to see how your metadata has been built-in into the MANIFEST.MF
file.
Run your JAR file with the java
command:
$ java -jar TextEdit.jar
You may even create a desktop file, so your software launches on the click on of an icon in your functions menu.
Improve it
In its present state, it is a very primary textual content editor, greatest suited to fast notes or brief README paperwork. Some enhancements (similar to including a vertical scrollbar) are fast and straightforward with a little analysis, whereas others (similar to implementing an in depth preferences system) require actual work.
But if you happen to’ve been that means to be taught a brand new language, this may very well be the right sensible venture on your self-education. Creating a textual content editor, as you possibly can see, isn’t overwhelming when it comes to code, and it’s manageable in scope. If you employ textual content editors regularly, then writing your personal will be satisfying and enjoyable. So open your favourite textual content editor (the one you wrote) and begin including options!
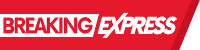