Naïve Bayes is a classification method that serves as the premise for implementing a number of classifier modeling algorithms. Naïve Bayes-based classifiers are thought of a number of the easiest, quickest, and easiest-to-use machine studying methods, but are nonetheless efficient for real-world functions.
Naïve Bayes relies on Bayes’ theorem, formulated by 18th-century statistician Thomas Bayes. This theorem assesses the likelihood that an occasion will happen primarily based on situations associated to the occasion. For instance, a person with Parkinson’s disease usually has voice variations; therefore such signs are thought of associated to the prediction of a Parkinson’s prognosis. The unique Bayes’ theorem supplies a way to find out the likelihood of a goal occasion, and the Naïve variant extends and simplifies this technique.
Solving a real-world downside
This article demonstrates a Naïve Bayes classifier’s capabilities to unravel a real-world downside (as opposed to a whole business-grade utility). I am going to assume you may have fundamental familiarity with machine studying (ML), so a number of the steps that aren’t primarily associated to ML prediction, comparable to knowledge shuffling and splitting, usually are not lined right here. If you might be an ML newbie or want a refresher, see An introduction to machine learning today and Getting started with open source machine learning.
The Naïve Bayes classifier is supervised, generative, non-linear, parametric, and probabilistic.
In this text, I am going to reveal utilizing Naïve Bayes with the instance of predicting a Parkinson’s prognosis. The dataset for this instance comes from this UCI Machine Learning Repository. This knowledge contains a number of speech sign variations to evaluate the chance of the medical situation; this instance will use the primary eight of them:
- MDVP:Fo(Hz): Average vocal basic frequency
- MDVP:Fhi(Hz): Maximum vocal basic frequency
- MDVP:Flo(Hz): Minimum vocal basic frequency
- MDVP:Jitter(%), MDVP:Jitter(Abs), MDVP:RAP, MDVP:PPQ, and Jitter:DDP: Five measures of variation in basic frequency
The dataset used on this instance, shuffled and break up to be used, is obtainable in my GitHub repository.
ML with Python
I am going to use Python to implement the answer. The software program I used for this utility is:
- Python three.eight.2
- Pandas 1.1.1
- scikit-learn zero.22.2.post1
There are a number of open supply Naïve Bayes classifier implementations accessible in Python, together with:
- NLTK Naïve Bayes: Based on the usual Naïve Bayes algorithm for textual content classification
- NLTK Positive Naïve Bayes: A variant of NLTK Naïve Bayes that performs binary classification with partially labeled coaching units
- Scikit-learn Gaussian Naïve Bayes: Provides partial match to assist an information stream or very massive dataset
- Scikit-learn Multinomial Naïve Bayes: Optimized for discrete knowledge options, instance counts, or frequency
- Scikit-learn Bernoulli Naïve Bayes: Designed for binary/Boolean options
I’ll use sklearn Gaussian Naive Bayes for this instance.
Here is my Python implementation of naive_bayes_parkinsons.py
:
import pandas as pd# Feature columns we use
x_rows=['MDVP:Fo(Hz)','MDVP:Fhi(Hz)','MDVP:Flo(Hz)',
'MDVP:Jitter(%)','MDVP:Jitter(Abs)','MDVP:RAP','MDVP:PPQ','Jitter:DDP']
y_rows=['standing']# Train
# Read prepare knowledge
train_data = pd.read_csv('parkinsons/Data_Parkinsons_TRAIN.csv')
train_x = train_data[x_rows]
train_y = train_data[y_rows]
print("train_x:n", train_x)
print("train_y:n", train_y)# Load sklearn Gaussian Naive Bayes and match
from sklearn.naive_bayes import GaussianNBgnb = GaussianNB()
gnb.match(train_x, train_y)# Prediction on prepare knowledge
predict_train = gnb.predict(train_x)
print('Prediction on prepare knowledge:', predict_train)# Accuray rating on prepare knowledge
from sklearn.metrics import accuracy_score
accuracy_train = accuracy_score(train_y, predict_train)
print('Accuray rating on prepare knowledge:', accuracy_train)# Test
# Read check knowledge
test_data = pd.read_csv('parkinsons/Data_Parkinsons_TEST.csv')
test_x = test_data[x_rows]
test_y = test_data[y_rows]# Prediction on check knowledge
predict_test = gnb.predict(test_x)
print('Prediction on check knowledge:', predict_test)# Accuracy Score on check knowledge
accuracy_test = accuracy_score(test_y, predict_test)
print('Accuray rating on check knowledge:', accuracy_train)
Run the Python utility:
$ python naive_bayes_parkinsons.pytrain_x:
MDVP:Fo(Hz) MDVP:Fhi(Hz) ... MDVP:RAP MDVP:PPQ Jitter:DDP
zero 152.125 161.469 ... zero.00191 zero.00226 zero.00574
1 120.080 139.710 ... zero.00180 zero.00220 zero.00540
2 122.400 148.650 ... zero.00465 zero.00696 zero.01394
three 237.323 243.709 ... zero.00173 zero.00159 zero.00519
.. ... ... ... ... ... ...
155 138.190 203.522 ... zero.00406 zero.00398 zero.01218[156 rows x eight columns]
train_y:
standing
zero 1
1 1
2 1
three zero
.. ...
155 1[156 rows x 1 columns]
Prediction on prepare knowledge: [1 1 1 zero ... 1]
Accuracy rating on prepare knowledge: zero.6666666666666666Prediction on check knowledge: [1 1 1 1 ... 1
1 1]
Accuracy rating on check knowledge: zero.6666666666666666
The accuracy scores on the prepare and check units are 67% on this instance; its efficiency will be optimized. Do you need to give it a strive? If so, share your strategy within the feedback beneath.
Under the hood
The Naïve Bayes classifier relies on Bayes’ rule or theorem, which computes conditional likelihood, or the chance for an occasion to happen when one other associated occasion has occurred. Stated in easy phrases, it solutions the query: If we all know the likelihood that occasion x occurred earlier than occasion y, then what’s the likelihood that y will happen when x happens once more? The rule makes use of a prior-prediction worth that’s refined progressively to reach at a last posterior worth. A basic assumption of Bayes is that each one parameters are of equal significance.
At a excessive degree, the steps concerned in Bayes’ computation are:
- Compute total posterior possibilities (“Has Parkinson’s” and “Doesn’t have Parkinson’s”)
- Compute possibilities of posteriors throughout all values and every attainable worth of the occasion
- Compute last posterior likelihood by multiplying the outcomes of #1 and #2 for desired occasions
Step 2 will be computationally fairly arduous. Naïve Bayes simplifies it:
- Compute total posterior possibilities (“Has Parkinson’s” and “Doesn’t have Parkinson’s”)
- Compute possibilities of posteriors for desired occasion values
- Compute last posterior likelihood by multiplying the outcomes of #1 and #2 for desired occasions
This is a really fundamental rationalization, and several other different components have to be thought of, comparable to knowledge varieties, sparse knowledge, lacking knowledge, and extra.
Hyperparameters
Naïve Bayes, being a easy and direct algorithm, doesn’t want hyperparameters. However, particular implementations might present superior options. For instance, GaussianNB has two:
- priors: Prior possibilities will be specified as an alternative of the algorithm taking the priors from knowledge.
- var_smoothing: This supplies the power to contemplate data-curve variations, which is useful when the info doesn’t observe a typical Gaussian distribution.
Loss features
Maintaining its philosophy of simplicity, Naïve Bayes makes use of a 0-1 loss function. If the prediction appropriately matches the anticipated final result, the loss is zero, and it is 1 in any other case.
Pros and cons
Pro: Naïve Bayes is without doubt one of the best and quickest algorithms.
Pro: Naïve Bayes provides affordable predictions even with much less knowledge.
Con: Naïve Bayes predictions are estimates, not exact. It favors pace over accuracy.
Con: A basic Naïve Bayes assumption is the independence of all options, however this will not at all times be true.
In essence, Naïve Bayes is an extension of Bayes’ theorem. It is without doubt one of the easiest and quickest machine studying algorithms, meant for simple and fast coaching and prediction. Naïve Bayes supplies good-enough, fairly correct predictions. One of its basic assumptions is the independence of prediction options. Several open supply implementations can be found with traits over and above what can be found within the Bayes algorithm.
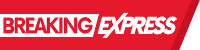