I’m an enormous advocate of studying a number of programming languages. That’s largely as a result of I are inclined to get tired of the languages I take advantage of probably the most. It additionally teaches me new and attention-grabbing methods to method programming.
Writing the identical program in a number of languages is an effective solution to be taught their variations and similarities. Previously, I wrote articles displaying the identical pattern knowledge plotting program written in C & C++, JavaScript with Node.js, and Python and Octave.
This article is a part of one other collection about writing a “guess the number” recreation in numerous programming languages. In this recreation, the pc picks a quantity between one and 100 and asks you to guess it. The program loops till you make an accurate guess.
Learning a brand new language
Venturing into a brand new language all the time feels awkward—I really feel like I’m shedding time since it might be a lot faster to make use of the instruments I do know and use on a regular basis. Luckily, firstly, I’m additionally very passionate about studying one thing new, and this helps me overcome the preliminary ache. And as soon as I be taught a brand new perspective or an answer that I might by no means have considered, issues turn out to be attention-grabbing! Learning new languages additionally helps me backport new methods to my outdated and examined instruments.
When I begin studying a brand new language, I normally search for a tutorial that introduces me to its syntax. Once I’ve a sense for the syntax, I begin engaged on a program I’m accustomed to and search for examples that may adapt to my wants.
What is Racket?
Racket is a programming language within the Scheme family, which is a dialect of Lisp. Lisp can be a household of languages, which may make it laborious to resolve which “dialect” to begin with once you need to be taught Lisp. All of the implementations have varied levels of compatibility, and this plethora of choices may flip away newbies. I feel that could be a pity as a result of these languages are actually enjoyable and stimulating!
Starting with Racket is smart as a result of it is extremely mature and versatile, and the neighborhood may be very lively. Since Racket is a Lisp-like language, a serious attribute is that it makes use of the prefix notation and a lot of parentheses. Functions and operators are utilized to an inventory of operands by prefixing them:
(function-name operand operand ...)(+ 2 three)
↳ Returns 5(record 1 2 three 5)
↳ Returns an inventory containing 1, 2, three, and 5(outline x 1)
↳ Defines a variable referred to as x with worth of 1(outline (f x y) (* x x))
↳ Defines a operate referred to as f with two parameters referred to as x and y that returns their product.
This is principally all there may be to find out about Racket syntax; the remainder is studying the features from the documentation, which may be very thorough. There are different elements of the syntax, like keyword arguments and quoting, however you don’t want them for this instance.
Mastering Racket is likely to be troublesome, and its syntax may look bizarre (particularly if you’re used to languages like Python), however I discover it very enjoyable to make use of. An enormous bonus is Racket’s programming setting, DrRacket, which may be very supportive, particularly if you find yourself getting began with the language.
The main Linux distributions supply packaged variations of Racket, so installation needs to be straightforward.
Guess the quantity recreation in Racket
Here is a model of the “guess the number” program written in Racket:
#lang racket(outline (inquire-user quantity)
(show "Insert a number: ")
(outline guess (string->quantity (read-line)))
(cond [(> quantity guess) (displayln "Too low") (inquire-user quantity)]
[(< quantity guess) (displayln "Too high") (inquire-user quantity)]
[else (displayln "Correct!")]))(displayln "Guess a number between 1 and 100")
(inquire-user (random 1 101))
Save this itemizing to a file referred to as guess.rkt
and run it:
$ racket guess.rkt
Here is a few instance output:
Guess a quantity between 1 and 100
Insert a quantity: 90
Too excessive
Insert a quantity: 50
Too excessive
Insert a quantity: 20
Too excessive
Insert a quantity: 10
Too low
Insert a quantity: 12
Too low
Insert a quantity: 13
Too low
Insert a quantity: 14
Too low
Insert a quantity: 15
Correct!
Understanding this system
I will undergo this system line by line. The first line declares the language the itemizing is written into: #lang racket
. This might sound unusual, however Racket is superb at writing interpreters for brand spanking new domain-specific languages. Do not panic, although! You can use Racket as it’s as a result of it is extremely wealthy in instruments.
Now for the following line. (outline ...)
is used to declare new variables or features. Here, it defines a brand new operate referred to as inquire-user
that accepts the parameter quantity
. The quantity
parameter is the random quantity that the person must guess. The remainder of the code contained in the parentheses of the outline
process is the physique of the inquire-user
operate. Notice that the operate identify comprises a splash; that is Racket’s idiomatic type for writing a protracted variable identify.
This operate recursively calls itself to repeat the query till the person guesses the proper quantity. Note that I’m not utilizing loops; I really feel that Racket programmers don’t like loops and solely use recursive features. This method is idiomatic to Racket, however when you favor, loops are an option.
The first step of the inquire-user
operate asks the person to insert a quantity by writing that string to the console. Then it defines a variable referred to as guess
that comprises regardless of the person entered. The read-line
function returns the person enter as a string. The string is then transformed to a quantity with the string->number
function. After the variable definition, the cond
function accepts a collection of situations. If a situation is glad, it executes the code inside that situation. These situations, (> quantity guess)
and (< quantity guess)
, are adopted by two features: a displayln
that offers clues to the person and a inquire-user
name. The operate calls itself once more when the person doesn’t guess the proper quantity. The else
clause executes when the 2 situations will not be met, i.e., the person enters the proper quantity. The program’s guts are this inquire-user
operate.
However, the operate nonetheless must be referred to as! First, this system asks the person to guess a quantity between 1 and 100, after which it calls the inquire-user
operate with a random quantity. The random quantity is generated with the random
function. You want to tell the operate that you simply need to generate a quantity between 1 and 100, however the random
operate generates integer numbers as much as max-1
, so I used 101.
Try Racket
Learning new languages is enjoyable! I’m an enormous advocate of programming languages polyglotism as a result of it brings new, attention-grabbing approaches and insights to programming. Racket is a superb alternative to begin studying program with a Lisp-like language. I recommend you give it a strive.
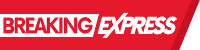