If you are in search of efficiency, complexity, or many potential options to unravel an issue, C ++ is at all times a superb candidate relating to extremes. Of course, performance normally comes with complexity, however some C++ peculiarities are nearly illegible. From my viewpoint, C++ method pointers could be the most complicated expressions I’ve ever come throughout, however I am going to begin with one thing less complicated.
The examples on this article can be found in my GitHub repository.
C: Pointer to features
Let’s start with some fundamentals: Assume you’ve a perform that takes two integers as arguments and returns an integer:
int sum(int a, intb)
In plain C, you may create a pointer to this perform, assign it to your sum(...)
perform, and name it by dereferencing. The perform’s signature (arguments, return kind) should adjust to the pointer’s signature. Aside from that, a perform pointer behaves like an peculiar pointer:
int (*funcPtrOne)(int, int);funcPtrOne = ∑
int resultOne = funcPtrOne(2, 5);
It will get a bit uglier should you take a pointer as an argument and return a pointer:
int *subsequent(int *arrayOfInt)
return ++arrayOfInt;int *(*funcPtrTwo)(int *intPtr);
funcPtrTwo = &subsequent;
int resultTwo = *funcPtrTwo(&array[zero]);
Function pointers in C retailer the deal with of a subroutine.
Pointers to strategies
Let’s step into C++: The excellent news is that you simply most likely will not want to make use of tips to strategies, besides in a couple of uncommon instances, like the next one. First, outline a category with member features you already know:
class MyClass
public:int sum(int a, int b)
;
1. Define a pointer to a technique of a sure class kind
Declare a pointer to a technique of the MyClass
kind. At this level, you do not know the precise methodology you need to name. You’ve solely declared a pointer to some arbitrary MyClass
methodology. Of course, the signature (arguments, return kind) matches the sum(…)
methodology you need to name later:
int (MyClass::*methodPtrOne)(int, int);
2. Assign a sure methodology
In distinction to C (or static member functions), methodology pointers do not level to absolute addresses. Each class kind in C++ has a digital methodology desk (vtable) that shops the deal with offset for every methodology. A way pointer refers to a sure entry within the vtable, so it additionally shops solely the offset worth. This precept additionally permits dynamic dispatch.
Because the signature of the sum(…)
methodology matches your pointer’s declaration, you may assign the signature to it:
methodPtrOne = &MyClass::sum;
three. Invoke the tactic
If you need to invoke the tactic with the pointer, you must present an occasion of the category kind:
MyClass clsInstance;
int outcome = (clsInstance.*methodPtrOne)(2,three);
You can entry the occasion with the .
operator, dereference the pointer with a *
, and thus name the tactic by offering two integers as arguments. Ugly, proper? But you may nonetheless go a step additional.
Using methodology pointers inside a category
Assume you might be creating an software with a client/server precept structure with a backend and a frontend. You do not care concerning the backend for now; as a substitute, you’ll concentrate on the frontend, which is predicated on a C++ class. The frontend’s full initialization depends on knowledge supplied by the backend, so that you want a further initialization mechanism. Also, you need to implement this mechanism generically as a way to prolong your frontend with different initialization strategies sooner or later (possibly dynamically).
First, outline an information kind that may retailer a technique pointer to an initialization methodology (init
) and the knowledge describing when this methodology ought to be referred to as (ticks
):
template<typename T>
struct DynamicInitCommand ;
Here is what the Frontend
class appears to be like like:
class Frontend
{
public:Frontend()
void tick()
unsigned int m_tickszero;
personal:void dynamicInit1()
std::cout << "dynamicInit1 called" << std::endl;
;void dynamicInit2()
std::cout << "dynamicInit2 called" << std::endl;
void dynamicInit3()
unsigned int m_initCntzero;
std::vector<DynamicInitCommand<Frontend> > m_dynamicInit;
};
After Frontend
is instantiated, the tick()
methodology is known as at mounted intervals by the backend. For instance, you may name it each 200ms:
int foremost(int argc, char* argv[])
Frontend frontendInstance;whereas(true)
Frontend
has three extra initialization strategies that should be referred to as based mostly on the worth of m_ticks
. The details about which initialization methodology to name at which tick is saved within the vector m_dynamicInit
. In the constructor (Frontend()
), append this data to the vector in order that the extra initialization features are referred to as after 5, 10, and 15 ticks. When the backend calls the tick()
methodology, the worth m_ticks
is incremented, and also you iterate over the vector m_dynamicInit
to examine whether or not an initialization methodology must be referred to as.
If that is the case, the tactic pointer should be dereferenced by referring to this
:
((*this).*(it->init))()
Summary
Methods pointers can get a bit sophisticated should you’re not acquainted with them. I did loads of trial and error, and it took time to search out the proper syntax. However, when you perceive the final precept, methodology pointers turn out to be much less terrifying.
This is essentially the most complicated syntax I’ve present in C++ to date. Do you realize one thing even worse? Post it within the feedback!
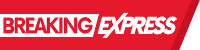