If you wish to be taught enter and output in C, begin by wanting on the stdio.h
embrace file. As you may guess from the title, that file defines all the usual (“std”) enter and output (“io”) features.
The first stdio.h
operate that most individuals be taught is the printf
operate to print formatted output. Or the places
operate to print a easy string. Those are nice features to print data to the person, however if you wish to do greater than that, you will must discover different features.
You can study a few of these features and strategies by writing a duplicate of a typical Linux command. The cp
command will copy one file to a different. If you have a look at the cp
man web page, you will see that cp
helps a broad set of command-line parameters and choices. But within the easiest case, cp
helps copying one file to a different:
cp infile outfile
You can write your personal model of this cp
command in C through the use of only some primary features to learn and write information.
Reading and writing one character at a time
You can simply do enter and output utilizing the fgetc
and fputc
features. These learn and write knowledge one character at a time. The utilization is outlined in stdio.h
and is kind of easy: fgetc
reads (will get) a single character from a file, and fputc
places a single character right into a file.
Writing the cp
command requires accessing information. In C, you open a file utilizing the fopen
operate, which takes two arguments: the title of the file and the mode you wish to use. The mode is often r
to learn from a file or w
to write down to a file. The mode helps different choices too, however for this tutorial, simply concentrate on studying and writing.
Copying one file to a different then turns into a matter of opening the supply and vacation spot information, then studying one character at a time from the primary file, then writing that character to the second file. The fgetc
operate returns both the one character learn from the enter file or the finish of file (EOF
) marker when the file is finished. Once you have learn EOF
, you have completed copying and you’ll shut each information. That code seems like this:
You can write your personal cp
program with this loop to learn and write one character at a time through the use of the fgetc
and fputc
features. The cp.c
supply code seems like this:
#embrace <stdio.h>int
primary(int argc, char **argv)
And you’ll be able to compile that cp.c
file right into a full executable utilizing the GNU Compiler Collection (GCC):
$ gcc -Wall -o cp cp.c
The -o cp
choice tells the compiler to avoid wasting the compiled program into the cp
program file. The -Wall
choice tells the compiler to activate all warnings. If you do not see any warnings, meaning every little thing labored accurately.
Reading and writing blocks of information
Programming your personal cp
command by studying and writing knowledge one character at a time does the job, nevertheless it’s not very quick. You may not discover when copying “everyday” information like paperwork and textual content information, however you will actually discover the distinction when copying giant information or when copying information over a community. Working on one character at a time requires vital overhead.
A greater method to write this cp
command is by studying a piece of the enter into reminiscence (referred to as a buffer), then writing that assortment of information to the second file. This is far quicker as a result of this system can learn extra of the information at one time, which requires fewer “reads” from the file.
You can learn a file right into a variable through the use of the fread
operate. This operate takes a number of arguments: the array or reminiscence buffer to learn knowledge into (ptr
), the scale of the smallest factor you wish to learn (measurement
), what number of of these stuff you wish to learn (nmemb
), and the file to learn from (stream
):
size_t fread(void *ptr, size_t measurement, size_t nmemb, FILE *stream);
The completely different choices present fairly a little bit of flexibility for extra superior file enter and output, akin to studying and writing information with a sure knowledge construction. But within the easy case of studying knowledge from one file and writing knowledge to a different file, you need to use a buffer that’s an array of characters.
And you’ll be able to write the buffer to a different file utilizing the fwrite
operate. This makes use of an identical set of choices to the fread
operate: the array or reminiscence buffer to learn knowledge from, the scale of the smallest factor it is advisable to write, what number of of these issues it is advisable to write, and the file to write down to.
size_t fwrite(const void *ptr, size_t measurement, size_t nmemb, FILE *stream);
In the case the place this system reads a file right into a buffer, then writes that buffer to a different file, the array (ptr
) will be an array of a set measurement. For instance, you need to use a char
array referred to as buffer
that’s 200 characters lengthy.
With that assumption, it is advisable to change the loop in your cp
program to learn knowledge from a file right into a buffer then write that buffer to a different file:
Here’s the complete supply code to your up to date cp
program, which now makes use of a buffer to learn and write knowledge:
#embrace <stdio.h>int
primary(int argc, char **argv)
FILE *infile;
FILE *outfile;
char buffer[200];
size_t buffer_length;/* parse the command line */
/* utilization: cp infile outfile */
if (argc != three)
fprintf(stderr, "Incorrect utilizationn");
fprintf(stderr, "Usage: cp infile outfilen");
return 1;
/* open the enter file */
infile = fopen(argv[1], "r");
if (infile == NULL)
fprintf(stderr, "Cannot open file for studying: %sn", argv[1]);
return 2;
/* open the output file */
outfile = fopen(argv[2], "w");
if (outfile == NULL)
fprintf(stderr, "Cannot open file for writing: %sn", argv[2]);
fclose(infile);
return three;
/* copy one file to the opposite */
/* use fread and fwrite */
whereas (!feof(infile))
/* achieved */
fclose(infile);
fclose(outfile);return zero;
Since you wish to examine this program to the opposite program, save this supply code as cp2.c
. You can compile that up to date program utilizing GCC:
$ gcc -Wall -o cp2 cp2.c
As earlier than, the -o cp2
choice tells the compiler to avoid wasting the compiled program into the cp2
program file. The -Wall
choice tells the compiler to activate all warnings. If you do not see any warnings, meaning every little thing labored accurately.
Yes, it actually is quicker
Reading and writing knowledge utilizing buffers is the higher method to write this model of the cp
program. Because it reads chunks of a file into reminiscence directly, this system does not must learn knowledge as typically. You may not discover a distinction in utilizing both methodology on smaller information, however you will actually see the distinction if it is advisable to copy one thing that is a lot bigger or when copying knowledge on slower media like over a community connection.
I ran a runtime comparability utilizing the Linux time
command. This command runs one other program, then tells you the way lengthy that program took to finish. For my check, I wished to see the distinction in time, so I copied a 628MB CD-ROM picture file I had on my system.
I first copied the picture file utilizing the usual Linux cp
command to see how lengthy that takes. By operating the Linux cp
command first, I additionally eradicated the likelihood that Linux’s built-in file-cache system would not give my program a false efficiency increase. The check with Linux cp
took a lot lower than one second to run:
$ time cp FD13LIVE.iso tmpfileactual 0m0.040s
person 0m0.001s
sys 0m0.003s
Copying the identical file utilizing my very own model of the cp
command took considerably longer. Reading and writing one character at a time took virtually 5 seconds to repeat the file:
$ time ./cp FD13LIVE.iso tmpfileactual 0m4.823s
person 0m4.100s
sys 0m0.571s
Reading knowledge from an enter right into a buffer after which writing that buffer to an output file is far quicker. Copying the file utilizing this methodology took lower than a second:
$ time ./cp2 FD13LIVE.iso tmpfileactual 0m0.944s
person 0m0.224s
sys 0m0.608s
My demonstration cp
program used a buffer that was 200 characters. I am positive this system would run a lot quicker if I learn extra of the file into reminiscence directly. But for this comparability, you’ll be able to already see the massive distinction in efficiency, even with a small, 200 character buffer.
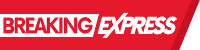