InternetAssembly is a bytecode format that virtually every browser can compile to its host system’s machine code. Alongside JavaScript and WebGL, InternetAssembly fulfills the demand for porting functions for platform-independent use within the net browser. As a compilation goal for C++ and Rust, InternetAssembly allows net browsers to execute code at near-native velocity.
When you discuss a InternetAssembly, software, you should distinguish between three states:
- Source code (e.g., C++ or Rust): You have an software written in a appropriate language that you just wish to execute within the browser.
- InternetAssembly bytecode: You select InternetAssembly bytecode as your compilation goal. As a outcome, you get a
.wasm
file. - Machine code (opcode): The browser masses the
.wasm
file and compiles it to the corresponding machine code of its host system.
InternetAssembly additionally has a textual content format that represents the binary format in human-readable textual content. For the sake of simplicity, I’ll check with this as WASM-text. WASM-text will be in comparison with high-level meeting language. Of course, you wouldn’t write an entire software based mostly on WASM-text, however it’s good to know the way it works below the hood (particularly for debugging and efficiency optimization).
This article will information you thru creating the basic Hello World program in WASM-text.
Creating the .wat file
WASM-text information often finish with .wat
. Start from scratch by creating an empty textual content file named helloworld.wat
, open it along with your favourite textual content editor, and paste in:
(module
;; Imports from JavaScript namespace
(import "console" "log" (func $log (param i32 i32))) ;; Import log operate
(import "js" "mem" (reminiscence 1)) ;; Import 1 web page of reminiscence (54kb)
;; Data part of our module
(information (i32.const zero) "Hello World from WebAssembly!")
;; Function declaration: Exported as hiyaWorld(), no arguments
(func (export "helloWorld")
i32.const zero ;; move offset zero to log
i32.const 29 ;; move size 29 to log (strlen of pattern textual content)
name $log
)
)
The WASM-text format is predicated upon S-expressions. To allow interplay, JavaScript features are imported with the import
assertion, and InternetAssembly features are exported with the export
assertion. For this instance, import the log
operate from the console
module, which takes two parameters of kind i32
as enter and one web page of reminiscence (64KB) to retailer the string.
The string can be written into the information
part at offset zero
. The information
part is an overlay of your reminiscence, and the reminiscence is allotted within the JavaScript half.
Functions are marked with the key phrase func
. The stack is empty when coming into a operate. Function parameters are pushed onto the stack (right here offset and size) earlier than one other operate is named (see name $log
). When a operate returns an f32
kind (for instance), an f32
variable should stay on the stack when leaving the operate (however this isn’t the case on this instance).
Creating the .wasm file
The WASM-text and the InternetAssembly bytecode have 1:1 correspondence. This means you possibly can convert WASM-text into bytecode (and vice versa). You have already got the WASM-text, and now you wish to create the bytecode.
The conversion will be carried out with the WebAssembly Binary Toolkit (WABT). Make a clone of the repository at that hyperlink and comply with the set up directions.
After you construct the toolchain, convert WASM-text to bytecode by opening a console and coming into:
wat2wasm helloworld.wat -o helloworld.wasm
You may also convert bytecode to WASM-text with:
wasm2wat helloworld.wasm -o helloworld_reverse.wat
A .wat
file created from a .wasm
file doesn’t embrace any operate nor parameter names. By default, InternetAssembly identifies features and parameters with their index.
Compiling the .wasm file
Currently, InternetAssembly solely coexists with JavaScript, so you need to write a brief script to load and compile the .wasm
file and do the operate calls. You additionally have to outline the features you’ll import in your InternetAssembly module.
Create an empty textual content file and identify it helloworld.html
, then open your favourite textual content editor and paste in:
nbsp;html>
Simple template
var reminiscence = new InternetAssembly.Memory();operate consoleLogString(offset, size)
var bytes = new Uint8Array(reminiscence.buffer, offset, size);
var string = new TextDecoder('utf8').decode(bytes);
console.log(string);
;var importObject =
console: ,
js :
;
InternetAssembly.instantiateStreaming(fetch('helloworld.wasm'), importObject)
.then(obj => );
</script>
</physique>
</html>
The InternetAssembly.Memory(...)
methodology returns one web page of reminiscence that’s 64KB in measurement. The operate consoleLogString
reads a string from that reminiscence web page based mostly on the size and offset. Both objects are handed to your InternetAssembly module as a part of the importObject
.
Before you possibly can run this instance, you might have to permit Firefox to entry information from this listing by typing about:config
within the tackle line and setting privateness.file_unique_origin
to true
:
Caution: This will make you susceptible to the CVE-2019-11730 safety concern.
Now, open helloworld.html
in Firefox and enter Ctrl+Ok to open the developer console.
Learn extra
This Hello World instance is simply one of many detailed tutorials in MDN’s Understanding WebAssembly text format documentation. If you wish to study extra about InternetAssembly and the way it works below the hood, check out these docs.
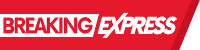