When you are scripting with Bash, generally it is advisable learn information from or write information to a file. Sometimes a file might comprise configuration choices, and different instances the file is the information your consumer is creating along with your software. Every language handles this process a little bit in another way, and this text demonstrates methods to deal with information information with Bash and different POSIX shells.
Install Bash
If you are on Linux, you in all probability have already got Bash. If not, you could find it in your software program repository.
On macOS, you should use the default terminal, both Bash or Zsh, relying on the macOS model you are working.
On Windows, there are a number of methods to expertise Bash, together with Microsoft’s formally supported Windows Subsystem for Linux (WSL).
Once you could have Bash put in, open your favourite textual content editor and prepare to code.
Reading a file with Bash
In addition to being a shell, Bash is a scripting language. There are a number of methods to learn information from Bash: You can create a kind of information stream and parse the output, or you’ll be able to load information into reminiscence. Both are legitimate strategies of ingesting data, however every has fairly particular use instances.
Source a file in Bash
When you “source” a file in Bash, you trigger Bash to learn the contents of a file with the expectation that it incorporates legitimate information that Bash can match into its established information mannequin. You will not supply information from any outdated file, however you should use this methodology to learn configuration information and features.
For occasion, create a file referred to as instance.sh
and enter this into it:
#!/bin/shgreet opensource.com
echo "The which means of life is $var"
Run the code to see it fail:
$ bash ./instance.sh
./instance.sh: line three: greet: command not discovered
The which means of life is
Bash would not have a command referred to as greet
, so it couldn’t execute that line, and it has no report of a variable referred to as var
, so there isn’t a identified which means of life. To repair this downside, create a file referred to as embody.sh
:
greet()
echo "Hello $"var=42
Revise your instance.sh
script to incorporate a supply
command:
#!/bin/shsupply embody.sh
greet opensource.com
echo "The which means of life is $var"
Run the script to see it work:
$ bash ./instance.sh
Hello opensource.com
The which means of life is 42
The greet
command is introduced into your shell surroundings as a result of it’s outlined within the embody.sh
file, and it even acknowledges the argument (opensource.com
on this instance). The variable var
is ready and imported, too.
Parse a file in Bash
The different option to get information “into” Bash is to parse it as a knowledge stream. There are some ways to do that. You can use grep
or cat
or any command that takes information and pipes it to stdout. Alternately, you should use what’s constructed into Bash: the redirect. Redirection by itself is not very helpful, so on this instance, I additionally use the built-in echo
command to print the outcomes of the redirect:
#!/bin/shecho $( < embody.sh )
Save this as stream.sh
and run it to see the outcomes:
$ bash ./stream.sh
greet() var=42
$
For every line within the embody.sh
file, Bash prints (or echoes) the road to your terminal. Piping it first to an acceptable parser is a standard option to learn information with Bash. For occasion, assume for a second that embody.sh
is a configuration file with key and worth pairs separated by an equal (=
) signal. You might get hold of values with awk
and even minimize
:
#!/bin/shmyVar=`grep var embody.sh | minimize -d'=' -f2`
echo $myVar
Try working the script:
Writing information to a file with Bash
Whether you are storing information your consumer created along with your software or simply metadata about what the consumer did in an software (for example, recreation saves or latest songs performed), there are various good causes to retailer information for later use. In Bash, it can save you information to information utilizing frequent shell redirection.
For occasion, to create a brand new file containing output, use a single redirect token:
#!/bin/shTZ=UTC
date > date.txt
Run the script a couple of instances:
$ bash ./date.sh
$ cat date.txt
Tue Feb 23 22:25:06 UTC 2021
$ bash ./date.sh
$ cat date.txt
Tue Feb 23 22:25:12 UTC 2021
To append information, use the double redirect tokens:
#!/bin/shTZ=UTC
date >> date.txt
Run the script a couple of instances:
$ bash ./date.sh
$ bash ./date.sh
$ bash ./date.sh
$ cat date.txt
Tue Feb 23 22:25:12 UTC 2021
Tue Feb 23 22:25:17 UTC 2021
Tue Feb 23 22:25:19 UTC 2021
Tue Feb 23 22:25:22 UTC 2021
Bash for straightforward programming
Bash excels at being simple to study as a result of, with just some primary ideas, you’ll be able to construct complicated applications. For the total documentation, consult with the excellent Bash documentation on GNU.org.
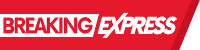