Jenkins is a free and open supply automation server for constructing, testing, and deploying code. It’s the spine of steady integration and steady supply (CI/CD) and may save builders hours every day and defend them from having failed code go reside. When code does fail, or when a developer must see the output of exams, Jenkins offers log information for assessment.
The default Jenkins pipeline logs might be tough to learn. This fast abstract of Jenkins logging fundamentals presents some suggestions (and code) on learn how to make them extra readable.
What you get
Jenkins pipelines are cut up into stages. Jenkins mechanically logs the start of every stage, like this:
[Pipeline] // stage
[Pipeline] stage (disguise)
[Pipeline] { (Apply all openshift sources)
[Pipeline] dir
The textual content is displayed with out a lot distinction, and essential issues (like the start of a stage) aren’t highlighted. In a pipeline log a number of hundred strains lengthy, discovering the place one stage begins and one other ends, particularly in the event you’re casually shopping the logs in search of a specific stage, might be daunting.
Jenkins pipelines are written as a mixture of Groovy and shell scripting. In the Groovy code, logging is sparse; many instances, it consists of grayed-out textual content within the command with out particulars. In the shell scripts, debugging mode (set -x
) is turned on, so each shell command is totally realized (variables are dereferenced and values printed) and logged intimately, as is the output.
It might be tedious to learn by way of the logs to get related data, on condition that there might be a lot. Since the Groovy logs that proceed and comply with a shell script in a pipeline aren’t very expressive, many instances they lack context:
[Pipeline] dir
Running in /house/jenkins/agent/workspace/devop-master/devops-server-pipeline/my-repo-dir/src
[Pipeline]
I can see what listing I’m working in, and I do know I used to be looking for file(s) and studying a YAML file utilizing Jenkins’ steps. But what was I in search of, and what did I discover and skim?
What might be executed?
I am glad you requested as a result of there are just a few easy practices and a few small snippets of code that may assist. First, the code:
def echoBanner(def ... msgs)def errorBanner(def ... msgs)
error(createBanner(msgs))def createBanner(def ... msgs)
// flatten operate hack included in case Jenkins safety
// is about to preclude calling Groovy flatten() static technique
// NOTE: works effectively on all nested collections besides a Map
def msgFlatten(def checklist, def msgs)
Add this code to the top of every pipeline or, to be extra environment friendly, load a Groovy file or make it a part of a Jenkins shared library.
At the beginning of every stage (or at explicit factors inside a stage), merely name echoBanner
:
echoBanner("MY STAGE", ["DOING SOMETHING 1", "DOING SOMETHING 2"])
Your logs in Jenkins will show the next:
===========================================MY STAGE
DOING SOMETHING 1
DOING SOMETHING 2===========================================
The banners are very straightforward to pick within the logs. They additionally assist outline the pipeline move when used correctly, and so they break the logs up properly for studying.
I’ve used this for some time now professionally in just a few locations. The suggestions has been very optimistic concerning serving to make pipeline logs extra readable and the move extra comprehensible.
The errorBanner
technique above works the identical manner, however it fails the script instantly. This helps spotlight the place and what precipitated the failure.
Best practices
- Use
echo
Jenkins steps liberally all through your Groovy code to tell the person what you are doing. These may also assist with documenting your code. - Use empty log statements (an empty echo step in Groovy,
echo ''
, or simplyecho
in shell) to interrupt up the output for simpler readability. You most likely use empty strains in your code for a similar objective. - Avoid the entice of utilizing
set +x
in your scripts, which hides logging executed shell statements. It would not a lot clear up your logs because it makes your pipelines a black field that hides what your pipeline is doing and any errors that seem. Make certain your pipelines’ performance is as clear as potential. - If your pipeline creates intermediate artifacts that builders and/or DevOps personnel might use to assist debug points, then log their contents, too. Yes, it makes the logs longer, however it’s solely textual content. It shall be helpful data in some unspecified time in the future, and what else is a log (if utilized correctly) than a wealth of details about what occurred and why?
Kubernetes Secrets: Where full transparency will not work
There are some issues that you just do not need to find yourself in your logs and be uncovered. If you are utilizing Kubernetes and referencing information held in a Kubernetes Secret, then you definately positively don’t desire that information uncovered in a log as a result of the information is barely obfuscated and never encrypted.
Imagine you need to take some information held in a Secret and inject it right into a templated JSON file. (The full contents of the Secret and the JSON template are irrelevant for this instance.) You need to be clear and log what you are doing since that is finest follow, however you do not need to expose your Secret information.
Change your script’s mode from debugging (set -x
) to command logging (set -v
). At the top of the delicate portion of the script, reset the shell to debugging mode:
sh """
# change script mode from debugging to command logging
set +x -v# seize information from secret in shell variable
MY_SECRET=$(kubectl get secret my-secret --no-headers -o 'custom-column=:.information.my-secret-data')# substitute template placeholder inline
sed s/%TEMPLATE_PARAM%/$/ my-template-file.json# do one thing with modified template-file.json...
# reset the shell to debugging mode
""
set -x +v
"
This will output this line to the logs:
sed s/%TEMPLATE_PARAM%/$/ my-template-file.json
This would not notice the shell variable MY_SECRET_DATA
, not like in shell debug mode. Obviously, this is not as useful as debug mode if an issue happens at this level within the pipeline and also you’re attempting to determine what went flawed. But it is one of the best stability between protecting your pipeline execution clear for each builders and DevOps whereas additionally protecting your Secrets hidden.
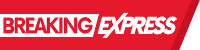