This is the fourth in a collection of articles about options that first appeared in a model of Python three.x. Python three.three was first launched in 2012, and although it has been out for a very long time, lots of the options it launched are underused and fairly cool. Here are three of them.
yield from
The yield
key phrase made Python far more highly effective. Predictably, everybody began utilizing it to create a complete ecosystem of iterators. The itertools module and the more-itertools PyPI bundle are simply two examples.
Sometimes, a brand new generator will need to use an present generator. As a easy (if considerably contrived) instance, think about you need to enumerate all pairs of pure numbers.
One technique to do it’s to generate all pairs within the order of sum of pair, first merchandise of pair
. Implementing this with yield from
is pure.
The yield from <x>
key phrase is brief for:
for merchandise in x:
yield merchandise
import itertoolsdef pairs():
for n in itertools.rely():
yield from ((i, n-i) for i in vary(n+1))
checklist(itertools.islice(pairs(), 6))
[(0, 0), (0, 1), (1, 0), (0, 2), (1, 1), (2, 0)]
Implicit namespace packages
Imagine a fictional firm referred to as Parasol that makes a bunch of stuff. Much of its inside software program is written in Python. While Parasol has open sourced a few of its code, a few of it’s too proprietary or specialised for open supply.
The firm makes use of an inside DevPI server to handle the inner packages. It doesn’t make sense for each Python programmer at Parasol to search out an unused identify on PyPI, so all the inner packages are referred to as parasol.<enterprise division>.<mission>
. Observing greatest practices, the builders need the bundle names to replicate that naming system.
This is essential! If the bundle parasol.accounting.numeric_tricks
installs a top-level module referred to as numeric_tricks
, this implies no one who relies on this bundle will have the ability to use a PyPI bundle that is named numeric_tricks
, irrespective of how nifty it’s.
However, this leaves the builders with a dilemma: Which bundle owns the parasol/__init__.py
file? The greatest resolution, beginning in Python three.three, is to make parasol
, and doubtless parasol.accounting
, to be namespace packages, which do not have the __init__.py
file.
Suppressing exception context
Sometimes, an exception in the midst of a restoration from an exception is an issue, and having the context to hint it’s helpful. However, typically it isn’t: the exception has been dealt with, and the brand new state of affairs is a unique error situation.
For instance, think about that after failing to search for a key in a dictionary, you need to fail with a ValueError()
if it can’t be analyzed:
import timedef expensive_analysis(knowledge):
time.sleep(10)
if knowledge[zero:1] == ">":
return knowledge[1:]
return None
This perform takes a very long time, so while you use it, you need to cache the outcomes:
cache =def last_letter_analyzed(knowledge):
strive:
analyzed = cache[knowledge]
besides KeyError:
analyzed = expensive_analysis(knowledge)
if analyzed is None:
increase ValueError("invalid data", knowledge)
cached[knowledge] = analyzed
return analyzed[-1]
Unfortunately, when there’s a cache miss, the traceback seems to be ugly:
last_letter_analyzed("stuff")
---------------------------------------------------------------------------KeyError Traceback (most up-to-date name final)
<ipython-input-16-a525ae35267b> in last_letter_analyzed(knowledge)
four strive:
----> 5 analyzed = cache[data]
6 besides KeyError:KeyError: 'stuff'
During dealing with of the above exception, one other exception happens:
ValueError Traceback (most up-to-date name final)<ipython-input-17-40dab921f9a9> in <module>
----> 1 last_letter_analyzed("stuff")
<ipython-input-16-a525ae35267b> in last_letter_analyzed(knowledge)
7 analyzed = expensive_analysis(knowledge)
eight if analyzed is None:
----> 9 increase ValueError("invalid data", knowledge)
10 cached[data] = analyzed
11 return analyzed[-1]ValueError: ('invalid knowledge', 'stuff')
If you employ increase ... from None
, you may get far more readable tracebacks:
def last_letter_analyzed(knowledge):
strive:
analyzed = cache[knowledge]
besides KeyError:
analyzed = expensive_analysis(knowledge)
if analyzed is None:
increase ValueError("invalid data", knowledge) from None
cached[knowledge] = analyzed
return analyzed[-1]
last_letter_analyzed("stuff")
---------------------------------------------------------------------------ValueError Traceback (most up-to-date name final)
<ipython-input-21-40dab921f9a9> in <module>
----> 1 last_letter_analyzed("stuff")
<ipython-input-20-5691e33edfbc> in last_letter_analyzed(knowledge)
5 analyzed = expensive_analysis(knowledge)
6 if analyzed is None:
----> 7 increase ValueError("invalid data", knowledge) from None
eight cached[data] = analyzed
9 return analyzed[-1]ValueError: ('invalid knowledge', 'stuff')
Welcome to 2012
Although Python three.three was launched nearly a decade in the past, lots of its options are nonetheless cool—and underused. Add them to your toolkit if you have not already.
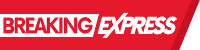