This is the fifth in a collection of articles about options that first appeared in a model of Python three.x. Python three.four was first launched in 2014, and although it has been out for a very long time, most of the options it launched are underused and fairly cool. Here are three of them.
enum
One of my favourite logic puzzles is the self-descriptive Hardest Logic Puzzle Ever. Among different issues, it talks about three gods who’re known as A, B, and C. Their identities are True, False, and Random, in some order. You can ask them questions, however they solely reply within the god language, the place “da” and “ja” imply “yes” and “no,” however you have no idea which is which.
If you determine to make use of Python to resolve the puzzle, how would you signify the gods’ names and identities and the phrases within the god language? The conventional reply has been to make use of strings. However, strings could be misspelled with disastrous penalties.
If, in a essential a part of your resolution, you evaluate to the string jaa
as a substitute of ja
, you’ll have an incorrect resolution. While the puzzle doesn’t specify what the stakes are, that is in all probability finest averted.
The enum
module offers you the power to outline these items in a debuggable but protected method:
import enum@enum.distinctive
class Name(enum.Enum):
A = enum.auto()
B = enum.auto()
C = enum.auto()
@enum.distinctive
class Identity(enum.Enum):
RANDOM = enum.auto()
TRUE = enum.auto()
FALSE = enum.auto()
@enum.distinctive
class Language(enum.Enum):
ja = enum.auto()
da = enum.auto()
One benefit of enums is that in debugging logs or exceptions, the enum is rendered helpfully:
identify = Name.A
id = Identity.RANDOM
reply = Language.da
print("I suspect", identify, "is", id, "because they answered", reply)
I believe Name.A is Identity.RANDOM as a result of they answered Language.da
While creating the “infrastructure” layer of a recreation, you need to cope with numerous recreation objects generically however nonetheless permit the objects to customise actions. To make the instance simpler to clarify, assume it is a text-based recreation. When you employ an object, more often than not, it is going to simply print You are utilizing <x>
. But utilizing a particular sword would possibly require a random roll, and it’ll fail in any other case.
When you purchase an object, it’s normally added to the stock. However, a very heavy rock will smash a random object; if that occurs, the stock will lose that object.
One method to strategy that is to have strategies use
and purchase
on objects. More and extra of those strategies might be added as the sport’s complexity will increase, making recreation objects unwieldy to jot down.
Instead, functools.singledispatch
means that you can add strategies retroactively—in a protected and namespace-respecting method.
You can outline lessons with no habits:
class Torch:
identify="torch"class Sword:
identify="sword"class Rock:
identify="rock"
import functools@functools.singledispatch
def use(x):
print("You use", x.identify)@functools.singledispatch
def purchase(x, stock):
stock.add(x)
For the torch, these generic implementations are sufficient:
stock = set()def deploy(factor):
purchase(factor, stock)
use(factor)
print("You have", [merchandise.identify for merchandise in stock])deploy(Torch())
You use torch
You have ['torch']
However, the sword and the rock want some specialised performance:
import random@use.register(Sword)
def use_sword(sword):
print("You try to use", sword.identify)
if random.random() < zero.9:
print("You succeed")
else:
print("You fail")deploy(sword)
You attempt to use sword
You succeed
You have ['sword', 'torch']
import random@purchase.register(Rock)
def acquire_rock(rock, stock):
to_remove = random.selection(record(stock))
stock.take away(to_remove)
stock.add(rock)deploy(Rock())
You use rock
You have ['sword', 'rock']
The rock may need crushed the torch, however your code is far simpler to learn.
pathlib
The interface to file paths in Python has been “smart-string manipulation” for the reason that starting of time. Now, with pathlib
, Python has an object-oriented method to manipulate paths:
import pathlib
gitconfig = pathlib.Path.residence() / ".gitconfig"
textual content = gitconfig.read_text().splitlines()
Admittedly, utilizing /
as an operator to generate path names is a bit cutesy, but it surely finally ends up being good in observe. Methods like .read_text()
permit you to get textual content out of small recordsdata without having to open and shut file handles manually.
This permits you to focus on the vital stuff:
for line in textual content:
if not line.strip().startswith("name"):
proceed
print(line.cut up("=")[1])
Moshe Zadka
Welcome to 2014
Python three.four was launched about seven years in the past, however a number of the options that first confirmed up on this launch are cool—and underused. Add them to your toolkit if you have not already.
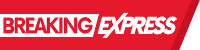