Web parts are a set of open supply applied sciences similar to JavaScript and HTML that can help you create customized components that you should utilize and reuse in net apps. The parts you create are unbiased of the remainder of your code, so that they’re simple to reuse throughout many initiatives.
Best of all, it is a platform normal supported by all main trendy browsers.
What’s in an internet element?
- Custom components: This JavaScript API lets you outline new sorts of HTML components.
- Shadow DOM: This JavaScript API supplies a option to connect a hidden separate Document Object Model (DOM) to a component. This encapsulates your net element by holding the styling, markup construction, and habits remoted from different code on the web page. It ensures that types will not be overridden by exterior types or, conversely, that a model out of your net element does not “leak” into the remainder of the web page.
- HTML templates: The factor lets you outline reusable DOM components. The factor and its contents will not be rendered within the DOM however can nonetheless be referenced utilizing JavaScript.
Write your first net element
You can write a easy net element along with your favourite textual content editor and JavaScript. This how-to makes use of bootstrap to generate easy stylings then creates a easy card net element to show the temperature of a location handed to it as an attribute. The element makes use of the Open Weather API, which requires you to generate an APPID/APIKey by signing in.
The syntax of calling this net element requires the situation’s longitude and latitude:
<weather-card longitude='85.8245' latitude='20.296' />
Create a file named weather-card.js that may include all of the code on your net element. Start by defining your element. This may be achieved by making a template factor and including some easy HTML components into it:
const template = doc.createElement('template');template.innerHTML = `
<div class="card">
<div class="card-body"></div>
</div>
`
Start defining the WebElement class and its constructor:
class WeatherCard extends HTMLElement
The constructor attaches the shadowRoot and units it to open mode. Then the template is cloned to shadowRoot.
Next, entry the attributes. These are the longitude and latitude, and also you want them to make a GET request to the Open Weather API. This must be achieved within the connectedCallback
perform. You can use the getAttribute
technique to entry the attributes or outline getters to bind them to this object:
get longitude()
return this.getAttribute('longitude');get latitude()
Now outline the connectedCallBack
technique that fetches climate information each time it’s mounted:
connectedCallback()
Once the climate information is retrieved, extra HTML components are added to the template. Now, your class is outlined.
Finally, outline and register a brand new customized factor by utilizing the tactic window.customElements.outline
:
window.customElements.outline('weather-card', WeatherCard);
The first argument is the title of the customized factor, and the second argument is the outlined class. Here’s a link to the entire component.
You’ve written your first net element! Now it is time to convey it to the DOM. To try this, you should load the JavaScript file along with your net element definition in your HTML file (title it index.html):
<head>
<meta charset="UTF-8">
</head><physique>
<weather-card longitude='85.8245' latitude='20.296'></weather-card>
<script src="http://opensource.com/./weather-card.js"></script>
</physique></html>
Here’s your net element in a browser:
Because net parts want solely HTML, CSS, and JavaScript, they’re natively supported by browsers and can be utilized seamlessly with frontend frameworks, together with React and Vue. The following easy code snippet exhibits the right way to use net parts with a easy React App bootstrapped with Create React App. For this, you must import the weather-card.js file you outlined earlier and use it as a element:
import './App.css';
import './weather-card';perform App()
export default App;
Lifecycle of an internet element
All parts comply with a lifecycle from initialization to elimination from the DOM (i.e., unmount). Methods are related to every lifecycle occasion as a way to management the parts higher. The numerous lifecycle occasions of an internet element embrace:
Modular open supply
Web parts is usually a highly effective option to develop net apps. Whether you are comfy with JavaScript or simply getting began with it, it is easy to create reusable code with this nice open normal, it doesn’t matter what browser your target market makes use of.
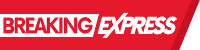