JavaScript is a programming language stuffed with nice surprises. Many folks first encounter JavaScript as a language for the online. There’s a JavaScript engine in all the key browsers, there are widespread frameworks equivalent to JQuery, Cash, and Bootstrap to assist make internet design simpler, and there are even programming environments written in JavaScript. It appears to be all over the place on the web, nevertheless it seems that it is also a helpful language for tasks like Electron, an open supply toolkit for constructing cross-platform desktop apps with JavaScript.
JavaScript is a surprisingly multipurpose language with a large assortment of libraries for far more than simply making web sites. Learning the fundamentals of the language is straightforward, and it is a gateway to constructing no matter you think about.
Install JavaScript
As you progress with JavaScript, it’s possible you’ll end up wanting superior JavaScript libraries and runtimes. When you are simply beginning, although, you do not have to put in JavaScript in any respect. All main internet browsers embody a JavaScript engine to run the code. You can write JavaScript utilizing your favourite textual content editor, load it into your internet browser, and see what your code does.
Get began with JavaScript
To write your first JavaScript code, open your favourite textual content editor, equivalent to Notepad++, Atom, or VSCode. Because it was developed for the online, JavaScript works nicely with HTML, so first, simply strive some fundamental HTML:
Save the file, after which open it in an internet browser.
To add JavaScript to this straightforward HTML web page, you possibly can both create a JavaScript file and confer with it within the web page’s head
or simply embed your JavaScript code within the HTML utilizing the <script>
tag. In this instance, I embed the code:
Reload the web page in your browser.
As you possibly can see, the <p>
tag as written nonetheless accommodates the string “Nothing here,” however when it is rendered, JavaScript alters it in order that it accommodates “Hello world” as a substitute. Yes, JavaScript has the facility to rebuild (or simply assist construct) a webpage.
The JavaScript on this easy script does two issues. First, it creates a variable referred to as myvariable
and locations the string “Hello world!” into it. Finally, it searches the present doc (the online web page because the browser is rendering it) for any HTML aspect with the ID instance
. When it locates instance
, it makes use of the innerHTML
operate to interchange the contents of the HTML aspect with the contents of myvariable
.
Of course, utilizing a customized variable is not crucial. It’s simply as straightforward to populate the HTML aspect with one thing being dynamically created. For occasion, you possibly can populate it with a timestamp:
Reload the web page to see a timestamp generated in the mean time the web page is rendered. Reload a couple of instances to observe the seconds increment.
JavaScript syntax
In programming, syntax refers back to the guidelines of how sentences (or “lines”) are written. In JavaScript, every line of code should finish in a semicolon (;
) in order that the JavaScript engine operating your code understands when to cease studying.
Words (or “strings”) should be enclosed in citation marks ("
), whereas numbers (or “integers”) go with out.
Almost the whole lot else is a conference of the JavaScript language, equivalent to variables, arrays, conditional statements, objects, features, and so forth.
Creating variables in JavaScript
Variables are containers for information. You can consider a variable as a field the place you possibly can put information to share together with your program. Creating a variable in JavaScript is finished with two key phrases you select primarily based on how you propose to make use of the variable: let
and var
. The var
key phrase denotes a variable supposed in your whole program to make use of, whereas let
creates variables for particular functions, often inside features or loops.
JavaScript’s built-in typeof
operate might help you determine what sort of information a variable accommodates. Using the primary instance, yow will discover out what sort of information myvariable
accommodates by modifying the displayed textual content to:
<string>
let myvariable = "Hello world!";
doc.getElementById("example").innerHTML = typeof(myvariable);
</string>
This renders “string” in your internet browser as a result of the variable accommodates “Hello world!” Storing completely different sorts of knowledge (equivalent to an integer) in myvariable
would trigger a unique information kind to be printed to your pattern internet web page. Try altering the contents of myvariable
to your favourite quantity after which reloading the web page.
Creating features in JavaScript
Functions in programming are self-contained information processors. They’re what makes programming modular. It’s as a result of features exist that programmers can write generic libraries that, as an example, resize photos or hold monitor of the passage of time for different programmers (such as you) to make use of in their very own code.
You create a operate by offering a customized title in your operate adopted by any quantity of code enclosed inside braces.
Here’s a easy internet web page that includes a resized picture and a button that analyzes the picture and returns the true picture dimensions. In this instance code, the <button>
HTML aspect makes use of the built-in JavaScript operate onclick
to detect consumer interplay, which triggers a customized operate referred to as get_size
:
Save the file and cargo it into your internet browser to strive the code.
Cross-platform apps with JavaScript
You can see from the code pattern how JavaScript and HTML work carefully collectively to create a cohesive consumer expertise. This is likely one of the nice strengths of JavaScript. When you write code in JavaScript, you inherit one of the widespread consumer interfaces of recent computing no matter platform: the online browser. Your code is cross-platform by nature, so your software, whether or not it is only a humble picture measurement analyzer or a fancy picture editor, online game, or no matter else you dream up, can be utilized by everybody with an internet browser (or a desktop, when you ship an Electron app).
Learning JavaScript is straightforward and enjoyable. There are a number of web sites with tutorials out there. There are additionally over one million JavaScript libraries that will help you interface with units, peripherals, the Internet of Things, servers, file techniques, and much extra. And as you are studying, hold our JavaScript cheat sheet shut by so that you bear in mind the positive particulars of syntax and construction.
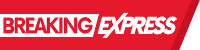