Adding a light and dark mode to my facet undertaking www.fediverse.to was a enjoyable journey. I particularly liked how intuitive the whole course of was. The prefers-color-scheme CSS property accommodates the person’s coloration scheme—gentle or darkish. I then outline SASS or CSS kinds for each modes, and the browser applies the model the person needs. That’s it! The seamless move from working system to browser to web site is a big win for customers and builders.
After tinkering with www.fediverse.to I made a decision so as to add gentle and darkish modes to this web site as effectively. I started with some web analysis on how you can greatest strategy this. This GitHub thread reveals the present progress of the characteristic. And this in-depth POC demonstrates how difficult the method might be.
The problem
The largest problem is that generally SASS and CSS do not play effectively with one another.
Let me clarify.
From my earlier submit on gentle and darkish themes, to create each kinds I wanted to outline CSS like this:
/* Light mode */
:root {
--body-bg: #FFFFFF;
--body-color: #000000;
}/* Dark mode */
@media (prefers-color-scheme: darkish) {
:root {
--body-bg: #000000;
--body-color: #FFFFFF;
}
}
This is straightforward sufficient. With the kinds outlined, I take advantage of var(–body-bg) and var(–body-color) in our CSS. The colours to change based mostly on the worth of prefers-color-scheme.
Bootstrap 5 makes use of Sass to outline coloration values. My web site’s color scheme in _variables.scss seems to be like this:
// User-defined colours
$my-link-color: #FFCCBB !default;
$my-text-color: #E2E8E4 !default;
$my-bg-color: #303C6C;
The answer appears apparent now, proper? I can mix prefers-color-scheme with the variables above, and growth!
// User-defined colours
:root {
--my-link-color: #FFCCBB;
--my-text-color: #E2E8E4;
--my-bg-color: #303C6C;
}/* Dark mode */
@media (prefers-color-scheme: darkish) {
:root {
--my-link-color: #FF0000;
--my-text-color: #FFFFFF;
--my-bg-color: #000000;
}
}
Additionally, I would like to exchange the $ values with their — variants in _variables.scss. After making the change and working jekyll construct, I get the next:
Conversion error: Jekyll::Converters::Scss encountered an error whereas changing 'css/predominant.scss':
Error: argument `$color2` of `combine($color1, $color2, $weight: 50%)` have to be a coloration on line 161:11 of _sass/_functions.scss, in perform `combine` from line 161:11 of _sass/_functions.scss, in perform `shade-color` from line 166:27 of _sass/_functions.scss, in perform `if` from line 166:11 of _sass/_functions.scss, in perform `shift-color` from line 309:43 of _sass/_variables.scss from line 11:9 of _sass/bootstrap.scss from line 1:9 of stdin >> @return combine(black, $coloration, $weight); ----------^
Error: Error: argument `$color2` of `combine($color1, $color2, $weight: 50%)` have to be a coloration on line 161:11 of _sass/_functions.scss, in perform `combine` from line 161:11 of _sass/_functions.scss, in perform `shade-color` from line 166:27 of _sass/_functions.scss, in perform `if` from line 166:11 of _sass/_functions.scss, in perform `shift-color` from line 309:43 of _sass/_variables.scss from line 11:9 of _sass/bootstrap.scss from line 1:9 of stdin >> @return combine(black, $coloration, $weight); ----------^
Error: Run jekyll construct --trace for extra data.
The error signifies that the Bootstrap mixins count on coloration values to be, effectively, coloration values, and never CSS variables. From right here, dig down into the Bootstrap code to rewrite the mixin. But I have to rewrite most of Bootstrap to get this to work. This page describes a lot of the choices accessible at this level. But I used to be in a position to make do with a less complicated strategy.
Since I do not use the whole suite of Bootstrap options, I used to be ready so as to add gentle and darkish mode with a mix of prefers-color-scheme, some CSS overrides, and just a little little bit of code duplication.
Step 1: Separate presentation from construction
Before making use of the brand new kinds to deal with gentle and darkish modes, I carried out some clean-up on the HTML and CSS.
The first step is guaranteeing that every one the presentation layer stuff is within the CSS and never the HTML. The presentation markup (CSS) ought to all the time keep separate from the web page construction (HTML). But an internet site’s supply code can get messy with time. You can skip this step in case your coloration lessons are already separated into CSS.
I discovered my HTML code peppered with Bootstrap coloration lessons. Certain div and footer tag used text-light, text-dark, bg-light, and bg-dark throughout the HTML. Since dealing with the sunshine and darkish theme depends on CSS, the colour lessons needed to go. So I moved them all from the HTML into my custom SASS file.
I left the contextual coloration lessons (bg-primary, bg-warning, text-muted, and so forth.) as-is. The colours I’ve picked for my gentle and darkish themes wouldn’t intrude with them. Make certain your theme colours work effectively with contextual colours. Otherwise, you need to transfer them into the CSS as effectively.
So far, I’ve written 100+ articles on this website. So I needed to scan all my posts below the _posts/
listing looking down coloration lessons. Like the step above, make sure that to maneuver all coloration lessons into the CSS. Don’t overlook to test the Jekyll collections and pages as effectively.
Step 2: Consolidate kinds wherever attainable
Consolidating and reusing styling components ensures you’ve much less to fret about. My Projects and Featured Writing sections on the house web page displayed card-like layouts. These have been utilizing customized CSS styling of their very own. I restyled them to match the article links and now I’ve much less to fret about.
There have been a number of different components utilizing kinds of their very own. Instead of restyling them, I selected to take away them.
The footer, for instance, used its personal background coloration. This would have required two totally different colours for gentle and darkish themes. I selected to take away the background from the footer to simplify the migration. The footer now takes the color of the background.
If your web site makes use of too many kinds, it is perhaps prudent to take away them for the migration. After the transfer to gentle/darkish themes is full, you’ll be able to add them again.
The purpose is to maintain the migration easy and add new kinds later if required.
Step 3: Add the sunshine and darkish coloration schemes
With the clean-up full, I can now give attention to including the styling components for gentle and darkish themes. I outline the brand new coloration kinds and apply them to the HTML components. I selected to begin with the next:
- –body-bg for the background coloration.
- –body-color for the primary physique/textual content coloration.
- –body-link-color for the hyperlinks.
- –card-bg for the Bootstrap Card background colours.
:root {
--body-bg: #EEE2DC;
--body-color: #AC3B61;
--body-link-color: #AC3B61;
--card-bg: #EDC7B7;
}/* Dark mode */
@media (prefers-color-scheme: darkish) {
:root {
--body-bg: #303C6C;
--body-color: #E2E8E4;
--body-link-color: #FFCCBB;
--card-bg: #212529;
}
}
With the colours outlined, I modified the CSS to make use of the brand new colours. For instance, the physique component now seems to be like this:
physique {
background-color: var(--body-bg);
coloration: var(--body-color) !vital;
}
You can view the rest of the CSS changes on GitLab.
You can override Bootstrap 5 defaults if it is compiled together with your Jekyll supply and never from the CDN. This would possibly make sense to simplify the customized styling it is advisable deal with. For instance, turning off link decoration made life just a little simpler for me.
$link-hover-decoration: none !default;
Step 4: The Navbar Toggler
Last however not least: The navbar toggler. In Bootstrap 5, navbar-light and navbar-dark management the colour of the toggler. These are outlined in the primary nav component and .navbar. Since I’m not hard-coding coloration lessons within the HTML anymore, I have to duplicate the CSS. I extended the default Sass and added my theme colors.
.navbar-toggler {
@lengthen .navbar-toggler;
coloration: var(--text-color);
border-color: var(--text-color);
}.navbar-toggler-icon {
@lengthen .navbar-toggler-icon;
background-image: escape-svg(url("data:image/svg+xml,<svg xmlns="http://www.w3.org/2000/svg" viewBox='0 0 30 30'><path stroke="#000000" stroke-linecap='round' stroke-miterlimit="10" stroke-width="2" d='M4 7h22M4 15h22M4 23h22'/></svg>"));
}
The code above is the default Bootstrap 5 toggler CSS code, with some minor modifications. One factor to notice right here: For the toggler icon, I hardcoded stroke= #000000 since black works with my theme colours. You could have to be extra artistic about selecting colours schemes that work effectively throughout the board.
(Ayush Sharma, CC BY-SA 4.0)
And that is about it! The gentle and darkish modes now work as anticipated!
(Ayush Sharma, CC BY-SA 4.0)
(Ayush Sharma, CC BY-SA 4.0)
(Ayush Sharma, CC BY-SA 4.0)
(Ayush Sharma, CC BY-SA 4.0)
Wrap up
Bootstrap 5 is advanced, to say the least. There is quite a bit to consider when overriding it together with your customized styling. Providing gentle and darkish variants for each Bootstrap 5 element is troublesome, but it surely’s attainable if you do not have too many parts to cope with.
By guaranteeing that the markup stays in Sass/CSS, reusing kinds, and overriding some Bootstrap 5 defaults, it is attainable to attain gentle and darkish modes. It’s not a complete strategy, however it’s sensible and serviceable till Bootstrap 5 decides to offer this characteristic out of the field.
I hope this offers you extra sensible concepts on how you can add gentle and darkish themes to your individual web site. If you discover a higher means to make use of your individual CSS magic, do not forget to share it with the neighborhood.
Happy coding 🙂
This article initially appeared on the creator’s blog and has been republished with permission.
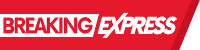