C is a well known programming language, common with skilled and new programmers alike. Source code written in C makes use of commonplace English phrases, so it is thought of human-readable. However, computer systems solely perceive binary code. To convert code into machine language, you utilize a instrument known as a compiler.
A quite common compiler is GCC (GNU C Compiler). The compilation course of includes a number of intermediate steps and adjoining instruments.
Install GCC
To verify whether or not GCC is already put in in your system, use the gcc
command:
$ gcc --version
If crucial, set up GCC utilizing your packaging supervisor. On Fedora-based techniques, use dnf
:
$ sudo dnf set up gcc libgcc
On Debian-based techniques, use apt
:
$ sudo apt set up build-essential
After set up, if you wish to verify the place GCC is put in, then use:
$ whereis gcc
Simple C program utilizing GCC
Here’s a easy C program to show how one can compile code utilizing GCC. Open your favourite textual content editor and paste on this code:
Save the file as hellogcc.c
after which compile it:
$ ls
hellogcc.c$ gcc hellogcc.c
$ ls -1
a.out
hellogcc.c
As you’ll be able to see, a.out
is the default executable generated because of compilation. To see the output of your newly-compiled utility, simply run it as you’ll any native binary:
Name the output file
The filename a.out
is not very descriptive, so if you wish to give a particular identify to your executable file, you should use the -o
possibility:
$ gcc -o hellogcc hellogcc.c$ ls
a.out hellogcc hellogcc.c$ ./hellogcc
Hello, GCC!
This possibility is helpful when growing a big utility that should compile a number of C supply recordsdata.
Intermediate steps in GCC compilation
There are literally 4 steps to compiling, though GCC performs them mechanically in easy use-cases.
- Pre-Processing: The GNU C Preprocessor (
cpp
) parses the headers (#embody statements), expands macros (#outline statements), and generates an intermediate file comparable tohellogcc.i
with expanded supply code. - Compilation: During this stage, the compiler converts pre-processed supply code into meeting code for a particular CPU structure. The ensuing meeting file is called with a
.s
extension, comparable tohellogcc.s
on this instance. - Assembly: The assembler (
as
) converts the meeting code into machine code in an object file, comparable tohellogcc.o
. - Linking: The linker (
ld
) hyperlinks the article code with the library code to provide an executable file, comparable tohellogcc
.
When operating GCC, use the -v
choice to see every step intimately.
$ gcc -v -o hellogcc hellogcc.c
(Jayashree Huttanagoudar, CC BY-SA 4.0)
Manually compile code
It may be helpful to expertise every step of compilation as a result of, below some circumstances, you do not want GCC to undergo all of the steps.
First, delete the recordsdata generated by GCC within the present folder, besides the supply file.
$ rm a.out hellogcc.o$ ls
hellogcc.c
Pre-processor
First, begin the pre-processor, redirecting its output to hellogcc.i
:
$ cpp hellogcc.c > hellogcc.i$ ls
hellogcc.c hellogcc.i
Take a take a look at the output file and see how the pre-processor has included the headers and expanded the macros.
Compiler
Now you’ll be able to compile the code into meeting. Use the -S
choice to set GCC simply to provide meeting code.
$ gcc -S hellogcc.i$ ls
hellogcc.c hellogcc.i hellogcc.s$ cat hellogcc.s
Take a take a look at the meeting code to see what’s been generated.
Assembly
Use the meeting code you’ve got simply generated to create an object file:
$ as -o hellogcc.o hellogcc.s$ ls
hellogcc.c hellogcc.i hellogcc.o hellogcc.s
Linking
To produce an executable file, you could hyperlink the article file to the libraries it is determined by. This is not fairly as simple because the earlier steps, nevertheless it’s instructional:
$ ld -o hellogcc hellogcc.o
ld: warning: can't discover entry image _start; defaulting to 0000000000401000
ld: hellogcc.o: in operate `fundamental`:
hellogcc.c:(.textual content+0xa): undefined reference to `places'
An error referencing an undefined places
happens after the linker is completed trying on the libc.so
library. You should discover appropriate linker choices to hyperlink the required libraries to resolve this. This is not any small feat, and it is depending on how your system is laid out.
When linking, you could hyperlink code to core runtime (CRT) objects, a set of subroutines that assist binary executables launch. The linker additionally must know the place to search out necessary system libraries, together with libc and libgcc, notably inside particular begin and finish directions. These directions may be delimited by the --start-group
and --end-group
choices or utilizing paths to crtbegin.o
and crtend.o
.
This instance makes use of paths as they seem on a RHEL 8 set up, so you might have to adapt the paths relying in your system.
$ ld -dynamic-linker
/lib64/ld-linux-x86-64.so.2
-o hi there
/usr/lib64/crt1.o /usr/lib64/crti.o
--start-group
-L/usr/lib/gcc/x86_64-redhat-linux/8
-L/usr/lib64 -L/lib64 hi there.o
-lgcc
--as-needed -lgcc_s
--no-as-needed -lc -lgcc
--end-group
/usr/lib64/crtn.o
The similar linker process on Slackware makes use of a special set of paths, however you’ll be able to see the similarity within the course of:
$ ld -static -o hi there
-L/usr/lib64/gcc/x86_64-slackware-linux/11.2.0/
/usr/lib64/crt1.o /usr/lib64/crti.o
hi there.o /usr/lib64/crtn.o
--start-group -lc -lgcc -lgcc_eh
--end-group
Now run the ensuing executable:
Some useful utilities
Below are just a few utilities that assist study the file sort, image desk, and the libraries linked with the executable.
Use the file
utility to find out the kind of file:
$ file hellogcc.c
hellogcc.c: C supply, ASCII textual content$ file hellogcc.o
hellogcc.o: ELF 64-bit LSB relocatable, x86-64, model 1 (SYSV), not stripped$ file hellogcc
hellogcc: ELF 64-bit LSB executable, x86-64, model 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=bb76b241d7d00871806e9fa5e814fee276d5bd1a, for GNU/Linux 3.2.0, not stripped
The use the nm
utility to checklist image tables for object recordsdata:
$ nm hellogcc.o
0000000000000000 T fundamental
U places
Use the ldd
utility to checklist dynamic hyperlink libraries:
$ ldd hellogcc
linux-vdso.so.1 (0x00007ffe3bdd7000)
libc.so.6 => /lib64/libc.so.6 (0x00007f223395e000)
/lib64/ld-linux-x86-64.so.2 (0x00007f2233b7e000)
Wrap up
In this text, you discovered the varied intermediate steps in GCC compilation and the utilities to look at the file sort, image desk, and libraries linked with an executable. The subsequent time you utilize GCC, you will perceive the steps it takes to provide a binary file for you, and when one thing goes improper, you know the way to step by means of the method to resolve issues.
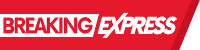