Java is a platform-independent language. Programs are transformed to bytecode after compilation. This bytecode will get transformed to machine code at runtime. An interpreter emulates the execution of bytecode directions for the summary machine on a selected bodily machine. Just-in-time (JIT) compilation occurs sooner or later throughout execution, and ahead-of-time (AOT) compilation occurs throughout construct time.
This article explains when an interpreter comes into play and when JIT and AOT will happen. I additionally talk about the trade-offs between JIT and AOT.
Source code, bytecode, machine code
Applications are usually written utilizing a programming language like C, C++, or Java. The set of directions written utilizing high-level programming languages known as supply code. Source code is human readable. To execute it on the goal machine, supply code must be transformed to machine code, which is machine readable. Source code is often transformed into machine code by a compiler.
In Java, nonetheless, the supply code is first transformed into an intermediate type referred to as bytecode. This bytecode is platform unbiased, which is why Java is effectively referred to as a platform-independent programming language. The major Java compiler javac
converts the Java supply code into bytecode. Then, the bytecode is interpreted by the interpreter.
Here is a small Hello.java
program:
Compile it utilizing javac
to generate a Hello.class
file containing the bytecode.
$ javac Hello.java
$ ls
Hello.class Hello.java
Now, use javap
to disassemble the content material of the Hello.class
file. The output of javap
relies on the choices used. If you do not select any choices, it prints fundamental info, together with which supply file this class file is compiled from, the package deal title, public and guarded fields, and strategies of the category.
$ javap Hello.class
Compiled from "Hello.java"
public class Hello {
public Hello();
public static void foremost(java.lang.String[]);
}
To see the bytecode content material within the .class
file, use the -c
possibility:
$ javap -c Hello.class
Compiled from "Hello.java"
public class Hello {
public Hello();
Code:
0: aload_0
1: invokespecial #1 // Method java/lang/Object."<init>":()V
4: returnpublic static void foremost(java.lang.String[]);
Code:
0: getstatic #2 // Field java/lang/System.out:Ljava/io/PrintStream;
3: ldc #3 // String Inside Hello World!
5: invokevirtual #4 // Method
java/io/PrintStream.println:(Ljava/lang/String;)V
8: return
}
To get extra detailed info, use the -v
possibility:
$ javap -v Hello.class
Interpreter, JIT, AOT
The interpreter is chargeable for emulating the execution of bytecode directions for the summary machine on a selected bodily machine. When compiling supply code utilizing javac
and executing utilizing the java
command, the interpreter operates throughout runtime and serves its objective.
$ javac Hello.java
$ java Hello
Inside Hello World!
The JIT compiler additionally operates at runtime. When the interpreter interprets a Java program, one other element, referred to as a runtime profiler, is silently monitoring this system’s execution to look at which portion of the code is getting interpreted and what number of instances. These statistics assist detect the hotspots of this system, that’s, these parts of code often being interpreted. Once they’re interpreted above a set threshold, they’re eligible to be transformed into machine code straight by the JIT compiler. The JIT compiler is often known as a profile-guided compiler. Conversion of bytecode to native code occurs on the fly, therefore the title just-in-time. JIT reduces overhead of the interpreter emulating the identical set of directions to machine code.
The AOT compiler compiles code throughout construct time. Generating often interpreted and JIT-compiled code at construct time improves the warm-up time of the Java Virtual Machine (JVM). This compiler was launched in Java 9 as an experimental characteristic. The jaotc
software makes use of the Graal compiler, which is itself written in Java, for AOT compilation.
Here’s a pattern use case for a Hello program:
When do decoding and compiling come into play: an instance
This instance illustrates when Java makes use of an interpreter and when JIT and AOT pitch in. Consider a easy Java program, Demo.java
:
//Demo.java
public class Demo {
public int sq.(int i) throws Exception {
return(i*i);
}public static void foremost(String[] args) throws Exception {
for (int i = 1; i <= 10; i++) {
System.out.println("call " + Integer.valueOf(i));
lengthy a = System.nanoTime();
Int r = new Demo().sq.(i);
System.out.println("Square(i) = " + r);
lengthy b = System.nanoTime();
System.out.println("elapsed= " + (b-a));
System.out.println("--------------------------------");
}
}
}
This easy program has a foremost
methodology that creates a Demo
object occasion, and calls the tactic sq.
, which shows the sq. root of the for
loop iteration worth. Now, compile and run the code:
The query now’s whether or not the output is a results of the interpreter, JIT, or AOT. In this case, it is wholly interpreted. How did I conclude that? Well, to get JIT to contribute to the compilation, the hotspots of the code should be interpreted above an outlined threshold. Then and solely then are these items of code queued for JIT compilation. To discover the brink for JDK 11:
$ java -XX:+PrintFlagsFinal -model | grep CompileThreshold
intx CompileThreshold = 10000 {pd product} {default}
[...]
openjdk model "11.0.13" 2021-10-19
OpenJDK Runtime Environment 18.9 (construct 11.0.13+8)
OpenJDK 64-Bit Server VM 18.9 (construct 11.0.13+8, combined mode, sharing)
The above output demonstrates {that a} specific piece of code ought to be interpreted 10,000 instances to be eligible for JIT compilation. Can this threshold be manually tuned, and is there some JVM flag that signifies whether or not a technique is JIT compiled? Yes, there are a number of choices to serve this objective.
One possibility for studying whether or not a technique is JIT compiled is -XX:+PrintCompilation
. Along with this selection, the flag -Xbatch
offers the output in a extra readable manner. If each interpretation and JIT are taking place in parallel, the -Xbatch
flag helps distinguish the output of each. Use these flags as follows:
$ java -Xbatch -XX:+PrintCompilation Demo
34 1 b 3 java.util.concurrent.ConcurrentHashMap::tabAt (22 bytes)
35 2 n 0 jdk.inner.misc.Unsafe::getObjectRisky (native)
35 3 b 3 java.lang.Object::<init> (1 bytes)
[...]
210 269 n 0 java.lang.replicate.Array::newArray (native) (static)
211 270 b 3 java.lang.String::substring (58 bytes)
[...]
--------------------------------
10 iteration
Square(i) = 100
Time taken= 50150
--------------------------------
The output of the above command is just too prolonged, so I’ve truncated the center portion. Note that together with the Demo program code, the JDKs inner class features are additionally getting compiled. This is why the output is so prolonged. Because my focus is Demo.java
code, I’ll use an possibility that may decrease the output by excluding the inner package deal features. The command –XX:CompileCommandFile
disables JIT for inner courses:
$ java -Xbatch -XX:+PrintCompilation -XX:CompileCommandFile=hotspot_compiler Demo
The file hotspot_compiler
referenced by -XX:CompileCommandFile
comprises this code to exclude particular packages:
$ cat hotspot_compiler
quiet
exclude java/* *
exclude jdk/* *
exclude solar/* *
In the primary line, quiet
instructs the JVM to not write something about excluded courses. To tune the JIT threshold, use -XX:CompileThreshold
with the worth set to five, which means that after decoding 5 instances, it is time for JIT:
$ java -Xbatch -XX:+PrintCompilation -XX:CompileCommandFile=hotspot_compiler
-XX:CompileThreshold=5 Demo
47 1 n 0 java.lang.invoke.MethodDeal with::hyperlinkToStatic(LLLLLL)L (native)
(static)
47 2 n 0 java.lang.invoke.MethodDeal with::invokeBasic(LLLLL)L (native)
47 3 n 0 java.lang.invoke.MethodDeal with::hyperlinkToParticular(LLLLLLL)L (native)
(static)
48 4 n 0 java.lang.invoke.MethodDeal with::hyperlinkToStatic(L)I (native) (static)
48 5 n 0 java.lang.invoke.MethodDeal with::invokeBasic()I (native)
48 6 n 0 java.lang.invoke.MethodDeal with::hyperlinkToParticular(LL)I (native)
(static)
[...]
1 iteration
69 40 n 0 java.lang.invoke.MethodDeal with::hyperlinkToStatic(ILIIL)I (native)
(static)
[...]
Square(i) = 1
78 48 n 0 java.lang.invoke.MethodDeal with::hyperlinkToStatic(ILIJL)I (native)
(static)
79 49 n 0 java.lang.invoke.MethodDeal with::invokeBasic(ILIJ)I (native)
[...]
86 54 n 0 java.lang.invoke.MethodDeal with::invokeBasic(J)L (native)
87 55 n 0 java.lang.invoke.MethodDeal with::hyperlinkToParticular(LJL)L (native)
(static)
Time taken= 8962738
--------------------------------
2 iteration
Square(i) = 4
Time taken= 26759
--------------------------------10 iteration
Square(i) = 100
Time taken= 26492
--------------------------------
The output remains to be not totally different from interpreted output! This is as a result of, as per Oracle’s documentation, the -XX:CompileThreshold
flag is efficient solely when TieredCompilation
is disabled:
$ java -Xbatch -XX:+PrintCompilation -XX:CompileCommandFile=hotspot_compiler
-XX:-TieredCompilation -XX:CompileThreshold=5 Demo
124 1 n java.lang.invoke.MethodDeal with::hyperlinkToStatic(LLLLLL)L (native) (static)
127 2 n java.lang.invoke.MethodDeal with::invokeBasic(LLLLL)L (native)
[...]
1 iteration
187 40 n java.lang.invoke.MethodDeal with::hyperlinkToStatic(ILIIL)I (native) (static)
[...]
(native) (static)
212 54 n java.lang.invoke.MethodDeal with::invokeBasic(J)L (native)
212 55 n java.lang.invoke.MethodDeal with::hyperlinkToParticular(LJL)L (native) (static)
Time taken= 12337415
[...]
--------------------------------
4 iteration
Square(i) = 16
Time taken= 37183
--------------------------------
5 iteration
214 56 b Demo::<init> (5 bytes)
215 57 b Demo::sq. (16 bytes)
Square(i) = 25
Time taken= 983002
--------------------------------
6 iteration
Square(i) = 36
Time taken= 81589
[...]
10 iteration
Square(i) = 100
Time taken= 52393
This part of code is now JIT compiled after the fifth interpretation:
--------------------------------
5 iteration
214 56 b Demo::<init> (5 bytes)
215 57 b Demo::sq. (16 bytes)
Square(i) = 25
Time taken= 983002
--------------------------------
Along with the sq.()
methodology, the constructor can be getting JIT compiled as a result of there’s a Demo occasion contained in the for
loop earlier than calling sq.()
. Hence, it should additionally attain the brink and be JIT compiled. This instance illustrates when JIT comes into play after interpretation.
To see the compiled model of the code, use the -XX:+PrintAssembly flag
, which works provided that there’s a disassembler within the library path. For OpenJDK, use the hsdis
disassembler. Download an acceptable disassembler library— on this case, hsdis-amd64.so
— and place it underneath Java_HOME/lib/server
. Make positive to make use of -XX:+UnlockDiagnosticVMOptions
earlier than -XX:+PrintAssembly
. Otherwise, JVM gives you a warning.
The whole command is as follows:
$ java -Xbatch -XX:+PrintCompilation -XX:CompileCommandFile=hotspot_compiler -XX:-TieredCompilation -XX:CompileThreshold=5 -XX:+UnlockDiagnosticVMOptions -XX:+PrintAssembly Demo
[...]
5 iteration
178 56 b Demo::<init> (5 bytes)
Compiled methodology (c2) 178 56 Demo::<init> (5 bytes)
whole in heap [0x00007fd4d08dad10,0x00007fd4d08dafe0] = 720
relocation [0x00007fd4d08dae88,0x00007fd4d08daea0] = 24
[...]
handler desk [0x00007fd4d08dafc8,0x00007fd4d08dafe0] = 24
[...]
dependencies [0x00007fd4d08db3c0,0x00007fd4d08db3c8] = 8
handler desk [0x00007fd4d08db3c8,0x00007fd4d08db3f8] = 48
----------------------------------------------------------------------
Demo.sq.(I)I [0x00007fd4d08db1c0, 0x00007fd4d08db2b8] 248 bytes
[Entry Point]
[Constants]
# {methodology} {0x00007fd4b841f4b0} 'sq.' '(I)I' in 'Demo'
# this: rsi:rsi = 'Demo'
# parm0: rdx = int
# [sp+0x20] (sp of caller)
[...]
[Stub Code]
0x00007fd4d08db280: movabs $0x0,%rbx ; {no_reloc}
0x00007fd4d08db28a: jmpq 0x00007fd4d08db28a ; {runtime_call}
0x00007fd4d08db28f: movabs $0x0,%rbx ; {static_stub}
0x00007fd4d08db299: jmpq 0x00007fd4d08db299 ; {runtime_call}
[Exception Handler]
0x00007fd4d08db29e: jmpq 0x00007fd4d08bb880 ; {runtime_call ExceptionBlob}
[Deopt Handler Code]
0x00007fd4d08db2a3: callq 0x00007fd4d08db2a8
0x00007fd4d08db2a8: subq $0x5,(%rsp)
0x00007fd4d08db2ad: jmpq 0x00007fd4d08a01a0 ; {runtime_call DeoptimizationBlob}
0x00007fd4d08db2b2: hlt
0x00007fd4d08db2b3: hlt
0x00007fd4d08db2b4: hlt
0x00007fd4d08db2b5: hlt
0x00007fd4d08db2b6: hlt
0x00007fd4d08db2b7: hlt
ImmutableOopMap{rbp=SlenderOop }laptop offsets: 96
ImmutableOopMap{}laptop offsets: 112
ImmutableOopMap{rbp=Oop }laptop offsets: 148 Square(i) = 25
Time taken= 2567698
--------------------------------
6 iteration
Square(i) = 36
Time taken= 76752
[...]
--------------------------------
10 iteration
Square(i) = 100
Time taken= 52888
The output is prolonged, so I’ve included solely the output associated to Demo.java
.
Now it is time for AOT compilation. This possibility was launched in JDK9. AOT is a static compiler to generate the .so
library. With AOT, the courses could be compiled to create an .so
library that may be straight executed as an alternative of decoding or JIT compiling. If JVM would not discover any AOT-compiled code, the standard interpretation and JIT compilation takes place.
The command used for AOT compilation is as follows:
$ jaotc --output=libDemo.so Demo.class
To see the symbols within the shared library, use the next:
$ nm libDemo.so
To use the generated .so
library, use -XX:AOTLibrary
together with -XX:+UnlockExperimentalVMOptions
as follows:
This output seems as whether it is an interpreted model itself. To make it possible for the AOT compiled code is utilized, use -XX:+PrintAOT
:
$ java -XX:+UnlockExperimentalVMOptions -XX:AOTLibrary=./libDemo.so -XX:+PrintAOT Demo
28 1 loaded ./libDemo.so aot library
80 1 aot[ 1] Demo.foremost([Ljava/lang/String;)V
80 2 aot[ 1] Demo.sq.(I)I
80 3 aot[ 1] Demo.<init>()V
1 iteration
Square(i) = 1
Time taken= 7252921
--------------------------------
2 iteration
Square(i) = 4
Time taken= 57443
[...]
10 iteration
Square(i) = 100
Time taken= 53586
Just to make it possible for JIT compilation hasn’t occurred, use the next:
$ java -XX:+UnlockExperimentalVMOptions -Xbatch -XX:+PrintCompilation -XX:CompileCommandFile=hotspot_compiler -XX:-TieredCompilation -XX:CompileThreshold=3 -XX:AOTLibrary=./libDemo.so -XX:+PrintAOT Demo
19 1 loaded ./libDemo.so aot library
77 1 aot[ 1] Demo.sq.(I)I
77 2 aot[ 1] Demo.foremost([Ljava/lang/String;)V
77 3 aot[ 1] Demo.<init>()V
77 2 aot[ 1] Demo.foremost([Ljava/lang/String;)V made not entrant
[...]
4 iteration
Square(i) = 16
Time taken= 43366
[...]
10 iteration
Square(i) = 100
Time taken= 59554
If any small change is made to the supply code subjected to AOT, it is vital to make sure that the corresponding .so
is created once more. Otherwise, the stale AOT-compiled .so
will not have any impact. For instance, make a small change to the sq. perform such that now it is calculating dice:
//Demo.java
public class Demo {public int sq.(int i) throws Exception {
return(i*i*i);
}public static void foremost(String[] args) throws Exception {
for (int i = 1; i <= 10; i++) {
System.out.println("" + Integer.valueOf(i)+" iteration");
lengthy begin = System.nanoTime();
int r= new Demo().sq.(i);
System.out.println("Square(i) = " + r);
lengthy finish = System.nanoTime();
System.out.println("Time taken= " + (finish-begin));
System.out.println("--------------------------------");
}
}
}
Now, compile Demo.java
once more:
$ java Demo.java
But, do not create libDemo.so
utilizing jaotc
. Instead, use this command:
$ java -XX:+UnlockExperimentalVMOptions -Xbatch -XX:+PrintCompilation -XX:CompileCommandFile=hotspot_compiler -XX:-TieredCompilation -XX:CompileThreshold=3 -XX:AOTLibrary=./libDemo.so -XX:+PrintAOT Demo
20 1 loaded ./libDemo.so aot library
74 1 n java.lang.invoke.MethodDeal with::hyperlinkToStatic(LLLLLL)L (native) (static)
2 iteration
sqrt(i) = 8
Time taken= 43838
--------------------------------
3 iteration
137 56 b Demo::<init> (5 bytes)
138 57 b Demo::sq. (6 bytes)
sqrt(i) = 27
Time taken= 534649
--------------------------------
4 iteration
sqrt(i) = 64
Time taken= 51916
[...]
10 iteration
sqrt(i) = 1000
Time taken= 47132
Though the previous model of libDemo.so
is loaded, JVM detected it as a stale one. Every time a .class
file is created, a fingerprint goes into the category file, and a category fingerprint is saved within the AOT library. Because the category fingerprint is totally different from the one within the AOT library, AOT-compiled native code will not be used. Instead, the tactic is now JIT compiled, as a result of the -XX:CompileThreshold
is ready to three.
AOT or JIT?
If you’re aiming to cut back the warm-up time of the JVM, use AOT, which reduces the burden throughout runtime. The catch is that AOT is not going to have sufficient information to resolve which piece of code must be precompiled to native code. By distinction, JIT pitches in throughout runtime and impacts the warm-up time. However, it should have sufficient profiling information to compile and decompile the code extra effectively.
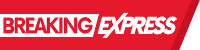