Having a darkish theme in your web site is a typical characteristic today. There are varied methods so as to add a darkish theme to your web site, and on this article, I exhibit a beginner-friendly method of programming a darkish theme for the online. Feel free to discover, make errors, and, extra importantly, be taught by manipulating the code in your individual method.
(Sachin Samal, CC BY-SA 4.0)
Icons
I like to offer a visible method for my customers to find the darkish mode characteristic. You can embody a straightforward set of icons by inserting the Font Awesome hyperlink within the <head> factor of your HTML.
Inside your <physique> tag, create a Font Awesome moon icon class, which you’ll swap to the Font Awesome solar icon class later utilizing JavaScript. This icon permits customers to change from the default mild theme to the darkish theme and again once more. In this case, you are altering from fa-moon whereas within the mild theme to fa-sun whereas at midnight theme. In different phrases, the icon is at all times the alternative of the present mode.
Next, create a CSS class in your stylesheet. You’ll append this utilizing the JavaScript add() technique to toggle between themes. The toggle() perform provides or removes a category identify from a component with JavaScript. This CSS code creates a changeTheme class, setting the background shade to darkish grey and the foreground shade (that is the textual content) to mild grey.
.changeTheme {
background: #1D1E22;
shade: #eee;
}
Toggle between themes
To toggle the looks of the theme button and to use the changeTheme class, use the onclick(), toggle(), incorporates(), add(), and take away() JavaScript strategies inside your <script> tag:
<script>
//will get the button by ID out of your HTML factor
const themeBtn = doc.getElementById("theme-btn");
//while you click on that button
themeBtn.onclick = () => {
//the default class "fa-moon" switches to "fa-sun" on toggle
themeBtn.classList.toggle("fa-sun");
//after the swap on toggle, in case your button incorporates "fa-sun" class
if (themeBtn.classList.incorporates("fa-sun")) {
//onclicking themeBtn, the changeTheme styling can be utilized to the physique of your HTML
doc.physique.classList.add("changeTheme");
} else {
// onclicking themeBtn, utilized changeTheme styling can be eliminated
doc.physique.classList.take away("changeTheme");
}
}
</script>
The full code:
<!doctype html>
<html lang="en">
<head>
<title>Toggle - Dark Theme</title>
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.0.7/css/all.css">
</head>
<style>
/* Toggle Dark Theme */
#theme-btn {
font-size: 1.5rem;
cursor: pointer;
shade: #596AFF;
}
#theme-btn:hover {
shade: #BB86FC;
}
.changeTheme {
background: #1D1E22;
shade: #eee;
}
</style>
<body>
<div id="theme-btn" class="far fa-moon"></div>
<script>
const themeBtn = doc.getElementById("theme-btn");
themeBtn.onclick = () => {
themeBtn.classList.toggle("fa-sun");
if (themeBtn.classList.incorporates("fa-sun")) {
doc.physique.classList.add("changeTheme");
} else {
doc.physique.classList.take away("changeTheme");
}
}
</script>
</body>
</html>
Complete themes
The code thus far could not totally swap the theme of a fancy web site. For occasion, your web site may need a header, a important, and a footer, every with a number of divs and different components. In that case, you could possibly create a regular darkish theme CSS class and append it to the specified internet components.
Get accustomed to your browser’s console
To examine your browser’s console, on the webpage the place you run this code, press Ctrl+Shift+I or right-click and choose the Inspect possibility.
When you choose Elements within the console and toggle your theme button, the browser provides you a sign of whether or not or not your JavaScript is working. In the console, you possibly can see that the CSS class you appended utilizing JavaScript is added and eliminated as you toggle.
(Sachin Samal, CC BY-SA 4.0)
Add a navigation and card part to see how including the CSS class identify on an HTML factor with JavaScript works.
Example code for a darkish theme
Here’s some instance code. You can alternately view it with a dwell preview here.
<!doctype html>
<html lang="en">
<head>
<title>Toggle - Dark Theme</title>
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.0.7/css/all.css">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
</head><style>
#header {
width: 100%;
box-shadow: 0px 4px 10px rgba(52, 72, 115, 0.35);
}
nav {
width: 100%;
show: flex;
flex-wrap: wrap;
justify-content: space-between;
align-items: heart;
}
.nav-menu {
max-width: 100%;
show: flex;
align-items: heart;
justify-content: space-between;
}
.nav-menu li {
margin: 0 0.5rem;
list-style: none;
text-decoration: none;
}
.nav-menu li a {
text-decoration: none;
shade: inherit;
}
h1 {
text-align: heart;
}/* Toggle Dark Theme */
#theme-btn {
font-size: 1.5rem;
cursor: pointer;
shade: #596AFF;
}#theme-btn:hover {
shade: #BB86FC;
}
.changeTheme {
background: #1D1E22;
shade: #eee;
}
/*-- Card Section --*/
.card-section .card {
border: none;
}
.card-body {
box-shadow: rgba(50, 50, 93, 0.25) 0px 2px 5px -1px,
rgba(0, 0, 0, 0.3) 0px 1px 3px -1px;
}
.card-body ul {
margin: 0;
padding: 0;
}
.card-body ul li {
text-decoration: none;
list-style-type: none;
text-align: heart;
font-size: 14px;
}
</style><body>
<header id="header">
<nav>
<div id="theme-btn" class="far fa-moon"></div>
<div class="navbar">
<ul class="nav-menu">
<li class="nav-item">
<a class="nav-link fas fa-home" href="# "> Home
</a>
</li>
</ul>
</div>
</nav>
</header>
<section>
<h1>Beginner Friendly Dark Theme</h1>
</section><section class="card-section mt-3">
<div class="container text-center">
<div class="row"><div class="col-md-4 col-sm-12">
<div class="card p-1 dark-theme">
<div class="card-body">
<h6>What is Lorem Ipsum?</h6>
<ul>
<li>Sed sit amet felis tellus.</li>
<li>Sed sit amet felis tellus.</li>
</ul>
</div>
</div>
</div><div class="col-md-4 col-sm-12">
<div class="card p-1 dark-theme">
<div class="card-body">
<h6>What is Lorem Ipsum?</h6>
<ul>
<li>Sed sit amet felis tellus.</li>
<li>Sed sit amet felis tellus.</li>
</ul>
</div>
</div>
</div><div class="col-md-4 col-sm-12">
<div class="card p-1 dark-theme">
<div class="card-body">
<h6>What is Lorem Ipsum?</h6>
<ul>
<li>Sed sit amet felis tellus.</li>
<li>Sed sit amet felis tellus.</li>
</ul>
</div>
</div>
</div></div>
</div>
</section>
<script>
const themeBtn = doc.getElementById("theme-btn");
const darkishTheme = doc.querySelectorAll(".dark-theme");
themeBtn.onclick = () => {
themeBtn.classList.toggle("fa-sun");
if (themeBtn.classList.incorporates("fa-sun")) {
doc.physique.classList.add("changeTheme");
for (const theme of darkishTheme) {
theme.fashion.backgroundColor = "#1D1E22";
theme.fashion.shade = "#eee";
}
} else {
doc.physique.classList.take away("changeTheme");
for (const theme of darkishTheme) {
theme.fashion.backgroundColor = "#eee";
theme.fashion.shade = "#1D1E22";
}
}
}
</script>
</body>
</html>
The for…of loop of JavaScript applies “.dark-theme” class styling properties to every card on the web page, no matter its place. It applies the theme to all internet components chosen with querySelectorAll() within the <script> tag. Without this, the font and background shade stay unchanged on toggle.
{
theme.fashion.backgroundColor = "#eee";
theme.fashion.shade = "#1D1E22";
}
If you set background-color or any shade property to the web page, your darkish theme wouldn’t work as anticipated. This is as a result of the preset CSS fashion overrides the one but to be appended. If you set the colour of the HTML font to black, it stays the identical at midnight theme, which you don’t need. For instance:
By including this code in your CSS or in a <fashion> tag, you set the font shade to black for all of the HTML on the web page. When you toggle the theme, the font stays black, which does not distinction with the darkish background. The identical goes for the background shade. This is widespread in the event you’ve used, for example, Bootstrap to create the card sections within the code above. When Bootstrap is used, the styling for every card comes from Bootstrap’s card.scss styling, which units background-color to white, which is extra particular than a basic CSS rule for the whole web page, and so it takes priority.
You should goal such instances by utilizing a JavaScript selector and set your required background-color or shade utilizing doc.fashion.backgroundColor or doc.fashion.shade properties.
Dark theme with a number of internet components
Here’s an example of a personal portfolio with a darkish theme characteristic that will aid you perceive find out how to allow darkish mode when you may have a number of internet components.
These steps are a beginner-friendly strategy to programming darkish themes. Whether you goal your HTML factor in JavaScript by utilizing getElementById() or getElementByClassName(), or with querySelector() or querySelectorAll(), is dependent upon the content material of your web site, and the way you wish to program as a developer. The identical is true with the number of JavaScript if…else statements and loops. Feel free to decide on your individual technique. As at all times, think about the constraints and adaptability of your programming strategy. Good luck!
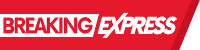