If you do not embody exams in your Drupal growth, likelihood is it is since you suppose it provides complexity and expense with out profit. Cypress is an open supply software with many advantages:
- Reliably exams something that runs in an online browser
- Works on any net platform (it is nice for testing initiatives utilizing front-end applied sciences like React)
- Highly extensible
- Increasingly well-liked
- Easy to study and implement
- Protects towards regression as your initiatives develop into extra advanced
- Can make your growth course of extra environment friendly
This article covers three matters that can assist you begin testing your Drupal venture utilizing Cypress:
Install Cypress
For the needs of this tutorial I’m assuming that you’ve got constructed an area dev atmosphere in your Drupal venture utilizing the `drupal/recommended-project` venture. Although particulars on creating such a venture are outdoors of the scope of this piece, I like to recommend Getting Started with Lando and Drupal 9.
Your venture has not less than this fundamental construction:
vendor/
net/
.editorconfig
.gitattributes
composer.json
composer.lock
The cypress.io website has complete installation instructions for numerous environments. For this text, I put in Cypress utilizing npm.
Initialize your venture utilizing the command npm init
. Answer the questions that Node.js asks you, after which you should have a bundle.json
file that appears one thing like this:
{
"name": "cypress",
"version": "1.0.0",
"description": "Installs Cypress in a test project.",
"main": "index.js",
"scripts": {
"test": "echo "Error: no check specified" && exit 1"
},
"author": "",
"license": "ISC"
}
Install Cypress in your venture:
$ npm set up cypress --save-dev
Run Cypress for the primary time:
$ npx cypress open
Because you have not added a config or any scaffolding recordsdata to Cypress, the Cypress app shows the welcome display that can assist you configure the venture. To configure your venture for E2E (end-to-end) testing, click on the Not Configured button for E2E Testing. Cypress provides some recordsdata to your venture:
cypress/
node_modules/
vendor/
net/
.editorconfig
.gitattributes
composer.json
composer.lock
cypress.config.js
package-lock.json
bundle.json
Click Continue and select your most popular browser for testing. Click Start E2E Testing in [your browser of choice]. I’m utilizing a Chromium-based browser for this text.
In a separate window, a browser opens to the Create your first spec web page:
(Jordan Graham, CC BY-SA 4.0)
Click on the Scaffold instance specs button to create a few new folders with instance specs that can assist you perceive how one can use Cypress. Read by way of these in your code editor, and you may probably discover the language (primarily based on JavaScript) intuitive and straightforward to comply with.
Click on any within the check browser. This reveals two panels. On the left, a textual content panel reveals every step within the lively spec. On the proper, a simulated browser window reveals the precise person expertise as Cypress steps by way of the spec.
Open the cypress.config.js
file in your venture root and alter it as follows:
const { defineConfig } = require("cypress");
module.exports = defineConfig({
element: {
fixturesFolder: "cypress/fixtures",
integrationFolder: "cypress/integration",
pluginsFile: "cypress/plugins/index.js",
screenshotsFolder: "cypress/screenshots",
supportFile: "cypress/support/e2e.js",
videosFolder: "cypress/videos",
viewportWidth: 1440,
viewportHeight: 900,
},
e2e: {
setupNodeOccasions(on, config) {
// implement node occasion listeners right here
},
baseUrl: "https://[your-local-dev-url]",
specPattern: "cypress/**/*.{js,jsx,ts,tsx}",
supportFile: "cypress/support/e2e.js",
fixturesFolder: "cypress/fixtures"
},
});
Change the baseUrl
to your venture’s URL in your native dev atmosphere.
These modifications inform Cypress the place to search out its sources and how one can discover the entire specs in your venture.
Write and run fundamental exams utilizing Cypress
Create a brand new listing referred to as integration
in your /cypress
listing. Within the integration
listing, create a file referred to as check.cy.js
:
cypress/
├─ e2e/
├─ fixtures/
├─ integration/
│ ├─ check.cy.js
├─ help/
node_modules/
vendor/
net/
.editorconfig
.gitattributes
composer.json
composer.lock
cypress.config.js
package-lock.json
bundle.json
Add the next contents to your check.cy.js
file:
describe('Loads the entrance web page', () => {
it('Loads the entrance web page', () => {
cy.go to("https://opensource.com/")
cy.get('h1.page-title')
.ought to('exist')
});
});
describe('Tests logging in utilizing an incorrect password', () => {
it('Fails authentication utilizing incorrect login credentials', () => {
cy.go to('/person/login')
cy.get('#edit-name')
.sort('Sir Lancelot of Camelot')
cy.get('#edit-pass')
.sort('tacos')
cy.get('enter#edit-submit')
.accommodates('Log in')
.click on()
cy.accommodates('Unrecognized username or password.')
});
});
When you click on on check.cy.js
within the Cypress software, watch every check description on the left as Cypress performs the steps in every describe()
part.
This spec demonstrates how one can inform Cypress to navigate your web site, entry HTML parts by ID, enter content material into enter parts, and submit the shape. This course of is how I found that I wanted so as to add the assertion that the <enter id="edit-submit">
factor accommodates the textual content Log in earlier than the enter was clickable. Apparently, the flex styling of the submit enter impeded Cypress’ means to “see” the enter, so it could not click on on it. Testing actually works!
Customize Cypress for Drupal
You can write your individual customized Cypress instructions, too. Remember the supportFile
entry within the cypress.config.js
file? It factors to a file that Cypress added, which in flip imports the ./instructions
recordsdata. Incidentally, Cypress is so intelligent that when importing logic or information fixtures, you need not specify the file extension, so that you import ./instructions
, not ./instructions.js
. Cypress seems for any of a dozen or so well-liked file extensions and understands how one can acknowledge and parse every of them.
Enter instructions into instructions.js
to outline them:
/**
* Logs out the person.
*/
Cypress.Commands.add('drupalLogout', () => {
cy.go to('/person/logout');
})
/**
* Basic person login command. Requires legitimate username and password.
*
* @param {string} username
* The username with which to log in.
* @param {string} password
* The password for the person's account.
*/
Cypress.Commands.add('loginAs', (username, password) => {
cy.drupalLogout();
cy.go to('/person/login');
cy.get('#edit-name')
.sort(username);
cy.get('#edit-pass').sort(password, {
log: false,
});
cy.get('#edit-submit').accommodates('Log in').click on();
});
This instance defines a customized Cypress command referred to as drupalLogout()
, which you should utilize in any subsequent logic, even different customized instructions. To log a person out, name cy.drupalLogout()
. This is the primary occasion within the customized command loginAs
to make sure that Cypress is logged out earlier than making an attempt to log in as a particular person.
Using atmosphere variables, you possibly can even create a Cypress command referred to as drush()
, which you should utilize to execute Drush instructions in your exams or customized instructions. Look at how easy this makes it to outline a customized Cypress command that logs a person in utilizing their UID:
/**
* Logs a person in by their uid through drush uli.
*/
Cypress.Commands.add('loginUserByUid', (uid) => {
cy.drush('user-login', [], { uid, uri: Cypress.env('baseUrl') })
.its('stdout')
.then(operate (url) {
cy.go to(url);
});
});
This instance makes use of the drush user-login
command (drush uli
for brief) and takes the authenticated person to the positioning’s base URL.
Consider the safety advantage of by no means studying or storing person passwords in your testing. Personally, I discover it superb {that a} front-end expertise like Cypress can execute Drush instructions, which I’ve at all times considered being very a lot on the again finish.
Testing, testing
There’s much more to Cypress, like fixtures (recordsdata that maintain check information) and numerous tips for navigating the generally advanced information buildings that produce a web site’s person interface. For a glance into what’s doable, watch the Cypress Testing for Drupal Websites webinar, notably the section on fixtures that begins at 18:33. That webinar goes into larger element about some fascinating use circumstances, together with an Ajax-enabled kind. Once you begin utilizing it, be at liberty to make use of or fork Aten’s public repository of Cypress Testing for Drupal.
Happy testing!
This article initially appeared on the Aten blog and is republished with permission.
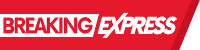