Transport Layer Security (TLS) is a cryptographic protocol primarily based on SSLv3 designed to encrypt and decrypt visitors between two websites. In different phrases, TLS ensures that you simply’re visiting the positioning you meant to go to and prevents anybody between you and the web site from seeing the info being handed backwards and forwards. This is achieved via the mutual alternate of digital certificates: a non-public one which exists on the internet server, and a public one usually distributed with internet browsers.
In manufacturing environments, all servers run securely, however server certificates might expire after some interval. It is then the server’s duty to validate, regenerate, and reuse newly generated certificates with none downtime. In this text, I show how TLS certificates are up to date dynamically utilizing an HTTPS server in Go.
These are the stipulations for following this tutorial:
- A fundamental understanding of client-server working fashions
- A fundamental data of Golang
Configuring an HTTP server
Before I focus on tips on how to replace certs dynamically on an HTTPS server, I’ll present a easy HTTP server instance. In this case, I want solely the http.ListenAndServe
operate to start out an HTTP server and http.HandleFunc
to register a response handler for a selected endpoint.
To begin, configure the HTTP server:
package deal most importantimport(
"net/http"
"fmt"
"log"
)func most important() {
mux := http.NewServeMux()
mux.HandleFunc("https://opensource.com/", func( res http.ResponseWriter, req *http.Request ) {
fmt.Fprint( res, "Running HTTP Server!!" )
})
srv := &http.Server{
Addr: fmt.Sprintf(":%d", 8080),
Handler: mux,
}// run server on port "8080"
log.Fatal(srv.ListenAndServe())
}
In the instance above, after I run the command go run server.go
, it is going to begin an HTTP server on port 8080. By visiting the http://localhost:8080 URL in your browser, it is possible for you to to see a Hello World! message on the display.
The srv.ListenAndServe()
name makes use of Go’s normal HTTP server configuration. However, you may customise a server utilizing a Server
construction kind.
To begin an HTTPS server, name the srv.ListenAndServeTLS(certFile, keyFile)
technique with some configuration, identical to the srv.ListenAndServe()
technique. TheListenAndServe
and ListenAndServeTLS
strategies can be found on each the HTTP package deal and the Server
construction.
The ListenAndServeTLS
technique is rather like the ListenAndServe
technique, besides it is going to begin an HTTPS server.
func ListenAndServeTLS(certFile string, keyFile string) error
As you may see from the tactic signature above, the one distinction between this technique and the ListenAndServe
technique is the extra certFile
and keyFile
arguments. These are the paths to the SSL certificates file and non-public key file, respectively.
Generating a non-public key and an SSL certificates
Follow these steps to generate a root key and certificates:
1. Create the basis key:
openssl genrsa -des3 -out rootCA.key 4096
2. Create and self-sign the basis certificates:
openssl req -x509 -new -nodes -key rootCA.key -sha256 -days 1024 -out rootCA.crt
Next, comply with these steps to generate a certificates (for every server):
1. Create the certificates key:
openssl genrsa -out localhost.key 2048
2. Create the certificate-signing request (CSR). The CSR is the place you specify the main points for the certificates you wish to generate. The proprietor of the basis key will course of this request to generate the certificates. When creating the CSR, it is very important specify the Common Name
offering the IP deal with or area identify for the service, in any other case the certificates can’t be verified.
openssl req -new -key localhost.key -out localhost.csr
3. Generate the certificates utilizing the TSL CSR and key together with the CA Root key:
openssl x509 -req -in localhost.csr -CA rootCA.crt -CAkey rootCA.key -CAcreateserial -out localhost.crt -days 500 -sha256
Finally, comply with the identical steps for producing certificates for every server to generate certificates for shoppers.
Configuring an HTTPS server
Now that you’ve each non-public key and certificates recordsdata, you may modify your earlier Go program and use the ListenAndServeTLS
technique as a substitute.
package deal most importantimport(
"net/http"
"fmt"
"log"
)func most important() {
mux := http.NewServeMux()
mux.HandleFunc("https://opensource.com/", func( res http.ResponseWriter, req *http.Request ) {
fmt.Fprint( res, "Running HTTPS Server!!" )
})
srv := &http.Server{
Addr: fmt.Sprintf(":%d", 8443),
Handler: mux,
}// run server on port "8443"
log.Fatal(srv.ListenAndServeTLS("localhost.crt", "localhost.key"))
}
When you run the above program, it is going to begin an HTTPS server utilizing localhost.crt
because the certFile
and locahost.key
because the keyFile
from the present listing containing this system file. By visiting the https://localhost:8443 URL through browser or command-line interface (CLI), you may see the outcome beneath:
$ curl https://localhost:8443/ --cacert rootCA.crt --key consumer.key --cert consumer.crtRunning HTTPS Server!!
That’s it! This is what most individuals need to do to launch an HTTPS server. Go manages the default conduct and performance of the TLS communication.
Configuring an HTTPS server for robotically replace certificates
While working the HTTPS server above, you handed certFile
and keyFile
to the ListenAndServeTLS
operate. However, if there’s any change in certFile
and keyFile
due to certificates expiry, the server must be restarted to take these results. To overcome that, you should utilize the TLSConfig
of the web/http
package deal.
The TLSConfig
construction supplied by the crypto/tls
package deal configures the TLS parameters of the Server
, together with server certificates, amongst others. All fields of the TLSConfig
construction are non-obligatory. Hence, assigning an empty struct worth to the TLSConfig
discipline isn’t any totally different than assigning a nil
worth. However, the GetCertificate
discipline may be very helpful.
kind Config struct {
GetCertificate func(*ShopperHelloInfo) (*Certificate, error)
}
The GetCertificate
discipline of the TLSConfig
construction returns a certificates primarily based on the given ShopperHelloInfo
.
I must implement GetCertificate
closure operate which makes use of tls.LoadX509KeyPair(certFile string, keyFile string)
or tls.X509KeyPair(certFile []byte, keyFile []byte)
operate to get the certificates.
Now I’ll create a Server
struct that makes use of TLSConfig
discipline worth. Here is a pattern format of the code:
package deal most importantimport (
"crypto/tls"
"fmt"
"log"
"net/http"
)func most important() {
mux := http.NewServeMux()
mux.HandleFunc("https://opensource.com/", func( res http.ResponseWriter, req *http.Request ) {
fmt.Fprint( res, "Running HTTPS Server!!n" )
})
srv := &http.Server{
Addr: fmt.Sprintf(":%d", 8443),
Handler: mux,
TLSConfig: &tls.Config{
GetCertificate: func(*tls.ShopperHelloInfo) (*tls.Certificate, error) {
// Always get newest localhost.crt and localhost.key
// ex: retaining certificates file someplace in world location the place created certificates up to date and this closure operate can refer that
cert, err := tls.LoadX509KeyPair("localhost.crt", "localhost.key")
if err != nil {
return nil, err
}
return &cert, nil
},
},
}// run server on port "8443"
log.Fatal(srv.ListenAndServeTLS("", ""))
}
In the above program, I carried out the GetCertificate
closure operate, which returns a cert object of kind Certificate
through the use of the LoadX509KeyPair
operate with the certificates and personal recordsdata created earlier. It additionally returns an error, so deal with it rigorously.
Since I’m preconfiguring server occasion srv
with a TLS configuration, I don’t want to supply certFile
and keyFile
argument values to the srv.ListenAndServeTLS
operate name. When I run this server, it is going to work identical to earlier than. But this time, I’ve abstracted all of the server configuration from the invoker.
$ curl https://localhost:8443/ --cacert rootCA.crt --key consumer.key --cert consumer.crtRunning HTTPS Server!!
Final ideas
A couple of extra suggestions by the use of closing: To learn the cert and key file contained in the GetCertificate
operate, the HTTPS server ought to run as a goroutine. There can also be a StackOverflow thread that gives different methods of configuration
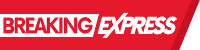