In the Lua programming language, an array known as a desk. A desk is utilized in Lua to retailer knowledge. If you are storing a variety of knowledge in a structured approach, it is helpful to know your choices for retrieving that knowledge while you want it.
Creating a desk in Lua
To create a desk in Lua, you instantiate the desk with an arbitrary identify:
mytable = {}
There are other ways you possibly can construction your knowledge in a desk. You may fill it with values, basically creating an inventory (known as a checklist in some languages):
mytable = { 'zombie', 'apocalypse' }
Or you may create an related array (known as a map or dictionary in some languages). You can add arbitrary keys to the desk utilizing dot notation. You may also add a price to that key the identical approach you add a price to a variable:
myarray = {}
myarray.baz = 'completely happy'
myarray.qux = 'halloween'
You can add verification with the assert()
operate:
You now have two tables: a list-style mytable
and an associative array-style myarray
.
Iterating over a desk with pairs
Lua’s pairs()
operate extracts key and worth pairs from a desk.
print('pairs of myarray:')for ok,v in pairs(myarray) do
print(ok,v)
finish
Here’s the output:
pairs of myarray:
baz completely happy
qux halloween
If there aren’t any keys in a desk, Lua makes use of an index. For occasion, the mytable
desk comprises the values zombie
and apocalypse
. It comprises no keys, however Lua can improvise:
print('pairs of mytable:')for ok,v in pairs(mytable) do
print(ok,v)
finish
Here’s the output:
1 zombie
2 apocalypse
Iterating over a desk with ipairs
To account for the truth that tables with out keys are frequent, Lua additionally offers the ipairs
operate. This operate extracts the index and the worth:
print('ipairs of mytable:')for i,v in ipairs(mytable) do
print(i,v)
finish
The output is, on this case, the identical because the output of pairs
:
1 zombie
2 apocalypse
However, watch what occurs while you add a key and worth pair to mytable
:
mytable.shock = 'this worth has a key'print('ipairs of mytable:')
for i,v in ipairs(mytable) do
print(i,v)
finish
Lua ignores the important thing and worth as a result of ipairs
retrieves solely listed entries:
1 zombie
2 apocalypse
The key and worth pair, nevertheless, have been saved within the desk:
print('pairs of mytable:')for ok,v in ipairs(mytable) do
print(ok,v)
finish
The output:
1 zombie
2 apocalypse
shock this worth has a key
Retrieving arbitrary values
You do not need to iterate over a desk to get knowledge out of it. You can name arbitrary knowledge by both index or key:
print('name by index:')
print(mytable[2])
print(mytable[1])
print(myarray[2])
print(myarray[1])print('name by key:')
print(myarray['qux'])
print(myarray['baz'])
print(mytable['surprise'])
The output:
name by index:
apocalypse
zombie
nil
nilname by key:
halloween
completely happy
this worth has a key
Data constructions
Sometimes utilizing a Lua desk makes much more sense than making an attempt to maintain observe of dozens of particular person variables. Once you perceive how you can construction and retrieve knowledge in a language, you are empowered to generate complicated knowledge in an organized and protected approach.
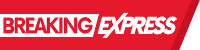