Lua is a scripting language used for procedural programming, practical programming, and even object-oriented programming. It makes use of a C-like syntax, however is dynamically typed, options automated reminiscence administration and rubbish assortment, and runs by deciphering bytecode with a register-based digital machine. This makes it an ideal language for rookies, but in addition a strong instrument for skilled programmers.
Lua has been considerably eclipsed from the general public view by languages like Python and JavaScript, however Lua has a number of benefits that make it in style in some main software program tasks. Lua is well embedded inside different languages, which means that you could embrace Lua recordsdata within the code base of one thing written in (as an example) Java and it runs as if it have been native Java code. It appears like magic, however in fact there are tasks like luaj working to make it doable, and it is solely doable as a result of Lua is designed for it. It’s partly due to this flexibility that you just’re more likely to discover Lua because the scripting language for video video games, graphic functions, and extra.
As with something, it takes time to good, however Lua is straightforward (and enjoyable) to study. It’s a constant language, a pleasant language with helpful error messages, and there is a lot of nice assist on-line. Ready to get began?
Installing Lua
On Linux, you possibly can set up Lua utilizing your distribution’s bundle supervisor. For occasion, on Fedora, CentOS, Mageia, OpenMandriva, and related distributions:
$ sudo dnf set up lua
On Debian and Debian-based methods:
$ sudo apt set up lua
For Mac, you should utilize MacPorts or Homebrew.
$ sudo port set up lua
For Windows, set up Lua utilizing Chocolatey.
To take a look at Lua in an interactive interpreter, kind lua
in a terminal.
Functions
As with many programming languages, Lua syntax typically includes a built-in perform or key phrase, adopted by an argument. For occasion, the print
perform shows any argument you present to it.
$ lua
Lua 5.4.2 Copyright (C) 1994-2020 Lua.org, PUC-Rio
> print('good day')
good day
Lua’s string
library can manipulate phrases (referred to as “strings” in programming.) For occasion, to rely the letters in a string, you utilize the len
perform of the string
library:
> string.len('good day')
5
Variables
A variable lets you create a particular place in your laptop’s reminiscence for short-term knowledge. You can create variables in Lua by inventing a reputation to your variable, after which placing some knowledge into it.
> foo = "hello world"
> print(foo)
good day world
> bar = 1+2
> print(bar)
3
Tables
Second solely to the recognition of variables in programming is the recognition of arrays. The phrase “array” actually means an association, and that is all a programming array is. It’s a selected association of information, and since there’s an association, an array has the benefit of being structured. An array is commonly used to carry out basically the identical function as a variable, besides that an array can implement an order to its knowledge. In Lua, an array known as a desk
.
Creating a desk is like making a variable, besides that you just set its preliminary content material to 2 braces ({}
):
> mytable = {}
When you add knowledge to a desk, it is also quite a bit like making a variable, besides that your variable identify at all times begins with the identify of the desk, and is separated with a dot:
> mytable.foo = "hello world"
> mytable.bar = 1+2
> print(mytable.foo)
good day world
> print(mytable.bar)
3
Scripting with Lua
Running Lua within the terminal is nice for getting instantaneous suggestions, nevertheless it’s extra helpful to run Lua as a script. A Lua script is only a textual content file containing Lua code, which the Lua command can interpret and execute.
The everlasting query, when simply beginning to study a programming language, is the way you’re alleged to know what to put in writing. This article has offered a superb begin, however to date you solely know two or three Lua features. The key, in fact, is in documentation. The Lua language is not that complicated, and it’s extremely cheap to confer with the Lua documentation site for a listing of key phrases and features.
Here’s a apply drawback.
Suppose you need to write a Lua script that counts phrases in a sentence. As with many programming challenges, there are a lot of methods to go about this, however say the primary related perform you discover within the Lua docs is string.gmatch
, which may seek for a selected character in a string. Words are normally separated by an empty area, so that you determine that counting areas + 1 must render a fairly correct rely of the phrases they’re separating.
Here’s the code for that perform:
perform wc(phrases,delimiter)
rely=1
for w in string.gmatch(phrases, delimiter) do
rely = rely + 1
finish
return rely
finish
These are the elements of that pattern code:
-
perform
: A key phrase declaring the beginning of a perform. A customized perform works principally the identical method as built-in features (likeprint
andstring.len
.) -
phrases
anddelimiter
: Arguments required for the perform to run. In the assertionprint('good day')
, the phrasegood day
is an argument. -
counter
: A variable set to 1. -
for
: A loop utilizing thestring.gmatch
perform because it iterates over thephrases
you’ve got enter into the perform, and searches for thedelimiter
you’ve got enter. -
rely = rely +1
: For everydelimiter
discovered, the worth ofrely
is re-set to its present worth plus 1. -
finish
: A key phrase ending thefor
loop. -
return rely
: This perform outputs (or returns) the contents of therely
variable. -
finish
: A key phrase ending the perform.
Now that you’ve got created a perform all your individual, you should utilize it. That’s an necessary factor to recollect a few perform. It would not run by itself. It waits so that you can name it in your code.
Type this pattern code right into a textual content file and put it aside as phrases.lua
:
perform wc(phrases,delimiter)
rely=1
for w in string.gmatch(phrases, delimiter) do
rely = rely + 1
finish
return rely
finish
outcome = wc('zombie apocalypse', ' ')
print(outcome)
outcome = wc('ice cream sandwich', ' ')
print(outcome)
outcome = wc('can you discover the bug? ', ' ')
print(outcome)
You’ve simply created a Lua script. You can run it with Lua. Can you discover the issue with this technique of counting phrases?
$ lua ./phrases.lua
2
3
6
You would possibly discover that the rely is wrong for the ultimate phrase as a result of there is a trailing area within the argument. Lua accurately detected the area and tallied it into rely
, however the phrase rely is wrong as a result of that exact area occurs to not delimit a phrase. I go away it to you to unravel that drawback, or to search out different methods wherein this technique is not perfect. There’s loads of rumination in programming. Sometimes it is purely educational, and different occasions it is a query of whether or not an utility works in any respect.
Learning Lua
Lua is a enjoyable and sturdy language, with progressive enhancements made with every launch, and an ever-growing developer base. You can use Lua as a utilitarian language for private scripts, or to advance your profession, or simply as an experiment in studying a brand new language. Give it a attempt, and see what Lua brings to the desk.
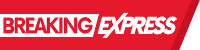