Knowing the best way to learn and write recordsdata may be helpful for numerous functions. In Rust, this job is finished utilizing the file system module (std::fs) in the usual library. In this text, I’ll offer you an summary on the best way to use this module.
To reveal this job, I ready instance code which can be obtainable on GitHub.
Preparation
When utilizing Rust, a operate that fails returns the Result sort. The file system module specifically returns the specialised sort std::io::Result<T, Error>. With this information, you possibly can return the identical sort from the principal()
operate:
fn principal() -> std::io::Result<()> {
/* ...code comes right here... */
Writing Rust recordsdata
Performing file I/O-operations in Rust is comparatively straightforward. Writing to a file may be simplified to 1 line:
use std::fs;
fs::write("favorite_websites.txt", b"opensource.com")?;
Ok(())
Using the error propagation operator (?)
, the error data will get handed on to the calling operate the place the error can subsequently be dealt with. As principal()
is the one different operate within the name stack, the error data will get handed on to the console output in case the write operation failed.
The syntax of the fs::write operate is sort of ahead. The first argument is the file path, which should be the kind std::path::Path. The second argument is the content material, which is definitely a slice of bytes ([u8]
). Rust converts the arguments handed into the right sort. Luckily, these sorts are principally the one sorts handled within the following examples.
A extra concise entry of the write operation may be achieved utilizing the file descriptor sort std::fs::File:
let mut file = fs::File::create("favorite_websites.txt")?;
file.write_all(b"opensource.comn")?;
Ok(())
As the file sort implements the Write trait, it’s doable to make use of the related strategies to write down to the file. However, the create
methodology can overwrite an already present file.
To get much more management of the file descriptor, the kind std::fs::OpenOptions should be used. This gives opening modes just like those in different languages:
let mut file = fs::OpenOptions::new()
.append(true)
.open("favorite_websites.txt")?;
file.write_all(b"sourceforge.netn")?;
Reading Rust recordsdata
What applies to writing additionally applies to studying. Reading will also be performed with a easy one-line of code:
let web sites = fs::read_to_string("favorite_websites.txt")?;
The above line reads the content material of the file and returns a string. In addition to studying a string, there’s additionally the std::fs::read operate which reads the info right into a vector of bytes if the file accommodates binary knowledge.
The subsequent instance reveals the best way to learn the content material of the file into reminiscence and subsequently print it line by line to a console:
let file = fs::File::open("favorite_websites.txt")?;
let traces = io::BufReader::new(file).traces();for line in traces {
if let Ok(_line) = line {
println!(">>> {}", _line);
}
}
Summary
If you might be already accustomed to different programming languages, you’ll have seen that there’s no close-
operate (or one thing related) that releases the file deal with. In Rust, the file deal with is launched as quickly because the associated variable goes out of scope. To outline the closing habits, a scope ({ })
across the file illustration may be utilized. I like to recommend that you simply get accustomed to Read and Write trait as you could find this trait carried out in lots of different sorts.
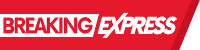