Python and Rust are very completely different languages, however they really go collectively fairly properly. But earlier than discussing methods to mix Python with Rust, I need to introduce Rust itself. You’ve possible heard of the language however could not have heard particulars about the way it works.
What is Rust?
Rust is a low-level language. This implies that the issues the programmers cope with are near the way in which computer systems “really” work.
For instance, integer varieties are outlined by bit dimension and correspond to CPU-supported varieties. While it’s tempting to say that this implies a+b
in Rust corresponds to at least one machine instruction, it doesn’t imply fairly that!
Rust’s compiler’s chain is non-trivial. It is helpful as a primary approximation to deal with statements like that as “kind of” true.
Rust is designed for zero-cost abstraction, that means lots of the abstractions accessible on the language stage are compiled away at runtime.
For instance, objects are allotted on the stack except explicitly requested for. The result’s that creating a neighborhood object in Rust has no runtime price (although initialization would possibly).
Finally, Rust is a memory-safe language. There are different memory-safe languages and different zero-cost abstraction languages. Usually, these are completely different languages.
Memory security doesn’t imply it’s not possible to have reminiscence violations in Rust. It does imply that there are solely two ways in which reminiscence violations can occur:
- A bug within the compiler.
- Code that is explicitly declared unsafe.
Rust customary library code has fairly a little bit of code that’s marked unsafe, although lower than what many assume. This doesn’t make the assertion vacuous although. With the (uncommon) exception of needing to jot down unsafe code your self, reminiscence violations consequence from the underlying infrastructure.
Why does Rust exist?
Why did individuals create Rust? What downside was not addressed by current languages?
Rust was designed as a language to attain a mix of high-performance code that’s reminiscence protected. This concern is more and more vital in a networked world.
The quintessential use case for Rust is low-level parsing of protocols. The knowledge to be parsed typically comes from untrusted sources and will should be parsed in a performant approach.
If this appears like what an online browser does, it’s no coincidence. Rust originated from the Mozilla Foundation as a approach to enhance the Firefox browser.
In the trendy world, browsers are now not the one issues on which there’s stress to be protected and quick. Even the widespread microservice structure, mixed with defense-in-depth ideas, should be capable to unpack untrusted knowledge shortly.
Counting characters
To perceive a “wrapping in Rust” instance, there must be an issue to resolve. Not simply any downside will do. The challenge must be:
- Easy sufficient to resolve
- Be helped by the power to jot down high-performance loops
- Somewhat real looking
The toy downside on this case is whether or not a personality seems greater than X instances in a string. This is one thing that is not simply amenable to performant common expressions. Even devoted Numpy code may be slower than needed as a result of typically there isn’t any have to scan your entire string.
You can think about some mixture of Python libraries and tips that make this attainable. However, the plain algorithm is fairly quick if carried out in a low-level language and makes issues extra readable.
A twist is added to make the issue barely extra attention-grabbing and exhibit some enjoyable components of Rust. The algorithm helps resetting the rely on a newline (does the character seem greater than X instances in a line?) or on an area (does the character seem greater than X instances in a phrase?).
This is the one nod given to “realism.” Any extra realism will make the instance not helpful pedagogically.
Enum assist
Rust helps enumeration (enums
). You can do many attention-grabbing issues with enums
.
For now, a three-way enum
with out some other twirls is used. The enum
encodes what character resets the rely.
#[derive(Copy)]
enum Reset {
NewlinesReset,
AreasReset,
NoReset,
}
Struct assist
The subsequent Rust part is a little more substantial: a struct
. A Rust struct
is considerably near a Python dataclass
. Again, you are able to do extra refined issues with a struct
.
#[pyclass]
struct Counter {
what: char,
min_number: u64,
reset: Reset,
}
Implementation blocks
You add a way to a struct
in a separate block in Rust: the impl
block. The particulars are outdoors the scope of this text.
In this instance, the tactic calls an exterior operate. This is usually performed to interrupt up the code. A extra refined use would instruct the Rust compiler to inline the operate to permit readability with none runtime price.
#[pymethods]
impl Counter {
#[new]
fn new(what: char, min_number: u64, reset: Reset) -> Self {
Counter{what: what, min_number: min_number, reset: reset}
}
fn has_count(
&self,
knowledge: &str,
) -> bool {
has_count(self, knowledge.chars())
}
}
Function
By default, Rust variables are fixed. Because the present rely has to alter, it’s declared as a mutable variable.
fn has_count(cntr: &Counter, chars: std::str::Chars) -> bool {
let mut current_count : u64 = 0;
for c in chars {
if got_count(cntr, c, &mut current_count) {
return true;
}
}
false
}
The loop goes over the characters and calls the operate got_count
. Again, that is performed to interrupt the code into slides. It does present methods to ship a mutable borrow reference to a operate.
Even although current_count
is mutable, each the sending and receiving websites explicitly mark the reference as mutable. This makes it clear which capabilities would possibly modify a worth.
Counting
The got_count
resets the counter, increments it, after which checks it. Rust’s colon-separated sequence of expressions evaluates to the results of the final expression, on this case, whether or not the brink was met.
fn got_count(cntr: &Counter, c: char, current_count: &mut u64) -> bool {
maybe_reset(cntr, c, current_count);
maybe_incr(cntr, c, current_count);
*current_count >= cntr.min_number
}
Reset code
The reset
code exhibits one other helpful factor in Rust: matching. A whole description of the matching skills in Rust can be a semester-level class, not two minutes in an unrelated speak, however this instance matches on a tuple matching certainly one of two choices.
fn maybe_reset(cntr: &Counter, c: char, current_count: &mut u64) -> () {
match (c, cntr.reset) {
('n', Reset::NewlinesReset) | (' ', Reset::AreasReset)=> {
*current_count = 0;
}
_ => {}
};
}
Increment assist
The increment compares the character to the specified one and, if matched, increments the rely.
fn maybe_incr(cntr: &Counter, c: char, current_count: &mut u64) -> (){
if c == cntr.what {
*current_count += 1;
};
}
Note that I optimized the code on this article for slides. It just isn’t essentially a best-practice instance of Rust code or methods to design a great API.
Wrap Rust code for Python
To wrap Rust code for Python, you should utilize PyO3. The PyO3 Rust “crate” (or library) permits inline hints for wrapping Rust code into Python, making it simpler to change each collectively.
Include PyO3 crate primitives
First, you will need to embody the PyO3 crate primitives.
use pyo3::prelude::*;
Wrap enum
The enum
must be wrapped. The derive
clauses are needed for wrapping the enum
for PyO3, as a result of they permit the category to be copied and cloned, making them simpler to make use of from Python.
#[pyclass]
#[derive(Clone)]
#[derive(Copy)]
enum Reset {
/* ... */
}
Wrap struct
The struct
is equally wrapped. These name “macros” in Rust, which generate the wanted interface bits.
#[pyclass]
struct Counter {
/* ... */
}
Wrap impl
Wrapping the impl
is extra attention-grabbing. Another macro is added referred to as new
. This methodology is marked as #[new]
, letting PyO3 know methods to expose a constructor for the built-in object.
#[pymethods]
impl Counter {
#[new]
fn new(what: char, min_number: u64,
reset: Reset) -> Self {
Counter{what: what,
min_number: min_number, reset: reset}
}
/* ... */
}
Define module
Finally, outline a operate that initializes the module. This operate has a selected signature, have to be named the identical because the module, and adorned with #[pymodule]
.
#[pymodule]
fn counter(_py: Python, m: &PyModule
) -> PyResult<()> {
m.add_class::<Counter>()?;
m.add_class::<Reset>()?;
Ok(())
}
The ?
exhibits that this operate can fail (for instance, if the category was not appropriately configured). The PyResult
is translated right into a Python exception at import time.
Maturin develop
For fast checking, maturin develop
builds and installs the library into the present digital setting. This helps iterate shortly.
$ maturin develop
Maturin construct
The maturin construct
command builds a manylinux
wheel, which may be uploaded to PyPI. The wheel is restricted to the CPU structure.
Python library
Using the library from Python is the great half. Nothing signifies a distinction between this and writing the code in Python. One helpful facet of that is that for those who optimize an current library in Python that already has unit assessments, you should utilize the Python unit assessments for the Rust library.
Import
Whether you used maturin develop
or pip set up
to put in it, importing the library is finished with import
.
import counter
Construct
The constructor was outlined precisely so the article could possibly be constructed from Python. This just isn’t all the time the case. Sometimes objects are solely returned from extra refined capabilities.
cntr = counter.Counter(
'c',
3,
counter.Reset.NewlinesReset,
)
Call
The closing pay-off is right here finally. Check whether or not this string has a minimum of three “c” characters:
>>> cntr.has_count("hello-c-c-c-goodbye")
True
Adding a newline causes the remaining to occur, and there aren’t three “c” characters with out an intervening newline:
>>> cntr.has_count("hello-c-c-nc-goodbye")
False
Using Rust and Python is simple
My objective is to persuade you that combining Rust and Python is simple. I wrote little code to “glue” them. Rust and Python have complementary strengths and weaknesses.
Rust is nice for high-performance, protected code. Rust has a steep studying curve and may be awkward for shortly prototyping an answer.
Python is simple to get began with and helps extremely tight iteration loops. Python does have a “speed cap.” Beyond a sure stage it’s tougher to get higher efficiency from Python.
Combining them is ideal. Prototype in Python and transfer efficiency bottlenecks to Rust.
With maturin
, your improvement and deployment pipelines are simpler to make. Develop, construct, and benefit from the combo!
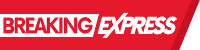