Some time loop performs a set of duties for so long as some predefined situation is true. This is taken into account a management construction that directs the movement of a program. It’s a means so that you can inform your code what to do by defining a situation that it could possibly check, and take motion primarily based on what it finds. The two sorts of whereas loops in Java are whereas and do whereas.
Java whereas loop
Some time loop is supposed to iterate over information till some situation is happy. To create some time loop, you present a situation that may be examined, adopted by the code you need to run. Java has a number of built-in check features, the only of that are mathematical operators (<
, >
, ==
, and so forth):
package deal com.opensource.instance;
public class Example {
public static void primary(String[] args) {
int depend = 0;
whereas (depend < 5) {
System.out.printf("%d ", depend);
depend++;
}
}
}
In this easy instance, the situation is that the variable depend
is lower than 5. Because depend
is instantiated at 0, after which incremented by 1 within the code inside the whereas loop, this system iterates a complete of 5 instances:
$ java ./whereas.java
0 1 2 3 4
Before it could possibly iterate a sixth time, the situation is not true, so the loop ends.
The conditional assertion for some time loop is significant. Getting it flawed may imply that your loop by no means executes. For occasion, suppose you had set depend == 5
because the situation:
whereas (depend == 5) {
System.out.printf("%d ", depend);
depend++;
When you run the code, it builds and runs efficiently, however nothing occurs:
$ java ./whereas.java
$
The loop has been skipped as a result of depend
was set to 0, and it is nonetheless 0 for the time being the whereas loop is first encountered. The loop by no means has a purpose to start out and depend
isn’t incremented.
The reverse of that is when a situation begins as true and might by no means be false, this leads to an infinite loop.
Java do whereas loop
Similar to the whereas loop, a do whereas loop checks for the conditional on the finish, not the start, of every iteration. With this, the code in your loop runs not less than as soon as as a result of there is no gateway to entry, solely a gateway to exit:
package deal com.opensource.instance;
public class Example {
public static void primary(String[] args) {
int depend = 9;
do {
System.out.printf("%d ", depend);
depend++;
} whereas(depend == 5);
}
}
In this pattern code, depend
is ready to 9. The situation for the loop to repeat is that depend
is the same as 5. But 9 is not equal to five. That test is not carried out till the top of the primary iteration, although:
$ java ./do.java
9
Java infinite loops
An infinite loop, as its identify suggests, by no means ends. Sometimes they’re created by mistake, however an infinite loop does have a sound use case. Sometimes you desire a course of to proceed indefinitely (that is functionally infinite as a result of you may’t assure once you want it to cease), and so that you may set your situation to one thing unattainable to satisfy.
Suppose you’ve got written an software that counts the variety of zombies remaining in your neighborhood throughout a zombie apocalypse. To simulate uncertainty over what number of loops are required to get to 0 zombies, my demo code retrieves a timestamp from the working system and units the worth of the counter (c
) to some quantity derived from that timestamp. Because this can be a easy instance and you do not actually need to get trapped in an infinite loop, this code counts all the way down to zero and makes use of the break
operate to power the loop to finish:
package deal com.opensource.instance;
public class Example {
public static void primary(String[] args) {
lengthy myTime = System.currentTimeMillis();
int c;
if ( myTimepercent2 == 0 ) {
c = 128;
} else {
c = 1024;
}
whereas(true) {
System.out.printf("%d Zombiesn", c);
// break for comfort
if ( c <= 0 ) { break; }
c--;
}
}
}
You might should run it just a few instances to set off a distinct complete variety of zombies, however typically your program iterates 128 instances and different instances 1,024 instances:
$ java ./zcount.java
1024 Zombies
1023 Zombies
[...]
0 Zombies
Can you inform why the loops finish at 0 and never at -1?
Java loops
Loops offer you management over the movement of your program’s execution. Iteration is widespread in programming, and whether or not you employ some time loop, a do whereas loop, or an infinite loop, understanding how loops work is significant.
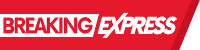