If you employ Python, you could have encountered the IndexError
error in response to some code you’ve got written. The IndexError
message in Python is a runtime error. To perceive what it’s and tips on how to repair it, you will need to first perceive what an index is. A Python record (or array or dictionary) has an index. The index of an merchandise is its place inside an inventory. To entry an merchandise in an inventory, you employ its index. For occasion, think about this Python record of fruits:
fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
This record’s vary is 5, as a result of an index in Python begins at 0.
- apple: 0
- banana: 1
- orange: 2
- pear: 3
- grapes: 4
- watermelon: 5
Suppose you should print the fruit identify pear
from this record. You can use a easy print
assertion, together with the record identify and the index of the merchandise you wish to print:
>>> fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
>>> print(fruits[3])
pear
What causes an IndexError in Python?
What in case you use an index quantity exterior the vary of the record? For instance, attempt to print the index quantity 6 (which does not exist):
>>> fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
>>> print(fruits[6])
Traceback (most up-to-date name final):
File "", line 2, in
IndexError: record index out of vary
As anticipated, you get IndexError: record index out of vary
in response.
How to repair IndexError in Python
The solely answer to repair the IndexError: record index out of vary
error is to make sure that the merchandise you entry from an inventory is inside the vary of the record. You can accomplish this by utilizing the vary()
an len()
features.
The vary()
operate outputs sequential numbers, beginning with 0 by default, and stopping on the quantity earlier than the required worth:
>>> n = vary(6)
>>> for i in n:
print(i)
0
1
2
3
4
5
5
The len()
operate, within the context of an inventory, returns the variety of objects within the record:
>>> fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
>>> print(len(fruits))
6
List index out of vary
By utilizing vary()
and len()
collectively, you’ll be able to forestall index errors. The len()
operate returns the size of the record (6, on this instance.) Using that size with vary()
turns into vary(6)
, which returns objects at index 0, 1, 2, 3, 4, and 5.
fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
for i in vary(len(fruits)):
print(fruits[i])
apple
banana
orange
pear
grapes
watermelon
Fix IndexError in Python loops
If you are not cautious, index errors can occur in Python loops. Consider this loop:
>>> fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
>>> n = 0
>>> whereas n <= len(fruits)
print(fruits[n])
n+=1
apple
banana
orange
pear
grapes
watermelon
Traceback (most up-to-date name final):
File "", line 4, in
IndexError: record index out of vary
The logic appears affordable. You’ve outlined n
as a counter variable, and you’ve got set the loop to happen till it equals the size of the record. The size of the record is 6, however its vary is 5 (as a result of Python begins its index
at 0). The situation of the loop is n <= 6
, and so thewhereas
loop stops when the worth of n
is the same as 6:
- When n is 0 => apple
- When n is 1 => banana
- When n is 2 => orange
- When n is 3 => pear
- When n is 4 => grapes
- When n is 5 => watermelon
- When n is 6 => IndexError: record index out of vary
When n
is the same as 6, Python produces an IndexError: record index out of vary
error.
Solution
To keep away from this error inside Python loops, use solely the < (“less than”) operator, stopping the whereas
loop on the final index of the record. This is one quantity wanting the record’s size:
>>> fruits = ["apple", "banana", "orange", "pear", "grapes", "watermelon"]
>>> n = 0
>>> whereas n < len(fruits)
print(fruits[n])
n+=1
apple
banana
orange
pear
grapes
watermelon
There’s one other solution to repair, this too, however I go away that to you to find.
No extra Python index errors
The final reason for IndexError
is an try and entry an merchandise that does not exist inside a knowledge construction. Using the vary()
and len()
features is one answer, and naturally understand that Python begins counting at 0, not 1.
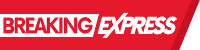