In programming, you typically want your code to iterate over a set of information to course of every merchandise individually. You use management constructions to direct the move of a program, and the way in which you inform your code what to do is by defining a situation that it may well take a look at. For occasion, you would possibly write code to resize a picture till all pictures have been resized as soon as. But how have you learnt what number of pictures there are? One consumer might need 5 pictures, and one other might need 100. How do you write code that may adapt to distinctive use circumstances? In many languages, Java included, one choice is the for loop. Another choice for Java is the Stream methodology. This article covers each.
For loop
A for loop takes a recognized amount of things and ensures that every merchandise is processed. An “item” is usually a quantity, or it may be a desk containing a number of entries, or any Java information kind. This primarily describes a counter:
For occasion, suppose you’ve three gadgets, and also you need Java to course of every one. Start your counter (name it c
) at 0 as a result of Java begins the index of arrays at 0. Set the cease worth to c < 3
or c ⇐ 2
. Finally, increment the counter by 1. Here’s some pattern code:
package deal com.opensource.instance;
public class Main {
public static void fundamental(String[] args) {
String[] myArray = {"zombie", "apocalypse", "alert" };
for (int i = 0; i < 3; i++) {
System.out.printf("%s ", myArray[i]);
}
}
}
Run the code to make sure all three gadgets are getting processed:
$ java ./for.java
zombie apocalypse alert
The circumstances are versatile. You can decrement as an alternative of increment, and you may increment (or decrement) by any quantity.
The for loop is a typical assemble in most languages. However, most programming languages are actively developed, and definitely, Java is all the time enhancing. There’s a brand new approach to iterate over information, and it is referred to as Java Stream.
Java Stream
The Java Stream interface is a characteristic of Java offering practical entry to a group of structured information. Instead of making a counter to “walk” by way of your information, you employ a Stream methodology to question the information:
package deal com.opensource.instance;
import java.util.Arrays;
import java.util.stream.Stream;
public class Example {
public static void fundamental(String[] args) {
// create an array
String[] myArray = new String[]{"plant", "apocalypse", "alert"};
// put array into Stream
Stream<String> myStream = Arrays.stream(myArray);
myStream.forEach(e -> System.out.println(e));
}
}
In this pattern code, you import the java.util.stream.Stream
library for entry to the Stream assemble, and java.util.Arrays
to maneuver an array into the Stream.
In the code, you create an Array as standard. Then you create a stream referred to as myStream
and put the information out of your array into it. Those two steps are broadly typical of Stream utilization: You have information, so you place the information right into a Stream so you possibly can analyze it.
What you do with the Stream is determined by what you need to obtain. Stream strategies are nicely documented in Java docs, however to imitate the fundamental instance of the for loop it is sensible to make use of the forEach
or forEachOrdered
methodology, which iterates over every component within the Stream. In this instance, you employ a lambda expression to create a parameter, which is handed to a print assertion:
$ java ./stream.java
plant
apocalypse
alert
When to make use of Stream
Streams do not actually exist to interchange for loops. In reality, Streams are supposed as ephemeral constructs that do not have to be saved in reminiscence. They’re supposed to collect information for evaluation after which disappear.
For occasion, watch what occurs in the event you attempt to use a Stream twice:
package deal com.opensource.instance;
import java.util.Arrays;
import java.util.stream.Stream;
public class Example {
public static void fundamental(String[] args) {
// create an array
String[] myArray = new String[]{"plant", "apocalypse", "alert"};
// put array into Stream
Stream<String> myStream = Arrays.stream(myArray);
lengthy myCount = myStream.depend();
System.out.println(myCount);
}
}
This will not work, and that is intentional:
$ java ./stream.java
Exception in thread "main"
stream has already been operated upon or closed
Part of the design philosophy of Java Stream is that you just carry information into your code. You then apply filters up entrance moderately than writing a bunch of if-then-else statements in a determined try to seek out the information you truly need, course of the information, after which eliminate the Stream. It’s much like a Linux pipe, wherein you ship the output of an software to your terminal solely to filter it by way of grep so you possibly can ignore all the information you do not want.
Here’s a easy instance of a filter to get rid of any entry in an information set that does not begin with an a
:
package deal com.opensource.instance;
import java.util.Arrays;
import java.util.stream.Stream;
public class Example {
public static void fundamental(String[] args) {
String[] myArray = new String[]{"plant", "apocalypse", "alert"};
Stream<String> myStream = Arrays.stream(myArray).filter(s -> s.startsWith("a"));
myStream.forEach(e -> System.out.println(e));
}
}
Here’s the output:
$ java ./stream.java
apocalypse
alert
For information you propose to make use of as a useful resource all through your code, use a for loop.
For information you need to ingest, parse, and overlook, use a Stream.
Iteration
Iteration is frequent in programming, and whether or not you employ a for loop or a Stream, understanding the construction and the choices you’ve means you can also make clever selections about how one can course of information in Java.
For superior utilization of Streams, learn Chris Hermansen’s article Don’t like loops? Try Java Streams.
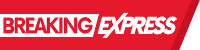