ChatOps is a collaboration mannequin that connects folks, processes, instruments, and automation right into a clear workflow. Mattermost is an open supply, self-hosted messaging platform that allows organizations to speak securely, successfully, and effectively. It’s an awesome open source alternative to Slack, Discord, and different proprietary messaging platforms. This article outlines the steps to create a ChatOps bot on Mattermost, together with the required code examples and explanations.
Prerequisites
Before beginning, guarantee that you’ve entry to a Mattermost server, have Python installed, and have put in the Mattermost Python driver using pip.
Create a bot account on Mattermost
To create a bot account, entry the Mattermost System Console, and add a bot account with acceptable entry permissions. Retrieve the bot’s username and password to be used within the Python script.
Set up the Mattermost Python driver
Install the Mattermost Python driver utilizing pip
, and import it within the Python script. Create a brand new driver occasion and log in to the Mattermost server.
Create the ChatOps bot in Python
Create a brand new Python script, outline the required libraries to be imported, and implement the bot’s performance utilizing the Mattermost driver’s API. Write code to deal with messages, instructions, and different occasions, and use the Mattermost driver’s API strategies to ship messages and notifications to channels and customers. Finally, debug and check the ChatOps bot.
Example of ChatOps bot code
Here is an instance Python code for a easy ChatOps bot that responds to a person’s message:
from mattermostdriver import Driver
bot_username="bot_username"
bot_password = 'bot_password'
server_url="https://your.mattermost.server.url"
def essential():
driver = Driver({'url': server_url, 'login_id': bot_username, 'password': bot_password, 'scheme': 'https'})
driver.login()
workforce = driver.groups.get_team_by_name('team_name')
channel = driver.channels.get_channel_by_name(workforce['id'], 'channel_name')
@driver.on('message')
def handle_message(put up, **kwargs):
if put up['message'] == 'howdy':
driver.posts.create_post({
'channel_id': put up['channel_id'],
'message': 'Hi there!'
})
driver.init_websocket()
if __name__ == '__main__':
essential()
Add options
Once you’ve got created a primary ChatOps bot on Mattermost, you may add extra options to increase its performance. Here are the steps:
-
Determine the characteristic you need to add: Before writing the code, you need to decide the characteristic so as to add to your ChatOps bot. This may be something from sending notifications to integrating with third-party instruments.
-
Write the code: Once you might have decided the characteristic you need to add, you can begin writing the code. The code will rely on the characteristic you add, however you should use the Mattermost Python driver to work together with the Mattermost API and implement the characteristic.
-
Test the code: After writing the code, it is necessary to check it to make sure that it really works as anticipated. Before deploying it to your manufacturing server, you may check the code on a improvement server or in a check channel.
-
Deploy the code: Once you might have examined it and it really works as anticipated, you may deploy it to your manufacturing server. Follow your group’s deployment course of and make sure the new code would not break any present performance.
-
Document the brand new characteristic: It’s necessary to doc the brand new characteristic you might have added to your ChatOps bot. This will make it simpler for different workforce members to make use of the bot and perceive its capabilities.
One instance of a ChatOps Bot characteristic could possibly be integrating with a third-party instrument and offering standing updates on sure duties.
from mattermostdriver import Driver
import requests
bot_username="bot_username"
bot_password = 'bot_password'
server_url="https://your.mattermost.server.url"
def essential():
driver = Driver({'url': server_url, 'login_id': bot_username, 'password': bot_password, 'scheme': 'https'})
driver.login()
workforce = driver.groups.get_team_by_name('team_name')
channel = driver.channels.get_channel_by_name(workforce['id'], 'channel_name')
@driver.on('message')
def handle_message(put up, **kwargs):
if put up['message'] == 'standing':
# Make a request to the third-party instrument API to get the standing
response = requests.get('https://api.thirdpartytool.com/status')
if response.status_code == 200:
standing = response.json()['status']
driver.posts.create_post({
'channel_id': put up['channel_id'],
'message': f'The standing is {standing}'
})
else:
driver.posts.create_post({
'channel_id': put up['channel_id'],
'message': 'Failed to get standing'
})
driver.init_websocket()
if __name__ == '__main__':
essential()
In this instance, the ChatOps bot listens for the command “status” and makes a request to a third-party instrument API to get the present standing. It then posts the standing replace within the Mattermost channel the place the command was issued. This permits workforce members to rapidly get updates on the standing of the duty with out having to depart the chat platform.
Open supply ChatOps
In abstract, making a ChatOps bot on Mattermost is an easy course of that may deliver quite a few advantages to your group’s communication and workflow. This article has offered a step-by-step breakdown and code examples that can assist you get began on creating your bot and even customise it by including new options. Now that the fundamentals, you may additional discover ChatOps and Mattermost to optimize your workforce’s collaboration and productiveness.
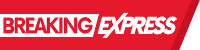