Asynchronous programming: Incredibly helpful however troublesome to be taught. You cannot keep away from async programming to create a quick and reactive utility. Applications with a excessive quantity of file or community I/O or with a GUI that ought to at all times be reactive profit tremendously from async programming. Tasks will be executed within the background whereas the consumer nonetheless makes inputs. Async programming is feasible in lots of languages, every with totally different types and syntax. Rust is not any exception. In Rust, this characteristic is named async-await.
While async-await has been an integral a part of Rust since model 1.39.0, most functions rely upon neighborhood crates. In Rust, besides for a bigger binary, async-await comes with zero prices. This article provides you an perception into asynchronous programming in Rust.
Under the hood
To get a fundamental understanding of async-await in Rust, you actually begin within the center.
The heart of async-await is the future trait, which declares the strategy ballot (I cowl this in additional element under). If a price will be computed asynchronously, the associated kind ought to implement the future trait. The ballot technique is named repeatedly till the ultimate worth is out there.
At this level, you might repeatedly name the ballot technique out of your synchronous utility manually with a view to get the ultimate worth. However, since I’m speaking about asynchronous programming, you’ll be able to hand over this activity to a different element: the runtime. So earlier than you may make use of the async syntax, a runtime should be current. I exploit the runtime from the tokio neighborhood crate within the following examples.
A helpful approach of constructing the tokio runtime accessible is to make use of the #[tokio::main]
macro in your primary operate:
#[tokio::main]
async fn primary(){
println!("Start!");
sleep(Duration::from_secs(1)).await;
println!("End after 1 second");
}
When the runtime is out there, now you can await futures. Awaiting implies that additional executions cease right here so long as the future must be accomplished. The await technique causes the runtime to invoke the ballot technique, which can drive the future to completion.
In the above instance, the tokios sleep operate returns a future that finishes when the required period has handed. By awaiting this future, the associated ballot technique is repeatedly known as till the future completes. Furthermore, the primary() operate additionally returns a future due to the async
key phrase earlier than the fn.
So for those who see a operate marked with async
:
async fn foo() -> usize { /**/ }
Then it’s simply syntactic sugar for:
fn foo() -> impl Future<Output = usize> { async { /**/ } }
Pinning and boxing
To take away a number of the shrouds and clouds of async-await in Rust, you will need to perceive pinning and boxing.
If you’re coping with async-await, you’ll comparatively rapidly step over the phrases boxing and pinning. Since I discover that the accessible explanations on the topic are quite obscure, I’ve set myself the objective of explaining the difficulty extra simply.
Sometimes it’s essential to have objects which might be assured to not be moved in reminiscence. This comes into impact when you have got a self-referential kind:
struct MustBePinned {
a: int16,
b: &int16
}
If member b is a reference (pointer) to member a of the identical occasion, then reference b turns into invalid when the occasion is moved as a result of the situation of member a has modified however b nonetheless factors to the earlier location. You can discover a extra complete instance of a self-referential kind within the Rust Async book. All that you must know now could be that an occasion of MustBePinned shouldn’t be moved in reminiscence. Types like MustBePinned don’t implement the Unpin trait, which might enable them to maneuver inside reminiscence safely. In different phrases, MustBePinned is !Unpin.
Back to the longer term: By default, a future can be !Unpin; thus, it shouldn’t be moved in reminiscence. So how do you deal with these sorts? You pin and field them.
The Pin<T> kind wraps pointer sorts, guaranteeing that the values behind the pointer will not be moved. The Pin<T> kind ensures this by not offering a mutable reference of the wrapped kind. The kind might be pinned for the lifetime of the article. If you by chance pin a kind that implements Unpin (which is secure to maneuver), it will not have any impact.
In follow: If you wish to return a future (!Unpin) from a operate, you will need to field it. Using Box<T> causes the sort to be allotted on the heap as a substitute of the stack and thus ensures that it may outlive the present operate with out being moved. In explicit, if you wish to hand over a future, you’ll be able to solely hand over a pointer to it because the future should be of kind Pin<Box<dyn Future>>.
Using async-wait, you’ll actually bump into this boxing and pinning syntax. To wrap this matter up, you simply have to recollect this:
- Rust doesn’t know whether or not a kind will be safely moved.
- Types that should not be moved should be wrapped inside Pin<T>.
- Most sorts are Unpinned sorts. They implement the trait Unpin and will be freely moved inside reminiscence.
- If a kind is wrapped inside Pin<T> and the wrapped kind is !Unpin, it isn’t doable to get a mutable reference out of it.
- Futures created by the async key phrase are !Unpin and thus should be pinned.
Future trait
In the future trait, every little thing comes collectively:
pub trait Future {
kind Output;fn ballot(self: Pin<&mut Self>, cx: &mut Context<'_>) -> Poll<Self::Output>;
}
Here is a straightforward instance of find out how to implement the future trait:
struct MyCounterFuture {
cnt : u32,
cnt_final : u32
}impl MyCounterFuture {
pub fn new(final_value : u32) -> Self {
Self {
cnt : 0,
cnt_final : final_value
}
}
}
impl Future for MyCounterFuture {
kind Output = u32;fn ballot(mut self: Pin<&mut Self>, cx: &mut Context<'_>) -> Poll<u32>{
self.cnt += 1;
if self.cnt >= self.cnt_final {
println!("Counting finished");
return Poll::Ready(self.cnt_final);
}cx.waker().wake_by_ref();
Poll::Pending
}
}#[tokio::main]
async fn primary(){
let my_counter = MyCounterFuture::new(42);let final_value = my_counter.await;
println!("Final value: {}", final_value);
}
Here is a straightforward instance of how the future trait is applied manually: The future is initialized with a price to which it shall rely, saved in cnt_final. Each time the ballot technique is invoked, the inner worth cnt will get incremented by one. If cnt is lower than cnt_final, the longer term alerts the waker of the runtime that the future is able to be polled once more. The return worth of Poll::Pending
alerts that the future has not accomplished but. After cnt is >= cnt_final, the ballot operate returns with Poll::Ready
, signaling that the future has accomplished and offering the ultimate worth.
This is only a easy instance, and naturally, there are different issues to care for. If you take into account creating your individual futures, I extremely recommend studying the chapter Async in depth within the documentation of the tokio crate.
Wrap up
Before I wrap issues up, right here is a few extra info that I take into account helpful:
- Create a brand new pinned and boxed kind utilizing Box::pin.
- The futures crate gives the sort BoxFuture which helps you to outline a future as return kind of a operate.
- The async_trait permits you to outline an async operate in traits (which is at present not allowed).
- The pin-utils crate gives macros to pin values.
- The tokios try_join! macro (a)waits on a number of futures which return a Result<T, E>.
Once the primary hurdles have been overcome, async programming in Rust is simple. You do not even should implement the future trait in your individual sorts for those who can outsource code that may be executed in parallel in an async operate. In Rust, single-threaded and multi-threaded runtimes can be found, so you’ll be able to profit from async programming even in embedded environments.
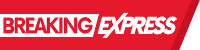