Java is (disputably) the undisputed heavyweight in open supply, cross-platform programming. While there are lots of great cross-platform frameworks, few are as unified and direct as Java.
Of course, Java can be a reasonably complicated language with subtleties and conventions all its personal. One of the most typical questions on Java relates to constructors: What are they and what are they used for?
Put succinctly: a constructor is an motion carried out upon the creation of a brand new object in Java. When your Java software creates an occasion of a category you could have written, it checks for a constructor. If a constructor exists, Java runs the code within the constructor whereas creating the occasion. That’s plenty of technical phrases crammed into a number of sentences, but it surely turns into clearer while you see it in motion, so be sure you have Java installed and prepare for a demo.
Life with out constructors
If you are writing Java code, you are already utilizing constructors, regardless that it’s possible you’ll not realize it. All lessons in Java have a constructor as a result of even when you have not created one, Java does it for you when the code is compiled. For the sake of demonstration, although, ignore the hidden constructor that Java gives (as a result of a default constructor provides no further options), and check out life with out an specific constructor.
Suppose you are writing a easy Java dice-roller software since you need to produce a pseudo-random quantity for a recreation.
First, you would possibly create your cube class to characterize a bodily die. Knowing that you just play plenty of Dungeons and Dragons, you resolve to create a 20-sided die. In this pattern code, the variable cube is the integer 20, representing the utmost attainable die roll (a 20-sided die can not roll greater than 20). The variable roll is a placeholder for what is going to ultimately be a random quantity, and rand serves because the random seed.
import java.util.Random;public class DiceRoller
As lengthy as you could have a Java improvement surroundings put in (equivalent to OpenJDK, you may run your software from a terminal:
$ java cube.java
You rolled a 12
In this instance, there isn’t a specific constructor. It’s a wonderfully legitimate and authorized Java software, but it surely’s just a little restricted. For occasion, in the event you set your recreation of Dungeons and Dragons apart for the night to play some Yahtzee, you would want 6-sided cube. In this straightforward instance, it would not be that a lot bother to alter the code, however that is not a practical possibility in complicated code. One method you can remedy this drawback is with a constructor.
Constructors in motion
The DiceRoller class on this instance challenge represents a digital cube manufacturing unit: When it is referred to as, it creates a digital die that’s then “rolled.” However, by writing a customized constructor, you may make your Dice Roller software ask what sort of die you’d wish to emulate.
Most of the code is identical, except for a constructor accepting some variety of sides. This quantity would not exist but, however it will likely be created later.
import java.util.Random;public class DiceRoller
Launch the applying and supply the variety of sides you need your die to have:
$ java cube.java 20
You rolled a 10
$ java cube.java 6
You rolled a 2
$ java cube.java 100
You rolled a 44
The constructor has accepted your enter, so when the category occasion is created, it’s created with the sides variable set to no matter quantity the person dictates.
Constructors are highly effective elements of programming. Practice utilizing them to unlock the complete potential of Java.
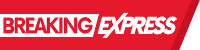